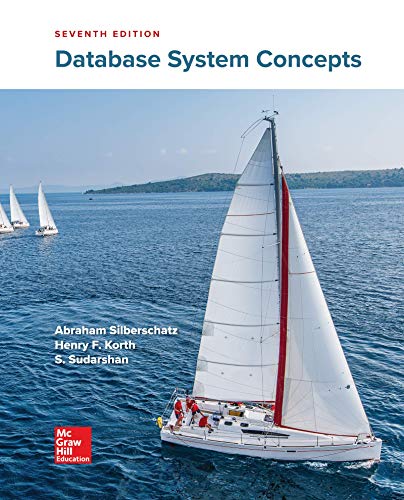
C++ ONLY
Add the following functions to the linked list.
int getSize() -> This function will return the number of elements in the linked-list. This function should work in O(1). For this keep track of a size variable and update it when we insert a new value in the linked-list.
int getValue(index) -> This function will return the value present in the input index. If the index is greater or equal to the size of the linked-list return -1.
void printReverse() -> This function will print the linked list in reverse order. You don’t need to reverse the linked list. Just need to print it in reverse order. You need to do this recursively. You cannot just take the elements in an array or
void swapFirst() -> This function will swap the first two nodes in the linked list. If the linked-list contains less than 2 elements then just do nothing and return.
To check your code add the following code in your main function.
LinkedList l; cout<<l.getSize()<<"\n"; l.InsertAtHead(5); cout<<l.getSize()<<"\n"; l.InsertAtHead(6); l.InsertAtHead(30); cout<<l.getSize()<<"\n"; l.InsertAtHead(20); l.InsertAtHead(30); cout<<l.getValue(2)<<"\n"; cout<<l.getValue(6)<<"\n"; l.printReverse(); l.Traverse(); l.swapFirst(); l.Traverse(); l.printReverse(); |
Output |
0 1 3 30 -1 5 6 30 20 30 30 20 30 6 5 20 30 30 6 5 5 6 30 30 20 |

Step by stepSolved in 3 steps with 1 images

- Topic: Singly Linked ListImplement the following functions in C++ program. Read the question carefully. (See attached photo for reference) void isEmpty() This method will return true if the linked list is empty, otherwise return false. void clear() This method will empty your linked list. Effectively, this should and already has been called in your destructor (i.e., the ~LinkedList() method) so that it will deallocate the nodes created first before deallocating the linked list itself.arrow_forwardQuestion 2: Linked List Implementation You are going to create and implement a new Linked List class. The Java Class name is "StringLinkedList". The Linked List class only stores 'string' data type. You should not change the name of your Java Class. Your program has to implement the following methods for the StringLinked List Class: 1. push(String e) - adds a string to the beginning of the list (discussed in class) 2. printList() prints the linked list starting from the head (discussed in class) 3. deleteAfter(String e) - deletes the string present after the given string input 'e'. 4. updateToLower() - changes the stored string values in the list to lowercase. 5. concatStr(int p1, int p2) - Retrieves the two strings at given node positions p1 and p2. The retrieved strings are joined as a single string. This new string is then pushed to the list using push() method.arrow_forwardCreate a user-defined function called newBoss. This function will move the last node in some singly linked list to the beginning of the linked list (making it the new head). The function takes one argument, a reference to the head of the linked list of type node_t. You may use the following typedef structure. The function returns the new head of the linked list. typedef struct node_s{ int data; struct node_s * nextptr; }node_t;arrow_forward
- Python Write a program that allows the user to add, view, and delete names in an array list. The program must present the user with options (add, view, or delete) to choose from and an option to quit/exit the program. For example: Add adds name(s) to the list. View - view name(s) in the list. Delete - removes name(s) from the list. The user must type the option (Add, View, or Delete), and the program must work accordingly.arrow_forwardstruct insert_at_back_of_sll { // Function takes a constant Book as a parameter, inserts that book at the // back of a singly linked list, and returns nothing. void operator()(const Book& book) { /// TO-DO (3) /// // Write the lines of code to insert "book" at the back of "my_sll". Since // the SLL has no size() function and no tail pointer, you must walk the // list looking for the last node. // // HINT: Do not attempt to insert after "my_sll.end()". // ///// END-T0-DO (3) ||||// } std::forward_list& my_sll; };arrow_forwardIn c++ Please show me the Test output *You may not use any STL containers in your implementations Need help with the following functions: -LinkedList(const LinkedList<T>& other_list) Constructs a container with a copy of each of the elements in another, in the same order. -LinkedList& operator=(const LinkedList& other_list) Replaces the contents of this list with a copy of each element in another, in the same order. -void resize(std::size_t n) resizes the list so that it contains n elements. -void resize(std::size_t n, const T &fill_values) resizes the list so that it contains n elements -void remove(const T &val) Removes from the container all the elements that compare equal to val - bool operator == (const LinkedList &another) Compares this list with another for equality - bool operator != (const LinkedList &another) Compares this list with another for equality CODE #include <iostream> template <typename T> class LinkedList { struct…arrow_forward
- PLEASE DO NOT USE BUILT IN FILES OR FUNCTIONS! Write a program in c++ and make sure it works, that reads a list of students (first names only) from a file. It is possible for the names tobe in unsorted order in the file but they have to be placed in sorted order within the linked list.The program should use a doubly linked list.Each node in the doubly linked list should have the student’s name, a pointer to the next student, and apointer to the previous student. Here is a sample visual. The head points to the beginning of the list. Thetail points to the end of the list.When inserting consider all the following conditions:if(!head){ //no other nodes}else if (strcmp(data, head->name)<0){ //smaller than head}else if (strcmp(data, tail->name)>0){ //larger than tail}else{ //somewhere in the middle} When deleting a student consider all the following conditions:student may be at the head, the tail or in the middleBelow, you will find a sample of what the…arrow_forwardDuplicate Set This function will receive a list of elements with duplicate elements. It should add all of the duplicate elements to a set and return the set containing the duplicate elements. A duplicate element is an element found more than one time in the specified list. The order of the set does not matter. Signature: public static HashSet<Object> duplicateSet(ArrayList<Object> list) Example: INPUT: [2, 4, 5, 3, 3, 5] OUTPUT: {5, 3}arrow_forwardIn C++ pleasearrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
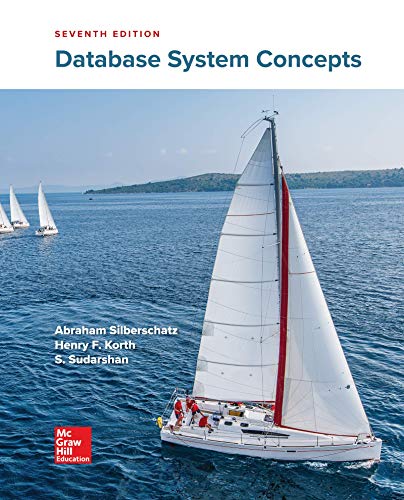
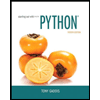
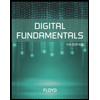
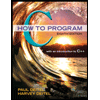
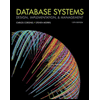
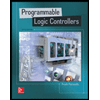