public int insert(int value); /* Creates a node with the parameter as its value * and inserts the node into the tree in the appropriate position. * This method should call upon the recursive insert_r() helper method * you write. * * we will make the assumption for this assignment (and implement it so) * that the values in a BST are unique... no duplicates * * If we insert a value and it succeeded... adds it to the tree * return the element that was added * * If we try to add an element and it is a duplicate, return the element * but make no changes to the tree... no new nodes added * * @param int value to be inserted in tree * @return integer that was insrted after insertion is done. * * Example 1: Suppose we have the tree below: * * (27) * / \ * (4) (29) * / \ * (1) (8) * After calling insert(5), we'd obtain the following tree: * * (27) * / \ * (4) (29) * / \ * (1) (8) * / * (5) * * Example 2: Suppose we have the tree below: * * (87) * / \ * (42) (128) * \ \ * (46) (145) * / * (44) * * After calling insert(147), we'd obtain the following tree: * * (87) * / \ * (42) (128) * \ \ * (46) (145) * / \ * (44) (147) * **/
public int insert(int value);
/* Creates a node with the parameter as its value
* and inserts the node into the tree in the appropriate position.
* This method should call upon the recursive insert_r() helper method
* you write.
*
* we will make the assumption for this assignment (and implement it so)
* that the values in a BST are unique... no duplicates
*
* If we insert a value and it succeeded... adds it to the tree
* return the element that was added
*
* If we try to add an element and it is a duplicate, return the element
* but make no changes to the tree... no new nodes added
*
* @param int value to be inserted in tree
* @return integer that was insrted after insertion is done.
*
* Example 1: Suppose we have the tree below:
*
* (27)
* / \
* (4) (29)
* / \
* (1) (8)
* After calling insert(5), we'd obtain the following tree:
*
* (27)
* / \
* (4) (29)
* / \
* (1) (8)
* /
* (5)
*
* Example 2: Suppose we have the tree below:
*
* (87)
* / \
* (42) (128)
* \ \
* (46) (145)
* /
* (44)
*
* After calling insert(147), we'd obtain the following tree:
*
* (87)
* / \
* (42) (128)
* \ \
* (46) (145)
* / \
* (44) (147)
*
**/
DESCRIPTIONS AND CODE IMPLEMENTATION IN PICTURES
FUNCTION SHOULD INSERT A NODE WITH A VALUE INTO A BST INTO ITS DESIGNATED SPOT
DO THIS RECURSIVELY
PLS AND THANK YOU!!!!!!!!!!!!!



Trending now
This is a popular solution!
Step by step
Solved in 2 steps

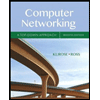
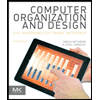
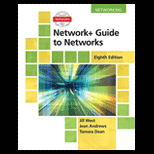
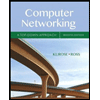
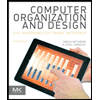
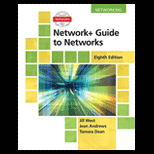
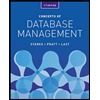
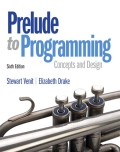
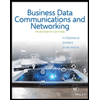