Please follow the steps to complete JAVA PROGRAM Create a class, EmployeeException, that has the following attributes: name: String SSN: String Salary: double The methods required in this class are: A constructor with no arguments that sets the attributes at default values A constructor that passes values for all attributes Accessor, mutator, display method for each attribute. An example of a display method for an attribute is writeOutName(): void, in which you just display the name: System.out.println("Employee Name: " + name); An equals method that has an object of type Employee as argument, and returns true if two employees have the same name, salary, and SSN. Write a driver program for the Employee class that uses an array that can hold up to 100 employees (the array will be of EmployeeException type). However, the user should be free to enter as many employees as needed. The driver class should use two exception classes to signal the user that the SSN entered is not correct. SSN needs to be entered as a 9-digit string without separators. You need to create these two classes: SSNStructureException for when any character in the social security number is not a digit. SSNLengthException for when the social security number entered, without connection characters (dash, or space), has a length other than nine. Download the template file, EmployeeException.txt, and save it as EmployeeException.java public class EmployeeException{ //*** Task #1: define the instance variables// *** Task #2: define constructor with no arguments// *** Task #3: define constructor passing values for all arguments// *** Tasks #4: define accessor, mutator, and write out methods for name attribute// *** Tasks #5: define accessor, mutator, and write out methods for sSN attribute// Display the SSN as ddd-dd-dddd//*** Tasks #6: define accessor, mutator, and write out methods for salary attribute //*** Tasks #7: Define method writeOutput() that display all information about the employee.// *** Tasks #8: Define equals method, having argument of type EmployeeException} Go through the template file and identify the tasks you have to complete, which are clearly marked throughout the file. Complete all the required tasks. Download the template file, EmployeeExceptionDriver.txt, and save it as EmployeeExceptionDriver.java. import java.util.Scanner; public class EmployeeExceptionDriver{ public static void main(String [] args){ //*** Task #1: define the variables required for the program //*** Task #2: define and instantiate variable of type Scanner to be able to read from //*** Task #3: create a loop in which you enter the data for employee. //*** Task #4: inside the loop, instantiate each element of the array with the constructor //*** Task #5: read the name of the employee //*** Task #6: read the salary of the employee //*** Task #7: read SSN using the exceptions blocks //*** Task #8: ask the user if there are more employees to enter //*** Task #9: calculate the average salary //*** Task #10: display the information about all employees with a note if their salary// is above average, under average or average. System.out.println("No more employees."); }} Compile and execute the files.
Please follow the steps to complete JAVA PROGRAM
- Create a class, EmployeeException, that has the following attributes:
- name: String
- SSN: String
- Salary: double
- The methods required in this class are:
- A constructor with no arguments that sets the attributes at default values
- A constructor that passes values for all attributes
- Accessor, mutator, display method for each attribute. An example of a display method for an attribute is writeOutName(): void, in which you just display the name:
System.out.println("Employee Name: " + name); - An equals method that has an object of type Employee as argument, and returns true if two employees have the same name, salary, and SSN.
- Write a driver program for the Employee class that uses an array that can hold up to 100 employees (the array will be of EmployeeException type). However, the user should be free to enter as many employees as needed.
- The driver class should use two exception classes to signal the user that the SSN entered is not correct. SSN needs to be entered as a 9-digit string without separators. You need to create these two classes:
- SSNStructureException for when any character in the social security number is not a digit.
- SSNLengthException for when the social security number entered, without connection characters (dash, or space), has a length other than nine.
- Download the template file, EmployeeException.txt, and save it as EmployeeException.java
-
public class EmployeeException{
-
//*** Task #1: define the instance variables//
-
*** Task #2: define constructor with no arguments//
-
*** Task #3: define constructor passing values for all arguments//
-
*** Tasks #4: define accessor, mutator, and write out methods for name attribute//
-
*** Tasks #5: define accessor, mutator, and write out methods for sSN attribute// Display the SSN as ddd-dd-dddd//***
-
Tasks #6: define accessor, mutator, and write out methods for salary attribute
//*** Tasks #7: Define method writeOutput() that display all information about the employee.//
-
*** Tasks #8: Define equals method, having argument of type EmployeeException}
-
- Go through the template file and identify the tasks you have to complete, which are clearly marked throughout the file. Complete all the required tasks.
- Download the template file, EmployeeExceptionDriver.txt, and save it as EmployeeExceptionDriver.java.
-
import java.util.Scanner;
-
public class EmployeeExceptionDriver{
-
public static void main(String [] args){
-
//*** Task #1: define the variables required for the program
-
//*** Task #2: define and instantiate variable of type Scanner to be able to read from
-
//*** Task #3: create a loop in which you enter the data for employee.
-
//*** Task #4: inside the loop, instantiate each element of the array with the constructor
-
//*** Task #5: read the name of the employee
-
//*** Task #6: read the salary of the employee
-
//*** Task #7: read SSN using the exceptions blocks
-
//*** Task #8: ask the user if there are more employees to enter
-
//*** Task #9: calculate the average salary
-
//*** Task #10: display the information about all employees with a note if their salary// is above average, under average or average.
-
System.out.println("No more employees.");
-
}}
-
- Compile and execute the files.

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

Your program only work for one emplyee. For second emplyee I can not enter name of employee. I need for five emplyoee.
![LT
=-1.8]
ava
ver.java
=-1.8]
ons.java
ava
river.java
E-1.8]
Eclipse File Edit Source Refactor Navigate Search Project Run Window Help
JVC1 - aaaa/src/aaaa/Emplyee Exception Driver.java - Eclipse IDE
▼
Q
EmplyeeExceptionDriver.java X
Problems @ Javadoc
Declaration
Console X
EmployeeException.jav
Chil new cmp cоуеесхсерс
30
57
XXN
58
EmplyeeException Driver [Java Application] /Library/Internet Plug-Ins/Java AppletPlugin.plugin/Contents/Home/bin
59
60
61
62
63
// Task #5: read the name Enter employee #1's name: John
System.out.print("Enter e Enter loyee #1's salary: 2200
nameOf Employee = keyboard
e[i].setName (nameOf Employ
64
65
66
67
Enter employee #1's SSN (9 digits): 123456789
Continue entering employees? (Y for Yes, or N for No)
y
68
69
System.out.println();
Enter employee #2's name:
Enter loyee #2's salary:
70
71
72
// Task #6: read the sala
73
74
75
System.out.print("Enter l
76
salaryOf Employee = keyboa
77
78
e[i].setSalary(salary0fEm
79
80
System.out.println();
81
82
keyboard.nextLine();
83
84
85
86
// Task #7: read SSN usin
87
88
try {
89
90
System.out.print("Ent
91
92
ssnOf Employee = keybo
93
94
95
96
if (ssn0f Employee. lenyilv
31 2
97
98
throw new SSNLengthException (ssn0f Employee, ssn0f Employee.length()): Updates Available
99
100
} else {
101
102
for (int j = 0; j < 9; ++j) {
102
521
JVC
JVC1
PowerPoint
MAY
22
280
الله
(●●
tv
YO
43
X
Software updates have been downloaded.
Click to review and install updates.
Set up Reminder options
OGRAM...
Sun May 22 10:10 AM
N Module 1: Guided Assignment
ASK AN EXPERT
d
ດາ
Photo File
W
CHAT √ MATH
verification.pdf
២
D](https://content.bartleby.com/qna-images/question/5919289c-3531-49df-94d9-1097b7b08cec/5a143102-128e-4c7c-bf17-d32a69a4ba1d/6xn6968_thumbnail.png)
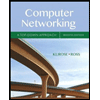
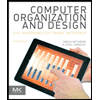
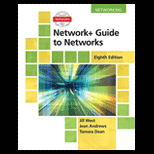
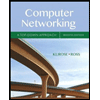
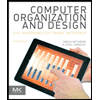
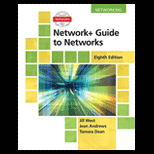
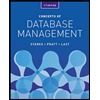
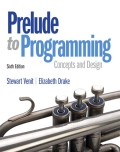
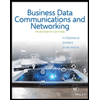