please create a python script for the practice excersie with an explanation. I provided my starter code and my desired outcome in the images. In that file implement the functions listed below by following the guidelines given. Assume the user enters the full name as "First Last". (No need to validate input) generate_EmailID: This function takes the user’s full name in the form “First Last” and creates and returns the email id by using these rules: The email id is all lower case. email id is of the form “last.first@fhs.edu”. e.g. For "John Doe" it will be "doe.john@fhs.edu". See sample runs above. generate_Password: This function takes the user's full name and generates and returns a temporary password by using these rules. The temporary password starts with the first 2 letters of the first name, made lower case. followed by a number which is the product of the lengths of the first and last name followed by a single random letter from these special characters: '!', '@', '#', '$', '%', '*' followed by the last 2 letters of the last name, made upper case. So for "John Doe" possible passwords generated could be: "jo12%OE" or "jo12$OE" See sample runs above. The starter file has a global list of the special characters SPECIAL_CHARS and an import statement which you will find useful.
please create a python script for the practice excersie with an explanation. I provided my starter code and my desired outcome in the images.
In that file implement the functions listed below by following the guidelines given. Assume the user enters the full name as "First Last". (No need to validate input)
generate_EmailID: This function takes the user’s full name in the form “First Last” and creates and returns the email id by using these rules:
The email id is all lower case.
email id is of the form “last.first@fhs.edu”. e.g. For "John Doe" it will be "doe.john@fhs.edu".
See sample runs above.
generate_Password: This function takes the user's full name and generates and returns a temporary password by using these rules. The temporary password
starts with the first 2 letters of the first name, made lower case.
followed by a number which is the product of the lengths of the first and last name
followed by a single random letter from these special characters: '!', '@', '#', '$', '%', '*'
followed by the last 2 letters of the last name, made upper case.
So for "John Doe" possible passwords generated could be: "jo12%OE" or "jo12$OE"
See sample runs above.
The starter file has a global list of the special characters SPECIAL_CHARS and an import statement which you will find useful.
![SPECIAL CHARS =
['!', '@', '#'
'8', '*']
def generate_EmailID (fullname):
''" Generate and return email id.
takes the user's full name in the form "First Last"
Generates and returns email id which
is all lower case.
is of the form "last.first@fhs.edu"
Ex: if fullname is "John Doe", emailID will be "doe.john@fhs.edu".
emailID = ""
pass #IMPLEMENT THIS
return emailID
def generate_Password(fullname):
'''Generates and returns temporary password such that:
The temporary password
starts with the first 2 letters of the first name, made lower case.
followed by a number that's product of the lengths of the first and last name
followed by a single random letter from '!@ # $ %* '
followed by the last 2 letters of the last name, made upper case.
Ex: if fullname is "John Doe" possible passwords generated
could be: "jol2%OE" or "jol2$0E"
passwd
pass #IMPLEMENT THIS
return passwd
def main():
print ("Welcome to Funnyville High School registration")
'y'
while (ans ==
fullname = input ("\nEnter your full name: ").strip()
email = generate EmailID(fullname)
password = generate_Password (fullname)
# Give user email id and password
print ("Your FHS email id: " + email)
print ("Your temporary passwd: "
ans =
'y'):
+ password)
#prompt to check if want to continue
input ("\nContinue? (y/n)").lower ()
ans
print ("Good Bye!")](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F535fabf5-596d-4dc5-9762-a0ef7f611594%2F89719d85-af72-4b89-ad5f-7b10a03012cb%2Fjqpzps_processed.png&w=3840&q=75)


Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

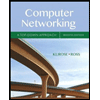
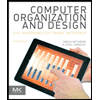
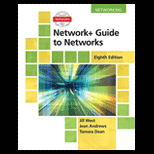
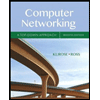
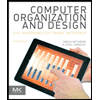
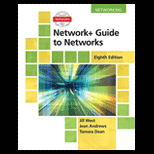
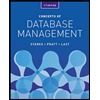
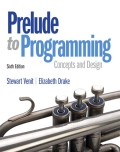
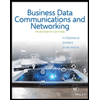