create a "UML Class Diagram" with the files below. I attempted to mae it myself, but I dont know if its right or not. Application.java: package com.mycompany.csc325_abstractfactorydesignpattern; import java.awt.print.Paper; /** * * @author MoaathAlrajab */ public class Application { //Application to describe a publication privateWrittenPiecepaper ; privateIllustrationdrawing; publicApplication(WritingFactoryfactory) { drawing = factory.drawSomething(); paper = factory.writeSomething(); } publicvoidrevealContent(){ paper.typeOfPiece(); drawing.depictInfo(); } } Book.java: package com.mycompany.csc325_abstractfactorydesignpattern; /** * * @author MoaathAlrajab */ public class Book implements WrittenPiece { @Override publicvoidtypeOfPiece() { System.out.println("Book is a written piece"); } } Charts.java: package com.mycompany.csc325_abstractfactorydesignpattern; /** * * @author MoaathAlrajab */ public class Charts implements Illustration { @Override publicvoiddepictInfo() { System.out.println("Charts is a type of illustration usually included+" + "in written pieces such as books and articles. "); } } ComicBookFactory.java: package com.mycompany.csc325_abstractfactorydesignpattern; /** * * @author MoaathAlrajab */ public class ComicBookFactory implements WritingFactory { @Override publicIllustrationdrawSomething() { returnnewHandDrawing(); } @Override publicWrittenPiecewriteSomething() { returnnewBook(); } } DriverClass.java: package com.mycompany.csc325_abstractfactorydesignpattern; /** * * @author MoaathAlrajab */ public class DriverClass { privatestaticApplicationconfigureApplication(){ Applicationapp; WritingFactoryfactory; StringprintName = "Check the config file for cominc"; if (printName.contains("paper")) { factory = newComicBookFactory(); app = newApplication(factory); } else { factory = newMenuscriptFactory(); app = newApplication(factory); } returnapp; } publicstaticvoidmain(String[] args) { Applicationapp = configureApplication(); app.revealContent(); } } Essay.java package com.mycompany.csc325_abstractfactorydesignpattern; /** * * @author MoaathAlrajab */ public class Essay implements WrittenPiece { @Override publicvoidtypeOfPiece() { System.out.println("Essay is a written piece"); } } HandDrawing.java:/ package com.mycompany.csc325_abstractfactorydesignpattern; /** * * @author MoaathAlrajab */ public class HandDrawing implements Illustration { @Override publicvoiddepictInfo() { System.out.println("Hand drawing is traditionally the meaning " + "of illustration "); } } Illustration.java: package com.mycompany.csc325_abstractfactorydesignpattern; /** * * @author MoaathAlrajab */ public interface Illustration { voiddepictInfo(); } MenuscriptFactory.java: package com.mycompany.csc325_abstractfactorydesignpattern; /** * * @author MoaathAlrajab */ public class MenuscriptFactory implements WritingFactory { @Override publicIllustrationdrawSomething() { returnnewCharts(); } @Override publicWrittenPiecewriteSomething() { returnnewPublicationPaper(); } } PublicationPaper.java: package com.mycompany.csc325_abstractfactorydesignpattern; /** * * @author MoaathAlrajab */ public class PublicationPaper implements WrittenPiece{ @Override publicvoidtypeOfPiece() { System.out.println("This is a publication article"); } WritingFactory.java: package com.mycompany.csc325_abstractfactorydesignpattern; /** * * @author MoaathAlrajab */ public interface WritingFactory { Illustration drawSomething(); WrittenPiece writeSomething(); } WrittenPiece.java: package com.mycompany.csc325_abstractfactorydesignpattern; /** * * @author MoaathAlrajab */ public interface WrittenPiece { void typeOfPiece(); }
create a "UML Class Diagram" with the files below. I attempted to mae it myself, but I dont know if its right or not.
Application.java:
package com.mycompany.csc325_abstractfactorydesignpattern;
import java.awt.print.Paper;
/**
*
* @author MoaathAlrajab
*/
public class Application {
//Application to describe a publication
privateWrittenPiecepaper ;
privateIllustrationdrawing;
publicApplication(WritingFactoryfactory) {
drawing = factory.drawSomething();
paper = factory.writeSomething();
}
publicvoidrevealContent(){
paper.typeOfPiece();
drawing.depictInfo();
}
}
Book.java:
package com.mycompany.csc325_abstractfactorydesignpattern;
/**
*
* @author MoaathAlrajab
*/
public class Book implements WrittenPiece {
@Override
publicvoidtypeOfPiece() {
System.out.println("Book is a written piece");
}
}
Charts.java:
package com.mycompany.csc325_abstractfactorydesignpattern;
/**
*
* @author MoaathAlrajab
*/
public class Charts implements Illustration {
@Override
publicvoiddepictInfo() {
System.out.println("Charts is a type of illustration usually included+"
+ "in written pieces such as books and articles. ");
}
}
ComicBookFactory.java:
package com.mycompany.csc325_abstractfactorydesignpattern;
/**
*
* @author MoaathAlrajab
*/
public class ComicBookFactory implements WritingFactory {
@Override
publicIllustrationdrawSomething() {
returnnewHandDrawing();
}
@Override
publicWrittenPiecewriteSomething() {
returnnewBook();
}
}
DriverClass.java:
package com.mycompany.csc325_abstractfactorydesignpattern;
/**
*
* @author MoaathAlrajab
*/
public class DriverClass {
privatestaticApplicationconfigureApplication(){
Applicationapp;
WritingFactoryfactory;
StringprintName = "Check the config file for cominc";
if (printName.contains("paper")) {
factory = newComicBookFactory();
app = newApplication(factory);
} else {
factory = newMenuscriptFactory();
app = newApplication(factory);
}
returnapp;
}
publicstaticvoidmain(String[] args) {
Applicationapp = configureApplication();
app.revealContent();
}
}
Essay.java
package com.mycompany.csc325_abstractfactorydesignpattern;
/**
*
* @author MoaathAlrajab
*/
public class Essay implements WrittenPiece {
@Override
publicvoidtypeOfPiece() {
System.out.println("Essay is a written piece");
}
}
HandDrawing.java:/
package com.mycompany.csc325_abstractfactorydesignpattern;
/**
*
* @author MoaathAlrajab
*/
public class HandDrawing implements Illustration {
@Override
publicvoiddepictInfo() {
System.out.println("Hand drawing is traditionally the meaning "
+ "of illustration ");
}
}
Illustration.java:
package com.mycompany.csc325_abstractfactorydesignpattern;
/**
*
* @author MoaathAlrajab
*/
public interface Illustration {
voiddepictInfo();
}
MenuscriptFactory.java:
package com.mycompany.csc325_abstractfactorydesignpattern;
/**
*
* @author MoaathAlrajab
*/
public class MenuscriptFactory implements WritingFactory {
@Override
publicIllustrationdrawSomething() {
returnnewCharts();
}
@Override
publicWrittenPiecewriteSomething() {
returnnewPublicationPaper();
}
}
PublicationPaper.java:
package com.mycompany.csc325_abstractfactorydesignpattern;
/**
*
* @author MoaathAlrajab
*/
public class PublicationPaper implements WrittenPiece{
@Override
publicvoidtypeOfPiece() {
System.out.println("This is a publication article");
}
WritingFactory.java:
package com.mycompany.csc325_abstractfactorydesignpattern;
/**
*
* @author MoaathAlrajab
*/
public interface WritingFactory {
Illustration drawSomething();
WrittenPiece writeSomething();
}
WrittenPiece.java:
package com.mycompany.csc325_abstractfactorydesignpattern;
/**
*
* @author MoaathAlrajab
*/
public interface WrittenPiece {
void typeOfPiece();
}


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

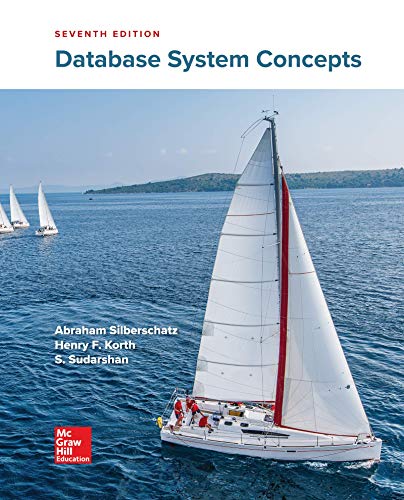
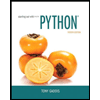
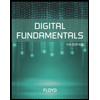
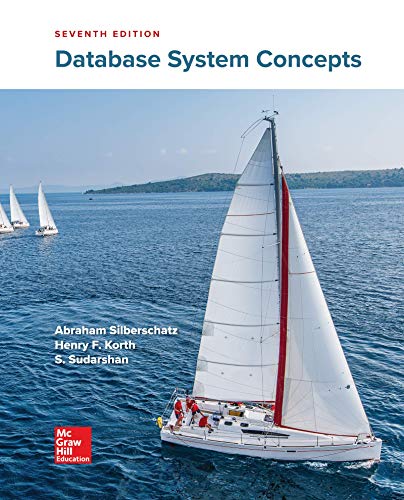
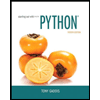
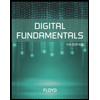
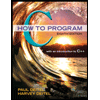
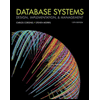
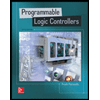