Please write a full code in JAVA for this: A function foo has k integers as input arguments, i.e., foo(int n1, int n2, ..., int nk). Each argument may belong to a different equivalence class, which are stored in an Eq.txt file. In the file, the nth row describes the nth input. Take the second row for example. The data 1, 10; 11, 20; 21, 30 indicates that n2 has three equivalence classes separated by the semi-colons. There is an internal method “int check(int n)” that returns the equivalence class n is in. The result of check(n2 = 3) will be 1 and check(n2 = 25) will be 3. Regarding the function foo, it computes the sum of the returned values by the check function for all input arguments. Follow the Eq.txt file to automatically create test cases for Strong Normal Equivalence class testing. The input argument values are randomly generated. Store your prepared test cases to a test.txt file. Each test case comes with an expected output at the end. All values are delimited by a comma. Eq.txt: 1, 15; 16, 30 1, 10; 11, 20; 21, 30 1, 5; 6, 10; 11, 15 1, 3; 4, 6; 7, 9; 10, 12 1, 12; 13, 24
Please write a full code in JAVA for this:
A function foo has k integers as input arguments, i.e., foo(int n1, int n2, ..., int nk). Each
argument may belong to a different equivalence class, which are stored in an Eq.txt file. In the file,
the nth row describes the nth input. Take the second row for example. The data 1, 10; 11, 20; 21, 30
indicates that n2 has three equivalence classes separated by the semi-colons. There is an internal
method “int check(int n)” that returns the equivalence class n is in. The result of check(n2 = 3) will
be 1 and check(n2 = 25) will be 3. Regarding the function foo, it computes the sum of the returned
values by the check function for all input arguments.
Follow the Eq.txt file to automatically create test cases for Strong Normal Equivalence class testing.
The input argument values are randomly generated. Store your prepared test cases to a test.txt file.
Each test case comes with an expected output at the end. All values are delimited by a comma.
Eq.txt:
1, 15; 16, 30
1, 10; 11, 20; 21, 30
1, 5; 6, 10; 11, 15
1, 3; 4, 6; 7, 9; 10, 12
1, 12; 13, 24

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

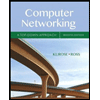
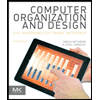
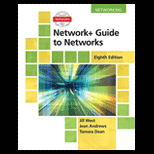
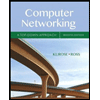
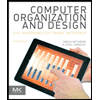
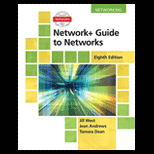
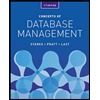
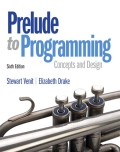
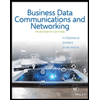