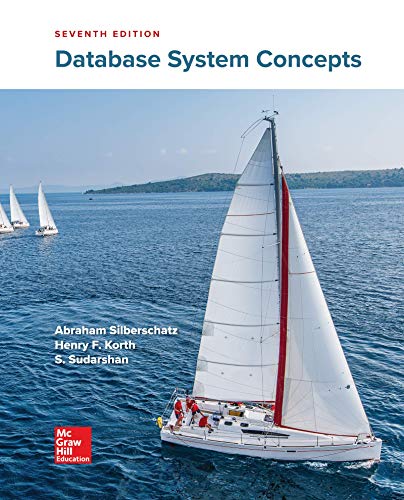
OOP program using c++
- Class Item having the following private attributes: (ID, name, quantity, price) and
the following public methods:
- Constructors (default, parameterized, and copy)
- Setters & Getters
- Operator overloading for the ==, +=,-=, >> and << operators
Note that the ID member variable is not entered or read from the user. It is
automatically set by the class as a serial ID starting with the first item of ID 1 and
incrementing with every new object.
2- Class Seller having the following private attributes: (name, email, items,maxItems),
where items is a dynamic array of objects of type Item with the size maxItems. The
class has the following public methods:
- Constructor (parameterized)
- Operator overloading for the insertion << operators
- Add An Item.
- This will take an Item object as a parameter:
- If the item already exists in the seller's items you will increase the item’s
quantity by the quantity of the parameter item using the (+=) in Item class,
and the price of the parameter object will be ignored. Use the == operator
for this where an item is equal to another if they have the same name.
- Else you will add it to the seller’s items.
- The member function should return a boolean that indicates the successful
addition of item, which will succeed if there is a place in the array and fail
otherwise.
- Sell An Item.
- This will take an item name and a quantity as parameters
- If the quantity is <= item’s quantity you will decrease it from item Using
the (-=) in Item class.
- Else you will print him “There is only {quantity} left for this item”.
- The member function should return a boolean which is true if the item was
found, false otherwise.
- Print Items.
- This will print all the item information for the seller.
- You will print each item using the (<<) op

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Calculator Assignment Modify a calculator program that currently displays the Abstract Syntax Tree (AST) structure after parsing an input expression. Instead of printing the AST, enhance the program to evaluate the expression and display the result. Add support for the binary "%" remainder (modulo) operator and introduce variables with the assignment operator "=". Key steps to implement the assignment operator and variables: Introduce a new static attribute in the AstNode class to represent variables: Add a method isIdentifier() to the TokenStream class to detect variables: Modify the primaryRule() in AstNode to handle variables and variable assignment: Handle new node types in the AstNode evaluate() method for variables and assignment: These changes allow the calculator to evaluate expressions, support variables, and handle variable assignments. Users can create and use variables in subsequent expressions.EmployeeJava Type: package week3;import static week3.MainApp.*; public enum…arrow_forwardImportant requirements for all questions: • All data members must be declared as “private” • No global variable is allowed to be declared and used • Methods within the class and the requested functions cannot have “cin” or “cout” but it should make use of parameters and return value instead. • “cin” and “cout” should be done in main() or any testing functions • Make sure that you clearly show how the C++ class, its methods and all the functions are being called at least twice and print out its return value and its results properly.arrow_forwardall operators that can be overloaded can be implemented as either member functions or regular functions. True Falsearrow_forward
- Using C# language: Programming PLO-2 Measured: Design, implement, and evaluate computer solutions utilizing structured and object-oriented programming methodologies. Design a class named Contractor. The class should keep the following information: Contractor name Contractor number Contractor start date Write one or more constructors and the appropriate accessor and mutator functions for the class.arrow_forwardQ2: Write a c++ program that implements the following UML diagram including functions and constructors' definitions. In addition to the user program code that declares object for each class and show how the derived class object uses the base class members. OOPCourse Student ID: int Grades[5]: int + setgrades(int arr[], int ): void + setID(int): int + setage(int): double + print():void + age:int + OOPstudent() OOPCourse OOPLAB OOPLab Reports[4]: int + setmarks(int arr[), int ): void + print():void + OOPLab()arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
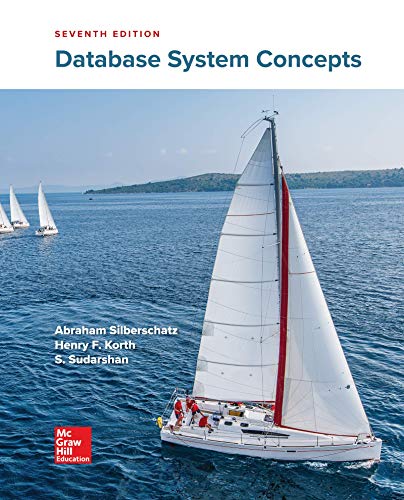
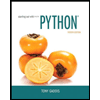
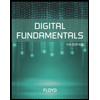
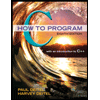
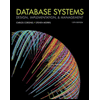
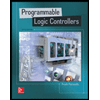