Please modify this program ASAP BECAUSE the program down below does not pass all the test cases when I upload it to hypergrade. The program must pass the test case when uploaded to Hypergrade.
JAVA
Please modify this program ASAP BECAUSE the program down below does not pass all the test cases when I upload it to hypergrade. The program must pass the test case when uploaded to Hypergrade.
import java.util.HashMap;
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.util.Scanner;
public class MorseEncoder {
private static HashMap<Character, String> codeMappings = new HashMap<>();
public static void main(String[] args) {
initializeMappings();
Scanner textScanner = new Scanner(System.in);
System.out.println("Please enter a string to convert to Morse code::");
String textForEncoding = textScanner.nextLine().toUpperCase();
if ("ENTER".equals(textForEncoding)) {
System.out.println();
return;
}
String encodedOutput = encodeText(textForEncoding);
System.out.println(encodedOutput);
}
private static void initializeMappings() {
try (BufferedReader mappingFile = new BufferedReader(new FileReader("morse.txt"))) {
String lineContent;
while ((lineContent = mappingFile.readLine()) != null) {
if(lineContent.length() < 5) {
continue;
}
char alphaChar = lineContent.charAt(0);
String morseString = lineContent.substring(4);
codeMappings.put(alphaChar, morseString);
}
} catch (IOException ioEx) {
ioEx.printStackTrace();
}
}
private static String encodeText(String textForEncoding) {
StringBuilder encodedStringBuilder = new StringBuilder();
for (char individualChar : textForEncoding.toCharArray()) {
if (individualChar == ' ') {
encodedStringBuilder.append(" ");
continue;
}
String morseSymbol = codeMappings.get(individualChar);
if (morseSymbol != null) {
encodedStringBuilder.append(morseSymbol);
encodedStringBuilder.append(' ');
}
}
return encodedStringBuilder.toString();
}
}
0 -----
1 .----
2 ..---
3 ...--
4 ....-
5 .....
6 -....
7 --...
8 ---..
9 ----.
, --..--
. .-.-.-
? ..--..
A .-
B -...
C -.-.
D -..
E .
F ..-.
G --.
H ....
I ..
J .---
K -.-
L .-..
M --
N -.
O ---
P .--.
Q --.-
R .-.
S ...
T -
U ..-
V ...-
W .--
X -..-
Y -.--
Z --..
Test Case 1
ENTER
\n
Test Case 2
abcENTER
.- -... -.-. \n
Test Case 3
This is a sample string 1234.ENTER
- .... .. ... .. ... .- ... .- -- .--. .-.. . ... - .-. .. -. --. .---- ..--- ...-- ....- .-.-.- \n



Step by step
Solved in 5 steps with 9 images

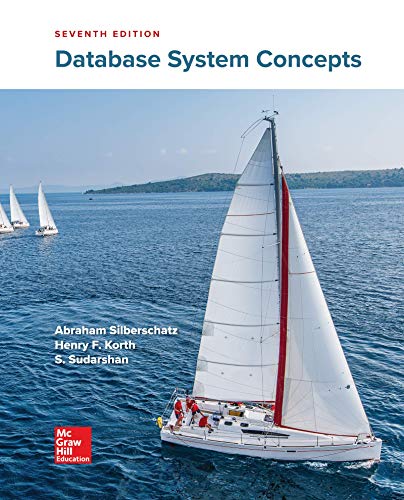
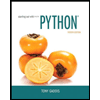
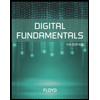
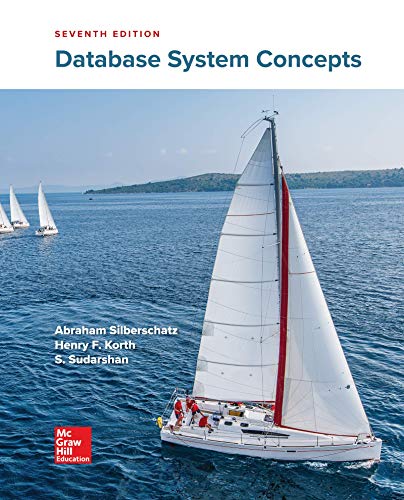
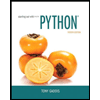
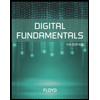
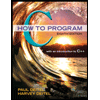
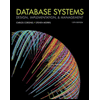
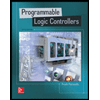