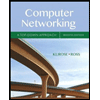
Programming Language: C++
Please use the resources included and provide notes for understanding. Thanks in advance.
Code two functions to fill an array with the names of every World Series-winning team from 1903 to 2020, then output each World Series winner with the number of times the team won the championship as well as the years they won them. The input file is attached, along with the main function and screenprint. Please note team names that include two words, such as Red Sox, have an underscore in place of the space. This enables you to use the extraction operator with a single string variable.
The following resources are included:
Here is main.
#include <iostream>
#include <fstream>
#include<string>
using namespace std;
// Add function declarations and documentation here
void fill(string teams[], int size);
void findWinner(string teams[], int size);
int main()
{
const int SIZE = 118;
int lastIndex;
string team[SIZE];
fill(team, SIZE);
findWinner(team, SIZE);
return 0;
}
// Add function definitions here
Here is your input file. It contains the list of all World Series winners by year in ascending order. The first World Series was played in 1903.
WorldSeriesChampions.txt
Americans
No_Series
Giants
White_Sox
Cubs
Cubs
Pirates
Athletics
Athletics
Red_Sox
Athletics
Braves
Red_Sox
Red_Sox
White_Sox
Red_Sox
Reds
Indians
Giants
Giants
Yankees
Senators
Pirates
Cardinals
Yankees
Yankees
Athletics
Athletics
Cardinals
Yankees
Giants
Cardinals
Tigers
Yankees
Yankees
Yankees
Yankees
Reds
Yankees
Cardinals
Yankees
Cardinals
Tigers
Cardinals
Yankees
Indians
Yankees
Yankees
Yankees
Yankees
Yankees
Giants
Dodgers
Yankees
Braves
Yankees
Dodgers
Pirates
Yankees
Yankees
Dodgers
Cardinals
Dodgers
Orioles
Cardinals
Tigers
Mets
Orioles
Pirates
Athletics
Athletics
Athletics
Reds
Reds
Yankees
Yankees
Pirates
Phillies
Dodgers
Cardinals
Orioles
Tigers
Royals
Mets
Twins
Dodgers
Athletics
Reds
Twins
Blue_Jays
Blue_Jays
Strike_cancelled_series
Braves
Yankees
Marlins
Yankees
Yankees
Yankees
Diamondbacks
Angels
Marlins
Red_Sox
White_Sox
Cardinals
Red_Sox
Phillies
Yankees
Giants
Cardinals
Giants
Red_Sox
Giants
Royals
Cubs
Astros
Red_Sox
Nationals
Dodgers
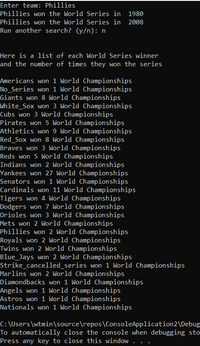

Step by stepSolved in 3 steps with 5 images

- In visual basic Write the code that will sequentially search through the array strFirstNames and will print out the word "Found" if the name "Brian" is in the array.arrow_forward1- Arrays can be created to store values of multiple data types at one place. True False 2- To copy the contents of one array to another you must copy the individual array elements. True Falsearrow_forwardAssume names is an Integer array with 20 elements. Use pseduocode design a for loop that displays each element of the array. Please clearly declare all variables and constants if needed and provide comments.arrow_forward
- the language is c++ the bold is the user input , code asks the user what the width and height of the array should be using arrays and loops for this codearrow_forwardPlease answer this question in 10 mins I will upvote your answer. Create a structure called student that has fields for name, student id, age, score, and grade. The field name is a char array, student id is an integer, age is an integer, score is a float, grade is a character. The maximum value of a score is 100.00 and you should assign a grade to each student based on their scores. Based on user input n, enter the student details of n students, and display their details. You should also report the mean score of the students and report the number of students for each grade (for e.g.: No of students who secured A+: 5, No of students who secured A:4arrow_forwardThe logic will allow the user to: Load a single dimensional array of size 50 with a random number The random number will range from 1 to 1,000 (you may have duplicate values) Find the highest value and the index location that it was in Find the smallest value and the index location that it was in Display the array’s contents. Display the highest value and its index location Display the lowest value and its index location Allow the user to execute this application multiple times (some sort of loop?) You will need one for loop to load the array as well as one or two for loops to search that array. The rand also has a “nasty” tendency to create the same results repeatedly in an exe. To avoid that, we have to “shuffle the deck” every time. This ensures that all numbers are an equal probability of appearing and not the same set of values (would create a boring game). // Add this to "shuffle the deck" every time to ensure that // different values could occur else the exe produces the…arrow_forward
- Assume you have declared an integer array named salary that contains exactly five elements. Using C++ programming language, Write a single statement to assign the value 25000 to the third element of this arrayarrow_forwardIn visual basic Create an array that will hold 10 integer values.arrow_forwardC++ Programming Code two functions to fill an array with the names of every World Series-winning team from 1903 to 2020, then output each World Series winner with the number of times the team won the championship as well as the years they won them. The input file is attached, along with the main function and screenprint. Please note team names that include two words, such as Red Sox, have an underscore in place of the space. This enables you to use the extraction operator with a single string variable. The following resources are included: Here is main. #include <iostream>#include <fstream>#include<string> using namespace std; // Add function declarations and documentation here void fill(string teams[], int size);void findWinner(string teams[], int size); int main(){ const int SIZE = 118;int lastIndex;string team[SIZE]; fill(team, SIZE);findWinner(team, SIZE); return 0;} // Add function definitions here WorldSeriesChampions.txt…arrow_forward
- C++ you have to you use #include <random> also included a sample output has too match the layout Thank youarrow_forwardThe program should allow the user to enter the age of the child and the number of days per week the child will be at the day care center. The program should output the appropriate weekly rate. The file provided for this lab contains all of the necessary variable declarations, except the two-dimensional array. You need to write the input statements and the code that initializes the two-dimensional array, determines the weekly rate, and prints the weekly rate. Comments in the code tell you where to write your statements. 1. Open the source code file named DayCare.cpp using Notepad or the text editor of your choice. 2. Declare and initialize the two-dimensional array. 3. Write the C++ statements that retrieve the age of the child and the number of days the child will be at the day care center. 4. Determine and print the weekly rate. 5. Save this source code file in a directory of your choice, and then make that directory your working directory. 6. Compile the source code…arrow_forwardVisual basic 6.0arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
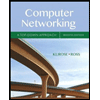
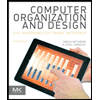
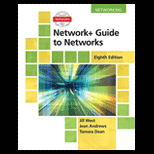
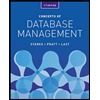
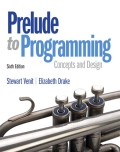
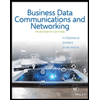