Objective Define a circular buffer data structure and test it. Problem Description: A circular buffer (also called a circular queue) is circular list of nodes where data items are added on one end of the buffer and removed from the other end. Because the nodes form a circular list, the list has no end or beginning: the tail node points to the head node, creating a ring of nodes. You may think of the nodes as containers or slots that are all initially empty but can be assigned a value in their data field info. Every time a new data item is inserted (inserting to a buffer is often referred as Writing), one slot is filled and the buffer has one less empty slot. Every time a data item is removed (referred to as Reading), the buffer has one more empty slot. Since the list has no beginning and no end, a pointer (writeIndex) is used to mark the next empty slot to write to and a second pointer (readIndex) is used to mark the next node to read from. The readIndex/writeIndex must be properly updated with each read/write operation. The read and write operations must also check the buffer status (empty/full) before reading/writing. Watch the following short video for a brief description of a circular buffer: (Links to an external site.)https://www.youtube.com/watch?v=39HHWATPcwY (Links to an external site.). Note also that because data is accessed in a First-In First-Out (FIFO) order, circular buffer is also referred as a circular queue. Define a class that that implements the circular buffer data structure as described above: The file circularBuffer.h below, contains the class specification for the circularBuffer class. Implement each method of the class in the same file. circularBuffer.h Download circularBuffer.h 2. Create a program to test your implementation of class circularBuffer. Use instructions listed in the comments in file testCircularBuffer.cpp to test your implementation. testCircularBuffer.cpp Download testCircularBuffer.cpp Recommendation: You should implement and test class circularBuffer incrementally: start by creating function stubs for all class methods, then implement and test each method one by one. Always start by implementing the class constructor. Deliverables: In a text file, clearly indicate the list of functions of class circularBuffer you implemented successfully. If you don't provide any list, I'll assume none were implemented and you will not get any credit. Submit the text file. Submit a copy of your implementation of class cicularBuffer (cicularBuffer.h) and testCircularBuffer.cpp Submit screenshots of your program runs using the test procedure outlined in testCircularBuffer.cpp to prove your work. Please do NOT submit any zip files. Rubric: Implementation (40 pts): class constructor, destructor, read, and write are worth 7 pts each. Implementation of the other member functions is worth 2 pts each. Testing (55 pts): Each step in the test procedure is worth 5 pts. This is based on screen shots Following programming best practices (5 pt): documentation (author name and date, brief description of program, limitations i.e. what was not completed from the specs), naming conventions, proper code indentation, no global variables, etc.
Objective
Define a circular buffer data structure and test it.
Problem Description:
A circular buffer (also called a circular queue) is circular list of nodes where data items are added on one end of the buffer and removed from the other end. Because the nodes form a circular list, the list has no end or beginning: the tail node points to the head node, creating a ring of nodes. You may think of the nodes as containers or slots that are all initially empty but can be assigned a value in their data field info. Every time a new data item is inserted (inserting to a buffer is often referred as Writing), one slot is filled and the buffer has one less empty slot. Every time a data item is removed (referred to as Reading), the buffer has one more empty slot. Since the list has no beginning and no end, a pointer (writeIndex) is used to mark the next empty slot to write to and a second pointer (readIndex) is used to mark the next node to read from. The readIndex/writeIndex must be properly updated with each read/write operation. The read and write operations must also check the buffer status (empty/full) before reading/writing. Watch the following short video for a brief description of a circular buffer: (Links to an external site.)https://www.youtube.com/watch?v=39HHWATPcwY (Links to an external site.). Note also that because data is accessed in a First-In First-Out (FIFO) order, circular buffer is also referred as a circular queue.
Define a class that that implements the circular buffer data structure as described above:
- The file circularBuffer.h below, contains the class specification for the circularBuffer class. Implement each method of the class in the same file.
- circularBuffer.h Download circularBuffer.h
2. Create a program to test your implementation of class circularBuffer. Use instructions listed in the comments in file testCircularBuffer.cpp to test your implementation.
- testCircularBuffer.cpp Download testCircularBuffer.cpp
Recommendation: You should implement and test class circularBuffer incrementally: start by creating function stubs for all class methods, then implement and test each method one by one. Always start by implementing the class constructor.
Deliverables:
- In a text file, clearly indicate the list of functions of class circularBuffer you implemented successfully. If you don't provide any list, I'll assume none were implemented and you will not get any credit. Submit the text file.
- Submit a copy of your implementation of class cicularBuffer (cicularBuffer.h) and testCircularBuffer.cpp
- Submit screenshots of your program runs using the test procedure outlined in testCircularBuffer.cpp to prove your work. Please do NOT submit any zip files.
Rubric:
- Implementation (40 pts): class constructor, destructor, read, and write are worth 7 pts each. Implementation of the other member functions is worth 2 pts each.
- Testing (55 pts): Each step in the test procedure is worth 5 pts. This is based on screen shots
- Following programming best practices (5 pt): documentation (author name and date, brief description of program, limitations i.e. what was not completed from the specs), naming conventions, proper code indentation, no global variables, etc.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

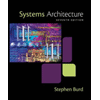
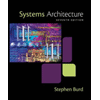