public class BinarySearch2{ public static void main(String[] args) { int[] myList = {20, 12, 10, 7, 5, 3, 2); int result = binarySearch2 (myList, 20); if (result == -1) System.out.println("Not found!"); else System.out.println("The index of the input key is " + result+ " :
public class BinarySearch2{ public static void main(String[] args) { int[] myList = {20, 12, 10, 7, 5, 3, 2); int result = binarySearch2 (myList, 20); if (result == -1) System.out.println("Not found!"); else System.out.println("The index of the input key is " + result+ " :
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Create a BinarySearch2.java to implement the binary search on a descending array. Create a
method for the binary search: “binarySearch2” in BinarySearch2.java. Implement your own code
in Eclipse. ensure it runs without errors.
![Learning X
← → C
A-Z Data X
44°F
Mostly cloudy
CPS 2231 Lab3.pdf
JSTOR H XE Science X PQ Represer X
kean.instructure.com/courses/12224/assignments/56685
231:02 > Assignments
B
9
}
}
else
|
▬▬
E Result Li X
public class BinarySearch2 [{
public static void main(String[] args) {
int[] myList = {20, 12, 10, 7, 5, 3, 2};
int result = binarySearch2 (myList, 20);
if (result == -1)
System.out.println("Not found!");
System.out.println("The index of the input key is " + result+ ".");
O Search
Connect X
}
public static int binarySearch2 (int[] list, int key) {
// finish the binary search in descending order array here.
Lab 3: 1- X b Success x
a
Page <
4 > of 6
New Tab x +
Download
RO
i Info
ZOOM
+
X Close
4x O
12:07 AM
2/9/2023
X](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F2ae76079-938b-4b5d-8ceb-1dfbc10f88ce%2Fb23d9d36-5375-4831-ad48-26627e0b1688%2Fxfe3bo_processed.png&w=3840&q=75)
Transcribed Image Text:Learning X
← → C
A-Z Data X
44°F
Mostly cloudy
CPS 2231 Lab3.pdf
JSTOR H XE Science X PQ Represer X
kean.instructure.com/courses/12224/assignments/56685
231:02 > Assignments
B
9
}
}
else
|
▬▬
E Result Li X
public class BinarySearch2 [{
public static void main(String[] args) {
int[] myList = {20, 12, 10, 7, 5, 3, 2};
int result = binarySearch2 (myList, 20);
if (result == -1)
System.out.println("Not found!");
System.out.println("The index of the input key is " + result+ ".");
O Search
Connect X
}
public static int binarySearch2 (int[] list, int key) {
// finish the binary search in descending order array here.
Lab 3: 1- X b Success x
a
Page <
4 > of 6
New Tab x +
Download
RO
i Info
ZOOM
+
X Close
4x O
12:07 AM
2/9/2023
X
![Learning X
← → C
A-Z Data X
44°F
Mostly cloudy
CPS 2231 Lab3.pdf
JSTOR H XE Science X PQ Represer X
kean.instructure.com/courses/12224/assignments/56685
231:02 > Assignments
Hint:
E Result Li X
In the ascending order case, our logic is as follows:
int mid
if (key list [mid])
(low + high) / 2;
O Search
Connect X
high = mid - 1;
else if (key == list [mid])
return mid;
else
low mid + 1;
In the descending order case, what should our logic be like?
median is the average of the two middle values
I
a
Lab 3: 1- X b Success x
Page <
TASK 5: Write a Java program to find the median of all elements in an array of integers. The
median is the middle value in an ordered set of numbers. If the number of elements is even, the
5
of 6
New Tab x +
Download
......
RO
i Info
ZOOM +
X Close
4x O
12:07 AM
2/9/2023
X](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F2ae76079-938b-4b5d-8ceb-1dfbc10f88ce%2Fb23d9d36-5375-4831-ad48-26627e0b1688%2Fh7artgxi_processed.png&w=3840&q=75)
Transcribed Image Text:Learning X
← → C
A-Z Data X
44°F
Mostly cloudy
CPS 2231 Lab3.pdf
JSTOR H XE Science X PQ Represer X
kean.instructure.com/courses/12224/assignments/56685
231:02 > Assignments
Hint:
E Result Li X
In the ascending order case, our logic is as follows:
int mid
if (key list [mid])
(low + high) / 2;
O Search
Connect X
high = mid - 1;
else if (key == list [mid])
return mid;
else
low mid + 1;
In the descending order case, what should our logic be like?
median is the average of the two middle values
I
a
Lab 3: 1- X b Success x
Page <
TASK 5: Write a Java program to find the median of all elements in an array of integers. The
median is the middle value in an ordered set of numbers. If the number of elements is even, the
5
of 6
New Tab x +
Download
......
RO
i Info
ZOOM +
X Close
4x O
12:07 AM
2/9/2023
X
Expert Solution

Step 1
Program Approach:-
1. Create the class BinarySearch2
2. Declare and initialize the array whose name is mylist
3. Call the function binarysearch2(mylist, 20)
4. Display the output
5. Create the user-defined function whose name is binarysearch2(int[] list, int key)
- Declare and initialize the variable
- int low1=0
- int high1=list.length-1
- Use the while loop to check the condition high1>=low1 when condition is true then go to step a otherwise go to step g
- a) mid1=(low1+high1)/2
- b) check the condition key<list[mid1] when condition is true then go to step c otherwise go to step d
- c) low1 = mid1+1
- d) check the condition key == list[mid1] when condition is true then go to step e otherwise go to step f
- e) return mid1
- f) high1=mid1-1
- g)return -1
Step by step
Solved in 4 steps with 1 images

Recommended textbooks for you
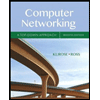
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
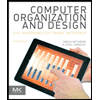
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
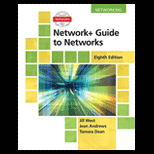
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
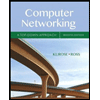
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
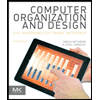
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
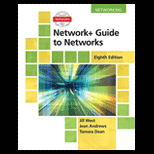
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
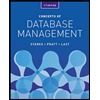
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
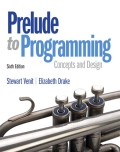
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
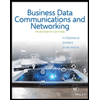
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY