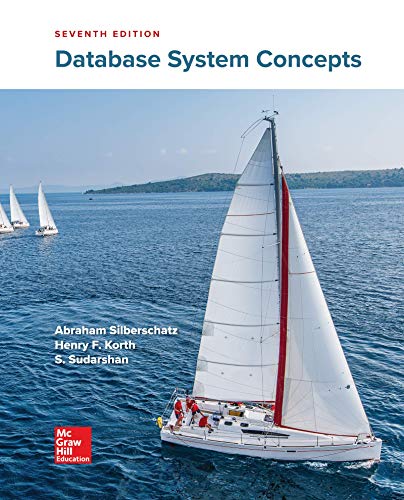
I need the last line sorted in numerical order from greatest to least.
2 4 6 8
1 3 5 7 9
8 6 4 2 1 3 5 7 9
9 7 5 3 1 2 4 6 8
here's the code
#include <iostream>
#include <
using namespace std;
void print(vector<int> v)
{
for (auto i : v)
{
cout << i << " ";
}
cout << endl;
}
void print_reverse(vector<int> v)
{
for (int i = v.size() - 1; i >= 0; i--)
{
cout << v[i] << " ";
}
cout << endl;
}
string convert(string s)
{
for (int i = 0; i < s.size(); i++)
{
if ((s[i] >= 'A' && s[i] <= 'Z') || (s[i] >= 'a' && s[i] <= 'z'))
continue;
else
s[i] = '!';
}
return s;
}
string erase(string s)
{
string ans;
for (int i = 0; i < s.length(); i++)
{
if (s[i] != '!')
ans += s[i];
}
return ans;
}
vector<int> add(vector<int>a, vector<int>b)
{
for (int i = 0; i < b.size(); i++)
{
a.insert(a.begin(), b[i]);
}
return a;
}
int main()
{
vector<int> A;
A.push_back(2);
A.push_back(4);
A.push_back(6);
A.push_back(8);
print(A);
vector<int> B = { 1,3,5,7,9 };
print(B);
B = add(B, A);
print(B);
(B.begin(), B.end());
print_reverse(B);
string C;
cout << "Enter a string: ";
cin >> C;
C = convert(C);
cout << C << endl;
C = erase(C);
cout << C;
cout << endl;
}

We need to write a C++ code to print the last line sorted in numerical order from greatest to least.
Step by stepSolved in 2 steps with 2 images

- complete the //TODOs #include<iostream> #include "swap.h" using namespace std; int main(int argc, char const *argv[]) { intfoo[5] = {1, 2, 3, 4, 5}; cout<<"Addresses of elements:"<<endl; //TODO Print the addresses of array elements cout<<endl; cout<<"Elements:"<<endl;; //TODO Print all the elements using pointers cout<<endl; inta,b; intf; intflag = 1; while(flag == 1){ cout<<"Enter indices of elements you want to swap?"<<endl; cout<<"First index"<<endl; cin>>a; cout<<"Second index"<<endl; cin>>b; cout<<"Enter 0 for pass-by-value, 1 for pass-by-pointer"<<endl; cin>>f; switch (f) { case0: // Pass by Value // Compare the resultant array with the array you get after passing by pointer cout<<"Before swapping"<<endl; for(inti = 0;i<5;i++){ cout<<foo[i]<<" "; } cout<<endl; swap(foo[a],foo[b]); cout<<"\nAfter swapping"<<endl;…arrow_forwardplease help me with codingarrow_forwardC++ Please help!arrow_forward
- Write a value-returning function that receives an array of structs of the type defined below and the length of the array as parameters. It should return as the value of the function the sum of the offspring amounts in the array. Note: you do not need a main function or to create an array. Assume the array already exists from main. #include <iostream>using namespace std; // struct declaration for Animal struct Animal { // a string for animal typestring animal_type;// a string for color string color;// an integer for number of offspring. int number_of_offspring;}; // Main function to declare a structure variable of type Animal. int main(){struct Animal A1;return 0;}arrow_forwardUse iterators to print each element of v separted by spaces. #include <iostream>#include <vector> using namespace std; int main(){vector<int> v = {1, 3, 5}; (.....) }arrow_forwardc++arrow_forward
- 4arrow_forwardWrite a statement that declares a dynamic array of 5 strings and lets a pointer variable p to point to that array A/arrow_forwardI need help on my c++ code i dont know how to properly use " tokennize " #include <iostream> #include <vector> #include <algorithm> #include "Date.h" #include "Person.h" #include "Student.h" #include "Faculty.h" using namespace std; void addStudent(vector<Student>& students); void addFaculty(vector<Faculty>& faculty); void ListCourse(string fName, vector<Student>students, vector<Faculty>faculty); void tokenizeDate(char* cDate, int& month, int& day, int& year); char menu(); int main() { //vectors for storage vector<Student>students; vector<Faculty>faculty; string courseName; //name of course cout << "Enter a name for your course: "; getline(cin, courseName); cout << endl; // getmenu char ch = menu(); //Loop repeat until quit while (ch !='Q') { if (ch=='I'){ addFaculty (faculty); } else if…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
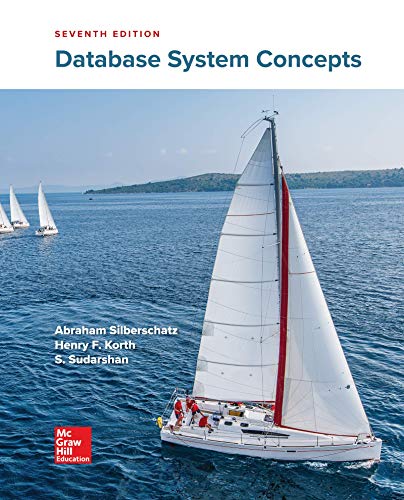
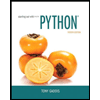
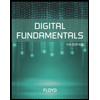
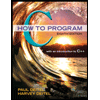
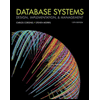
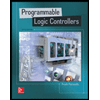