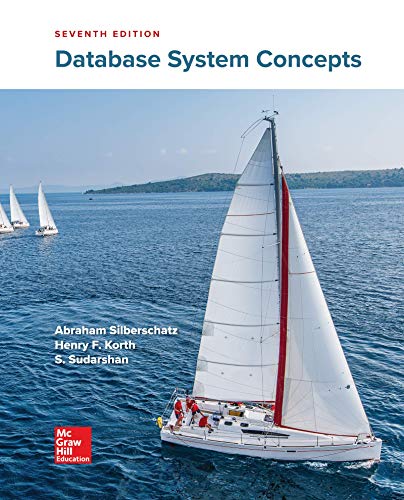
Concept explainers
package lab1;
/**
* A utility class containing several recursive methods
*
* <pre>
*
* For all methods in this API, you are forbidden to use any loops,
* String or List based methods such as "contains", or methods that use regular expressions
* </pre>
*
*
*/
public final class Lab1 {
/**
* This is empty by design, Lab class cannot be instantiated
*/
privateLab1(){
// empty by design
}
/**
* Returns the product of a consecutive set of numbers from <code> start </code>
* to <code> end </code>.
*
* @param start is an integer number
* @param end is an integer number
* @return the product of start * (start + 1) * ..*. + end
* @pre.
* <code> start </code> and <code> end </code> are small enough to let
* this method return an int. This means the return value at most
* requires 4 bytes and no overflow would happen.
*/
publicstaticintproduct(ints,inte){
if(s==e){
returne;
}else{
returns*product(s+1,e);
}
}
publicstaticvoidmain(String[]args){
ints=1;
inte=5;
intresult=product(s,e);
System.out.println("The product is: "+result);
return-999;
}
/**
* This method creates a string using the given char by repeating the character
* <code> n </code> times.
*
* @param first is the given character by which the string is made.
* @param n is the number of characters in the final string
* @return a string made of the given character.
*
* @pre. n is not negative.
*/
publicstaticStringmakeString(charfirst,intn){
returnnull;
charfirst='#';
intn=5;
Stringoutput=makeString(first,n);
System.out.println(output);
}
publicstaticStringmakeString(charch,intn){
if(n<=0){
return"";
}else{
returnch+makeString(ch,n-1);
}
}
/**
* This method accepts a positive integer n and that returns n characters: The
* middle character should always be an asterisk ("*"). If you are writing an
* even number of characters, there will be two asterisks in the middle ("**").
* Before the asterisk(s) you should add less-than characters {@code ("<")}.
* After the asterisk(s) you should add greater-than characters {@code (">")}.
*
* @param n is the total number of strings returned by the method
* @return returns a string made of 1 or 2 stars between a number of
* {@code ("<")} and {@code (">")} characters. The string length should
* be equal to n
* @pre. the integer n should be positive
*/
publicstaticStringwriteChars(intn){
returnnull;
System.out.println(writeChars(1));// Output: *
System.out.println(writeChars(2));// Output: **
System.out.println(writeChars(3));// Output: <*>
System.out.println(writeChars(4));// Output: <**>
System.out.println(writeChars(5));// Output: <<*>>
System.out.println(writeChars(6));// Output: <<**>>
System.out.println(writeChars(7));// Output: <<<*>>>
System.out.println(writeChars(8));// Output: <<<**>>>
}
publicstaticStringwriteChars(intn){
if(n==1){
return"*";
}elseif(n==2){
return"**";
}else{
return"<"+writeChars(n-2)+">";
}
}
}
/**
* This method returns a substring of the given input string that is enclosed in
* two given characters.
*
* @param str is a string that contains at least two characters including
* <code> open </code> and <code> close </code>
* @param open is a character at the beginning of the string that should be
* returned.
* @param close is a character at the end of the string that should be returned.
* @return returns a string enclosed in two given characters of
* <code> open </code> and <code> close </code>.
* @pre. The given str
* contains only one <code> open </code> and one <code> close </code>
* character.
*/
publicstaticStringgetSubstring(Stringinput,charfirst,charsecond){
returnnull;
Stringinput="Hello (there] how are you?";
charfirst='(';
charsecond=']';
Stringoutput=extractEnclosedSubstring(input,first,second);
System.out.println(output);// Output: there
}
publicstaticStringextractEnclosedSubstring(Stringinput,charfirst,charsecond){
intIndexone=input.indexOf(first);
intIndextwo=input.indexOf(second);
if(Indexone!=-1&&Indextwo!=-1&&Indextwo>Indexone){
returninput.substring(Indexone+1,Indextwo);
}else{
returnnull;// Enclosing characters not found or in incorrect order
}
}
}
/**
* This method converts a decimal value into its binary representation.
*
* @param value is a positive integer number
* @return the binary representation of the input.
* @pre. the input is a positive
* integer number.
*/
publicstaticStringdecimalToBinary(intdec){
intdec=23;
Stringbinary=decimalToBinary(dec);
System.out.println(binary);
}
publicstaticStringdecimalToBinary(intn){
if(n==0){
return"0";
}elseif(n==1){
return"1";
}else{
intq=n/2;
intr=n%2;
returndecimalToBinary(q)+Integer.toString(r);
}
}
}
returnnull;
}
heres my code in eclipse IDE, can you please fix the errors because it will not run.
}

The implementation of various common operations such as calculating the product of a range of numbers, constructing a string of repetitive characters, and converting decimal to binary is achieved using recursive techniques in Java. Additionally, the program demonstrates creating a string pattern and extracting a substring enclosed between two specified characters from a given string. The main objective is to showcase the use of recursive methods to solve problems without the use of loops or built-in string/list methods.
Algorithm:
Product of Numbers:
- Base Case: If the start is equal to the end, return the value of end.
- Recursive Case: Multiply the start value with the result of the method called with start incremented by 1.
Create String:
- Base Case: If n is zero or negative, return an empty string.
- Recursive Case: Concatenate the character to the result of the method called with n decremented by 1.
Write Characters:
- Base Case: If n is 1, return "*"; if n is 2, return "**".
- Recursive Case: Return a string with "<", concatenated with the result of the method called with n decremented by 2, concatenated with ">".
Extract Enclosed Substring:
- Locate the indices of the opening and closing characters.
- Extract and return the substring between these characters.
Decimal to Binary:
- Base Case: If n is 0, return "0"; if n is 1, return "1".
- Recursive Case: Divide n by 2 and concatenate the remainder to the result of the method called with the quotient.
Step by stepSolved in 6 steps with 1 images

- Java prgm basedarrow_forwardclass implementation file -- Rectangle.cpp class Rectangle { #include #include "Rectangle.h" using namespace std; private: double width; double length; public: void setWidth (double); void setLength (double) ; double getWidth() const; double getLength() const; double getArea () const; } ; // set the width of the rectangle void Rectangle::setWidth (double w) { width = w; } // set the length of the rectangle void Rectangle::setLength (double l) { length l; //get the width of the rectangle double Rectangle::getWidth() const { return width; // more member functions herearrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
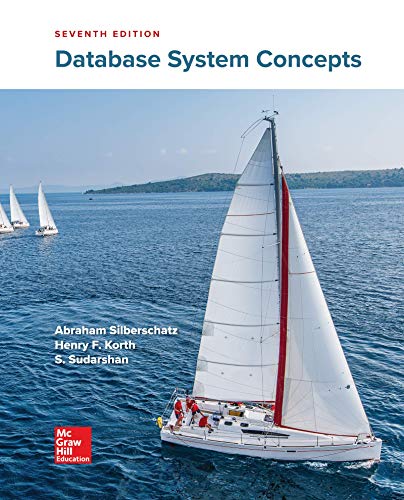
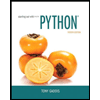
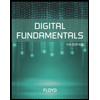
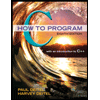
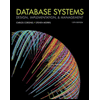
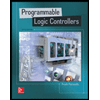