Please help there's an error in my code but I can't figure it out.
Please help there's an error in my code but I can't figure it out.
This is the task:
In this assignment, you will design and program a basic application for a small hardware store. You must
use the Java language to create a utility that implements
(i) the application logic;
(ii) a simple mathematical model for the real problem;
(Ili) basic user interface.
Assume that the hardware store's inventory has products of three types:
- Products of type X, Products of type Y, Products of type Z.
Your program must show the list of available products in the form of a menu and ask the user to choose
the product, its amount, and the planned date of purchasing. Then, the program must calculate and
output the total.
To keep and manage information about a product, you must create a class. The information should include the product’s ID, name, price, and type. Each field must have setters and getters. Create a UML diagram.
Use the input method or hardcoding to test your program with all three types of products. After your program can produce the correct result for each product type, hardcode an inventory of 6-10 products of different types. Note the inventory has a group of objects of the same class, so an array must be used to store the inventory.
Create an input method that prints a menu with the inventory and asks the user to choose a product. Use the array of products as a parameter to pass it to the menu.
Now your program must produce the result according to the user’s choice, requested amount, and the date. For the date input, use three integer numbers 1-12 for a month, 1-31 for a day, and 2022-2050 for a year.
Start development process by planning your data and program organization. Because the primary data
(products) have multiple properties, it is feasible to keep them as objects. Though you can begin with
the program's structure and logic - the program must have a method to read user's data, three methods
to calculate totals, and a method to show the result.
As the first step, program the methods to calculate totals. At this stage, consider direct input (no menu)
or hardcoding of the parameters for each of the three methods and ensure they produce correct results
Use switch or if-else-if to call the method that you want to run.
Here is my code:
import java.util.Scanner;
public class Inventory {
privatestaticfinalintLightBulb60W = 1;
privatestaticfinalintLightBulb100W = 2;
privatestaticfinalintBoltM5 = 3;
privatestaticfinalintBoltM8 = 4;
privatestaticfinalintHose25 = 5;
privatestaticfinalintHose50 = 6;
publicstaticvoidmain(String[]args){
// Project 2
// A basic application for a small hardware store
//Scanner Object
Scannerkeyboard=newScanner(System.in);
// Identifier declarations
intmonth;
intday;
intyear;
Stringcost;
inttotal;
intunits;
// Inventory list
getInventoryList();
// List of products
int[]productTypeList=newint[]{1,2,3,4,5,6};
double[]priceList=newdouble[]{3,5.99,0.15,0.25,10,15};
//Prints out inventory
privatestaticfinalvoidgetInventoryList(){
System.out.println("The Inventory: ");
System.out.println("1 Light Bulb 60W 3.00 X");
System.out.println("2 Light Bulb 100W 5.99 X");
System.out.println("3 Steel Bolt M5 0.15 Y");
System.out.println("4 Steel Bolt M8 0.25 Y");
System.out.println("5 Hose 25 feet 10.00 Z");
System.out.println("6 Hose 50 feet 15.00 Z\n"); {
}
// Asks user to input date of purchase
System.out.print("When do you plan your purchase? \n");
System.out.print("Month of purchase (mm): ");
month = keyboard.nextInt();
System.out.print("Day of purchase (dd): ");
day = keyboard.nextInt();
System.out.print("Year of purchase (yyyy): ");
year = keyboard.nextInt();
// Asks user to input list
System.out.print("Which item number from the list do you desire? \n");
System.out.print("1, 2, 3, 4, 5 or 6?\n");
total = keyboard.nextInt();
System.out.print("Your choice: " + total + "\n");
// Units
System.out.print("How many units do you desire? \n");
units = keyboard.nextInt();
System.out.print("Number of units: ");
// Switch Statement
switch(ProductType)
{
case LightBulb60W:
cost = "3.00";
break;
case LightBulb100W:
cost = "5.99";
break;
case BoltM5:
cost = "0.15";
break;
case BoltM8 :
cost = "0.25";
break;
case Hose25 :
cost = "10.00";
break;
case Hose50:
cost = "15.00";
break;
}
System.out.println(total);
System.out.print("Your total for " + ProductType + " is: " + "$" + cost * units + "\n");
}
}

Step by step
Solved in 3 steps with 1 images

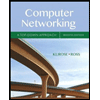
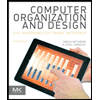
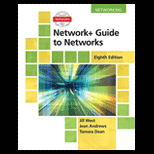
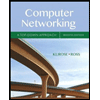
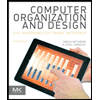
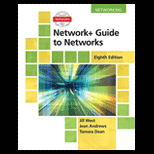
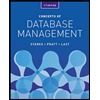
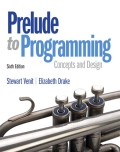
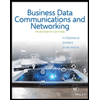