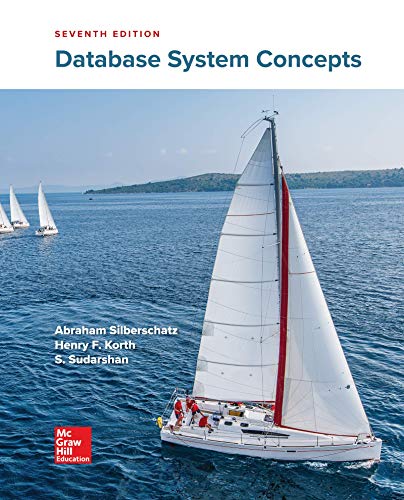
Concept explainers
Calculator Assignment
Modify a calculator
Key steps to implement the assignment operator and variables:
- Introduce a new static attribute in the AstNode class to represent variables:
- Add a method isIdentifier() to the TokenStream class to detect variables:
- Modify the primaryRule() in AstNode to handle variables and variable assignment:
- Handle new node types in the AstNode evaluate() method for variables and assignment:
These changes allow the calculator to evaluate expressions, support variables, and handle variable assignments. Users can create and use variables in subsequent expressions.
EmployeeJava Type:
package week3;
import static week3.MainApp.*;
public enum EmployeeType {
FULL_TIME (EMPLOYEE_FULL_TIME),
PART_TIME (EMPLOYEE_PART_TIME),
CONSULTANT (EMPLOYEE_CONSULTANT);
private int value;
public int getValue() {
return value;
}
private EmployeeType( int value ) {
this.value = value;
}
@Override
public String toString() {
if (this.ordinal() == FULL_TIME.ordinal() ) {
return "FT";
} else if (this.ordinal() == PART_TIME.ordinal() ) {
return "PT";
} else {
return "CONSULTANT";
}
}
}
MAINAPP.java
package week3;
public class MainApp {
public static final int EMPLOYEE_FULL_TIME = 100;
public static final int EMPLOYEE_PART_TIME = 200;
public static final int EMPLOYEE_CONSULTANT = 300;
private static void printEmployeeType(EmployeeType employeeType) {
switch (employeeType) {
case FULL_TIME:
System.out.println(employeeType.getValue());
break;
case PART_TIME:
System.out.println(employeeType.getValue());
break;
case CONSULTANT:
System.out.println(employeeType.getValue());
break;
}
}//printEmployeeType
public static void main(String[] args) {
EmployeeType employeeType = EmployeeType.FULL_TIME;
printEmployeeType( employeeType );
employeeType = EmployeeType.PART_TIME;
printEmployeeType( employeeType );
employeeType = EmployeeType.CONSULTANT;
printEmployeeType( employeeType );
}//main
}//class MainApp

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

- arrow_back Starting Out With Visual C# (5th Edition) 5th Edition Chapter 11, Problem 1PP arrow_back_ios PREVIOUS NEXT arrow_forward_ios Question share_out_linedSHARE SOLUTION Chapter 11, Problem 1PP Program Plan Intro Employee and ProductionWorker Classes Program plan: Design the form: Place a three text boxes control on the form, and change its name and properties to get the employee name, number, and hourly pay rate from the user. Place a four label boxes control on the form, and change its name and properties. Place a two radio buttons control on the form, and change its name and properties. Place a one group box control on the form, and change its name and properties. Place a command button on the form, and change its name and properties to retrieve the object properties and then display the values into label box. In code window, write the code: Program.cs: Include the required libraries. Define the namespace “Program11_1”. Define a class “Program”. Define a constructor for the…arrow_forwardfunctions return the value of a data member. A. Accessor OB. Member C. Mutator OD. Utilityarrow_forwardA(n) is a unique form of class member that enables an object to store and retrieve data.arrow_forward
- The attributes field of objects is a place where data may be saved. The class is solely responsible for its own properties.arrow_forwardCIST 1305 UNIT 10 DROP BOX ASSIGNMENT Create the class diagram and write the pseudocode for a class named Computer that holds the make, model, and amount of memory of a computer. Include methods to set the values for each data field, and include a method that displays all the values for each field.arrow_forwardclass IndexItem { public: virtual int count() = 0; virtual void display()= 0; };class Book : public IndexItem { private: string title; string author; public: Book(string title, string author): title(title), author(author){} virtual int count(){ return 1; } virtual void display(){ /* YOU DO NOT NEED TO IMPLEMENT THIS FUNCTION */ } };class Category: public IndexItem { private: /* fill in the private member variables for the Category class below */ ? int count; public: Category(string name, string code): name(name), code(code){} /* Implement the count function below. Consider the use of the function as depicted in main() */ ? /* Implement the add function which fills the category with contents below. Consider the use of the function as depicted in main() */ ? virtualvoiddisplay(){ /* YOU DO NOT NEED TO IMPLEMENT THIS FUNCTION */ } };arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
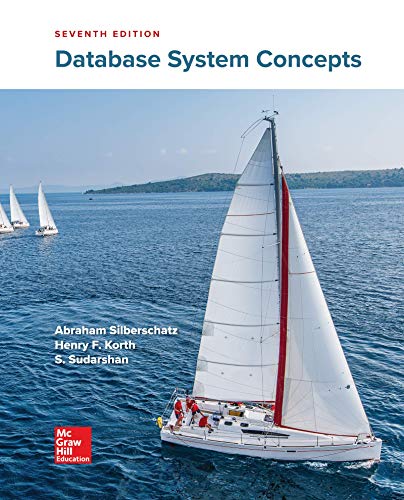
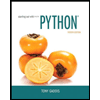
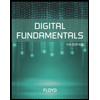
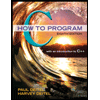
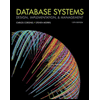
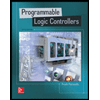