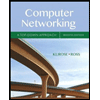
PLEASE HELP IN FUNCTIONAL PROGRAMMING CODE
I have to insert a few lines to generate random numbers and for determining the time taken to get results
(define (insert L M)
(if (null? L) M
(if (null? M) L
(if (< (car L) (car M))
(cons (car L) (insert (cdr L) M))
(cons (car M) (insert (cdr M) L))))))
;; Exp. (insertionsort '(4 2 10 3 -1 5)) ==> (-1 2 3 4 5 10)
(define (insertionsort L)
(if (null? L) '()
insert (list (car L)) (insertionsort (cdr L)))))
(define (random-list n)
(unless (exact-nonnegative-integer? n)
(raise-argument-error 'make-list "exact-nonnegative-integer?" 0 n))
(let loop ([n n] [r '()])
(if (zero? n) r (loop (sub1 n) (cons (random 2147483647) r)))))
Sample function call:
(insertionsort (random-list 10000))

Step by stepSolved in 4 steps with 2 images

- in the C++ version please suppose to have a score corresponding with probabilities at the end and do not use the count[] function. Please explain the detail when coding. DO NOT USE ARRAY. The game of Pig The game of Pig is a dice game with simple rules: Two players race to reach 100 points. Each turn, a player repeatedly rolls a die until either a 1 ("pig") is rolled or the player holds and scores the sum of the rolls (i.e. the turn total). At any time during a player's turn, the player is faced with two decisions: roll - if the player rolls 1: the player scores nothing and it becomes the opponents turn. 2 - 6: the number is added to the player's turn total and the player's turn continues. hold - The turn total is added to the player's score and it becomes the opponent's turn. This game is a game of probability. Players can use their knowledge of probabilities to make an educated game decision. Assignment specifications Hold-at-20 means that the player will choose to roll…arrow_forwardData Structures and Algorithms in C/C++ a) Implement the addLargeNumbers function with the following prototype: void addLargeNumbers(const char *pNum1, const char *pNum2); This function should output the result of adding the two numbers passed in as strings. Here is an example call to this function with the expected output: /* Sample call to addLargeNumbers */ addLargeNumbers("592", "3784"); /* Expected output */ 4376 b) Implement a test program that demonstrates adding at least three pairs of large numbers (numbers larger than can be represented by a long). c) (1 point) Make sure your source code is well-commented, consistently formatted, uses no magic numbers/values, follows programming best-practices, and is ANSI-compliant.arrow_forwardI need help Implement this python function. Please help mearrow_forward
- QUESTION 6 Given the code: void a(int n) { if(n<1) { cout << n*2 < " "; return; } cout << n*2 << " "; a(n-1); } 1. Trace the function when n is 4.arrow_forwardThe programming language is - Python a. Write a function called daysOver that takes three arguments: a dictionary, a location (such as ‘Sydney’, ‘Adelaide’,etc) and a temperature and returns the number of days that were over the given temperature for the given location. For example, if we call the function using the line: total = daysOver(dictionaryData, ‘Adelaide’, 40) total will hold the number of days that Adelaide had a temperature greater than 40 celsius in the data. As a test, there were 54 days over 40 celsius in the data. Check that total is 54 for the example when you run your code. b. Use the daysOver function to print the number of days over 35 celsius for each of the following cities: 'Adelaide','Perth','Melbourne','Canberra','Sydney','Brisbane','Darwin' c. Which of the Australian cities has the most number of days over 35 celsius? My spreadsheet looks like this Since I cannot attach an excel file, my excel file name is "weatherAUS.csv"arrow_forwardFor the following C++ code find and write the recurrence relation. You need to model the runtime of function "Func" in terms of n. (only the recurrence relation in terms of n, No output of the code or final runtime analysis is required) s= array L[] start index e= array L[] end index void Func(int L[], int s, int e) { if (s < e) { i=s-1; for (int j = s; j <= e - 1; j++) { if (A[j] <= x) { i++; swap (&L[i], &L[j]); } } swap (&L[i + 1], &L[e]); int k = i+1; Func(L, s, k - 1); Func(L, k + 1, e); } } Please explain how you get the relation Thank you!arrow_forward
- Describe an efficient way of putting a VECTOR representing a deck of n cards into random order in C++. You may use the function randomlnt(n), which returns a random number between 0 and n-1, inclusive. Your method should guarantee that every possible ordering is equally likely. What is the running time of your function?arrow_forwardPlease use C++ and make sure it's for a sorted array Write a function, removeAll, that takes three parameters: an array of integers,the number of elements in the array, and an integer (say, removeItem). Thefunction should find and delete all of the occurrences of removeItem in thearray. If the value does not exist or the array is empty, output an appropriatemessage. (Note that after deleting the element, the number of elements in thearray is reduced.) Assume that the array is sorted.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
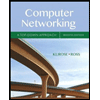
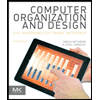
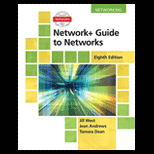
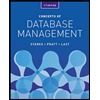
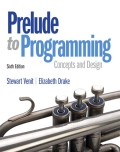
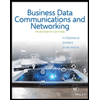