Please fill in the blanks for C, from 1 to 68. /*The program will get the information of each client and print it right back. We could get the information of all clients first, then print them all later for better readability using an array of struct.*/ #include #include #define length 70 //assume there's no names longer than 70 /*It's good practice to add all function headers to the top of the program*/ __1__ __2__ getEmployeeInfo(); __3__ printEmployeeInfo(__4__ __5__ em); __6__ __7__ getClientInfo(__8__ i); __9__ printClientInfo(__10__ __11__ cli, __12__ i); /*Employee struct: all the arrays use the same constant size defined on top name-first, last-(string) title (string) number of clients (integer) number of years working at the company(can take decimal points). */ __13__ employee { __14__ first_name[__15__]; __16__ last_name[__17__]; __18__ title[__19__]; __20__ num_clients; __21__ num_yrs_worked;//3 and a half year would be 3.5 }; /*Client struct: name (first, last) client ID (integer) age (integer) number of products purchased (integer).*/ __22__ client { __23__ first_name[__24__]; __25__ last_name[__26__]; __27__ clientID; __28__ age; }; /*EMPLOYEE This function get an employee's info, save it to the employee struct, and return the employee struct*/ __29__ __30__ getEmployeeInfo() { struct employee emp; printf("\nNeed 2 values. Enter the employee full name (first, then last): "); scanf(" %s %__31__",__32__, emp.last_name); printf("Enter the employee's title: "); scanf(" __33__",__34__); printf("Need 2 numbers. Enter the number of clients, then years of employment:"); scanf("%__35__ %__36__", __37__, __38__); return __39__; } /*This function takes an employee struct and print their info Ex: Hello first_name last_name. Title: def. Number of clients: abc. #years of employment: xyz.*/ __40__ printEmployeeInfo(__41__ __42__ em) { printf("xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx\n"); printf("Printing back Employee Info\n"); printf("\nHello %s %s.\nTitle: %s.\n", em.first_name, em.last_name, em.title); printf("Number of clients:__43__.\n", __44__); printf("Number years of employment: %.1f.\n", em.num_yrs_worked); printf("xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx"); } /*CLIENT*/ /*This function take an index, get a client's info and return the client struct*/ __45__ __46__ getClientInfo(int i) { printf("\nProcessing Client #%d", i+1); struct client cli; printf("\nNeed 2 values. Enter the client full name (first, then last): "); scanf(" __47__ __48__",__49__, __50__); printf("Need 2 numbers. Enter your client ID, followed by your age: "); scanf("__51__ __52__",__53__, __54__); return __55__; } /*This function takes an client struct and print their info: Ex: Hello first_name last_name. Client ID: abc. Age: xyz. */ __56__ printClientInfo(struct client cli, int i) { printf("\n------------------------\n"); printf("Printing Client #%d info: ", i+1); printf("\nHello %__57__ %__58__.\n", __59__, cli.last_name); printf("ClientID: %__60__.\n", __61__); printf("Age: %__62__.", __63__); printf("\n------------------------\n"); } //MAIN int main() { //Create 2 struct for employee, and client in that order struct employee my_emp; struct client my_cli; printf("Hi, I'm here to take some statistics: "); /*Get and print EMPLOYEE's info*/ __64__ = getEmployeeInfo(); printEmployeeInfo(__65__); /*Get and print CLIENTs's info Use \ to break printf to multiple lines without getting errors*/ printf("\n\nNow, let's move on to your client(s). \nAccording to the chart,\ you have %d clients.\n", my_emp.num_clients); //Going through all the clients, get&print each client's info for(int i = 0; i < __66__; i ++) { __67__ = getClientInfo(i); printClientInfo(__68__, i); } printf("\n\nThank you for your patience. You have a great day :)\n"); return 0; }
Please fill in the blanks for C, from 1 to 68.
/*The program will get the information of each client and print it right back. We could get the information of all clients first, then print them all later for better readability using an array of struct.*/
#include<stdio.h>
#include<stdbool.h>
#define length 70 //assume there's no names longer than 70
/*It's good practice to add all function headers
to the top of the program*/
__1__ __2__ getEmployeeInfo();
__3__ printEmployeeInfo(__4__ __5__ em);
__6__ __7__ getClientInfo(__8__ i);
__9__ printClientInfo(__10__ __11__ cli, __12__ i);
/*Employee struct:
all the arrays use the same constant size defined on top
name-first, last-(string)
title (string)
number of clients (integer)
number of years working at the company(can take decimal points). */
__13__ employee
{
__14__ first_name[__15__];
__16__ last_name[__17__];
__18__ title[__19__];
__20__ num_clients;
__21__ num_yrs_worked;//3 and a half year would be 3.5
};
/*Client struct:
name (first, last)
client ID (integer)
age (integer)
number of products purchased (integer).*/
__22__ client
{
__23__ first_name[__24__];
__25__ last_name[__26__];
__27__ clientID;
__28__ age;
};
/*EMPLOYEE
This function get an employee's info,
save it to the employee struct, and
return the employee struct*/
__29__ __30__ getEmployeeInfo()
{
struct employee emp;
printf("\nNeed 2 values. Enter the employee full name (first, then last): ");
scanf(" %s %__31__",__32__, emp.last_name);
printf("Enter the employee's title: ");
scanf(" __33__",__34__);
printf("Need 2 numbers. Enter the number of clients, then years of employment:");
scanf("%__35__ %__36__", __37__, __38__);
return __39__;
}
/*This function takes an employee struct and print their info
Ex: Hello first_name last_name. Title: def. Number of clients: abc. #years of employment: xyz.*/
__40__ printEmployeeInfo(__41__ __42__ em)
{
printf("xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx\n");
printf("Printing back Employee Info\n");
printf("\nHello %s %s.\nTitle: %s.\n", em.first_name, em.last_name, em.title);
printf("Number of clients:__43__.\n", __44__);
printf("Number years of employment: %.1f.\n", em.num_yrs_worked);
printf("xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx");
}
/*CLIENT*/
/*This function take an index, get a client's info and return the client struct*/
__45__ __46__ getClientInfo(int i)
{
printf("\nProcessing Client #%d", i+1);
struct client cli;
printf("\nNeed 2 values. Enter the client full name (first, then last): ");
scanf(" __47__ __48__",__49__, __50__);
printf("Need 2 numbers. Enter your client ID, followed by your age: ");
scanf("__51__ __52__",__53__, __54__);
return __55__;
}
/*This function takes an client struct and print their info:
Ex: Hello first_name last_name. Client ID: abc. Age: xyz. */
__56__ printClientInfo(struct client cli, int i)
{
printf("\n------------------------\n");
printf("Printing Client #%d info: ", i+1);
printf("\nHello %__57__ %__58__.\n", __59__, cli.last_name);
printf("ClientID: %__60__.\n", __61__);
printf("Age: %__62__.", __63__);
printf("\n------------------------\n");
}
//MAIN
int main()
{
//Create 2 struct for employee, and client in that order
struct employee my_emp;
struct client my_cli;
printf("Hi, I'm here to take some statistics: ");
/*Get and print EMPLOYEE's info*/
__64__ = getEmployeeInfo();
printEmployeeInfo(__65__);
/*Get and print CLIENTs's info
Use \ to break printf to multiple lines without getting errors*/
printf("\n\nNow, let's move on to your client(s). \nAccording to the chart,\
you have %d clients.\n", my_emp.num_clients);
//Going through all the clients, get&print each client's info
for(int i = 0; i < __66__; i ++)
{
__67__ = getClientInfo(i);
printClientInfo(__68__, i);
}
printf("\n\nThank you for your patience. You have a great day :)\n");
return 0;
}

#include<stdio.h>
#include<stdbool.h>
#define length 70 //assume there's no names longer than 70
/*It's good practice to add all function headers
to the top of the program*/
struct employee getEmployeeInfo();
void printEmployeeInfo(struct employee em);
struct client getClientInfo(int i);
void printClientInfo(struct client cli, int i);
/*Employee struct:
all the arrays use the same constant size defined on top
name-first, last-(string)
title (string)
number of clients (integer)
number of years working at the company(can take decimal points). */
struct employee
{
char first_name[length];
char last_name[length];
char title[length];
int num_clients;
double num_yrs_worked;//3 and a half year would be 3.5
};
/*Client struct:
name (first, last)
client ID (integer)
age (integer)
number of products purchased (integer).*/
struct client
{
char first_name[length];
char last_name[length];
int clientID;
int age;
};
/*EMPLOYEE
This function get an employee's info,
save it to the employee struct, and
return the employee struct*/
struct employee getEmployeeInfo()
{
struct employee emp;
printf("\nNeed 2 values. Enter the employee full name (first, then last): ");
scanf(" %s %s",emp.first_name, emp.last_name);
printf("Enter the employee's title: ");
scanf("%s",emp.title);
printf("Need 2 numbers. Enter the number of clients, then years of employment:");
scanf("%d %lf", &emp.num_clients, &emp.num_yrs_worked);
return emp;
}
/*This function takes an employee struct and print their info
Ex: Hello first_name last_name. Title: def. Number of clients: abc. #years of employment: xyz.*/
void printEmployeeInfo(struct employee em)
{
printf("xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx\n");
printf("Printing back Employee Info\n");
printf("\nHello %s %s.\nTitle: %s.\n", em.first_name, em.last_name, em.title);
printf("Number of clients:%d.\n", em.num_clients);
printf("Number years of employment: %.1f.\n", em.num_yrs_worked);
printf("xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx");
}
/*CLIENT*/
/*This function take an index, get a client's info and return the client struct*/
struct client getClientInfo(int i)
{
printf("\nProcessing Client #%d", i+1);
struct client cli;
printf("\nNeed 2 values. Enter the client full name (first, then last): ");
scanf(" %s %s",cli.first_name, cli.last_name);
printf("Need 2 numbers. Enter your client ID, followed by your age: ");
scanf("%d %d",&cli.clientID, &cli.age);
return cli;
}
/*This function takes an client struct and print their info:
Ex: Hello first_name last_name. Client ID: abc. Age: xyz. */
void printClientInfo(struct client cli, int i)
{
printf("\n------------------------\n");
printf("Printing Client #%d info: ", i+1);
printf("\nHello %s %s.\n", cli.first_name, cli.last_name);
printf("ClientID: %d.\n", cli.clientID);
printf("Age: %d.", cli.age);
printf("\n------------------------\n");
}
//MAIN
int main()
{
//Create 2 struct for employee, and client in that order
struct employee my_emp;
struct client my_cli;
printf("Hi, I'm here to take some statistics: ");
/*Get and print EMPLOYEE's info*/
my_emp = getEmployeeInfo();
printEmployeeInfo(my_emp);
/*Get and print CLIENTs's info
Use \ to break printf to multiple lines without getting errors*/
printf("\n\nNow, let's move on to your client(s). \nAccording to the chart,\
you have %d clients.\n", my_emp.num_clients);
//Going through all the clients, get&print each client's info
for(int i = 0; i < my_emp.num_clients; i ++)
{
my_cli = getClientInfo(i);
printClientInfo(my_cli, i);
}
printf("\n\nThank you for your patience. You have a great day :)\n");
return 0;
}
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

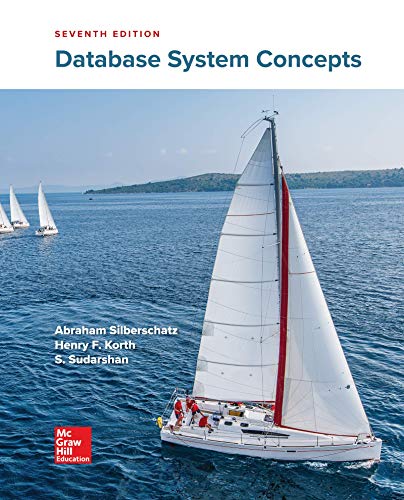
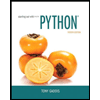
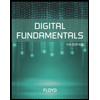
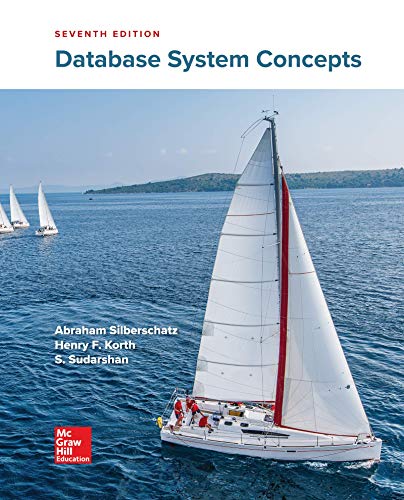
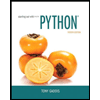
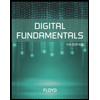
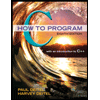
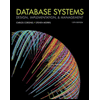
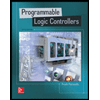