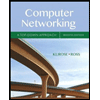
please modify the c language program into a pep 9 assembly language
#include <stdio.h> int num, answer; int divide ( int numer, int denom ) { int quotient, remain; remain = numer; quotient = 0; while ( remain >= denom ) { remain -= denom; quotient++; } // end for return quotient; } // end of divide( ) int modulus ( int n, int d ) { int quot, rem; rem = n; quot = 0; while ( rem >= d ) { rem -= d; quot++; } // end for return rem; } // end of modulus( ) int main() { printf("? "); scanf("%d", &num); answer = divide(num, 20); printf("%d / 20 = %d\n", num, answer); answer = modulus(num, 20); printf("%d mod 20 = %d\n", num, answer); answer = divide(num, 35); printf("%d / 35 = %d\n", num, answer); answer = modulus(num, 35); printf("%d mod 35 = %d\n", num, answer); return 0; } // end of main

Step by stepSolved in 2 steps

- Convert the following c++ code into pep9 assembly language. #include <iostream> using namespace std; void times(int& prod, int mpr, int mcand) { prod = 0; while (mpr != 0) { if (mpr % 2 == 1) prod = prod + mcand; mpr /= 2; mcand *= 2; } } int main(){ int product, n, m; cout << "Enter two numbers: "; cin >> n >> m; times(product, n, m); cout << "Product: " << product << endl; return 0; }arrow_forwardConvert the following c++ code into pep9 assembly language #include <iostream> using namespace std; void times(int& prod, int mpr, int mcand) { prod = 0; while (mpr != 0) { if (mpr % 2 == 1) prod = prod + mcand; mpr /= 2; mcand *= 2; } } int main(){ int product, n, m; cout << "Enter two numbers: "; cin >> n >> m; times(product, n, m); cout << "Product: " << product << endl; return 0; }arrow_forwardChange from psuedocode to source code in C language: START MetGoalCtr = 0 FailedCtr = 0 TotalSales = 0 GET Level while (Level != 'X' && Level != 'x') { switch(Level) { case 'a': case 'A':SalesGoal = 15000.00 break case 'b': case 'B':SalesGoal = 10000.00 break case 'c': case 'C':SalesGoal = 5000.00 break default:SalesGoal =60000.00 } GET ActualSales TotalSales += ActualSales if (ActualSales >= SalesGoal) { ++MetGoalCtr } else { ++FailedCtr } GET Level } DISPLAY TotalSales) DISPLAY MetGoalCtr) DISPLAY FailedCtr) STOParrow_forward
- translate the following C++ program into Pep/9 Assembly. #include<iostream> using namespace std; void showNext(int age){ int nextYr; nextYr = age + 1; cout << "Age:" << age<< endl; cout << "Age next year: " << nextYr << endl;}int main() { int myAge; cout << "Enter age: "; cin >> myAge; showNext (myAge); return 0;}arrow_forwardComplete the following C++ programs into Pep/9 assembly language: 1) int main(){int cop; int driver; cop = 0; driver = 40; while (cop <= driver) { cop += 25; driver += 20; } cout << cop; return 0;}arrow_forwardtranslate a c++ program into pep/9 assembly language #include <iostream> using namespace std; int myAge;void ShowVal(int age){ cout << "Age:" << age << endl;} int main() {cout << "Enter age: ";cin >> myAge;ShowVal(myAge);return 0;}arrow_forward
- Convert the following C++ programs into Pep/9 assembly #include <iostream> using namespace std; void times(int& prod, int mpr, int mcand) { prod = 0; while (mpr != 0) { if (mpr % 2 == 1) prod = prod + mcand; mpr /= 2; mcand *= 2; } } int main(){ int product, n, m; cout << "Enter two numbers: "; cin >> n >> m; times(product, n, m); cout << "Product: " << product << endl; return 0; } Submit: Pep/9 source code along with screen capture showing it running in the Pep simulatorarrow_forwardConvert the following C++ program to pep9/Assembly language BR Main format not machine language #include <iostream>using namespace std; int times(int mpr, int mcand){int prod = 0;while (mpr != 0){if (mpr % 2 == 1)prod = prod + mcand;mpr /= 2;mcand *= 2;} return prod;} int main(){ int n, m; cout << "Enter two numbers: ";cin >> n >> m; cout << "Product: " << times(n, m) << endl; return 0;}arrow_forwardConvert the following to Pep/9 assembly: #include <iostream>using namespace std;int main(){ int number; cin >> number; if (number % 2 == 0) { cout << “Even\n”; } else { cout << “Odd\n”; } return 0;}arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
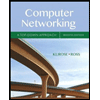
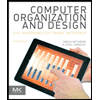
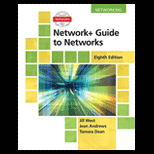
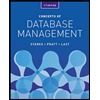
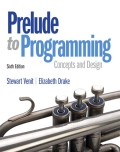
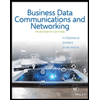