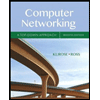
Need help writing the following method: get(int id)
– returns a Student, assuming that the student is either registered for the course or on the waitlist. If there is no student in the table or waitlist that has this id, get() should return null. You should go directly to slot id % m rather than iterating through all the slots.
For context
public class Course {
public String code;
public int capacity;
public SLinkedList<Student>[] studentTable;
public int size;
public SLinkedList<Student> waitlist;
public Course(String code) {
this.code = code;
this.studentTable = new SLinkedList[10];
this.size = 0;
this.waitlist = new SLinkedList<Student>();
this.capacity = 10;
}
public Course(String code, int capacity) {
this.code = code;
this.studentTable = new SLinkedList[capacity];
this.size = 0;
this.waitlist = new SLinkedList<>();
this.capacity = capacity;
}
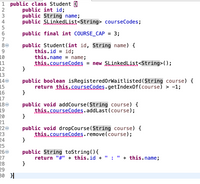

Step by stepSolved in 2 steps

- 25 def iceCreamOrder (cost flavor # vanilla 26 27 28 29 30 31 32 def run(): 33 34 35 36 37 38 = 6): input ("What flavor would you like?\n") return "The flavor print(iceCreamOrder ())] " + flavor + will cost printStatement() print (returnValues()) print (parameters (1,4)) print (parameters (5,7)) print (iceCreamOrder()) return #Do NOT change anything in this line! + str(cost) + " dollars."arrow_forwardCode NOT working. What to do? Here is code: appleList = [["Apple",52,14,0,0],["McIntosh red",80,18,0,0.5],["Gala (Apple)",52,11.4,0.2,0.3],["Fuji Apple",52,11.4,0.2,0.3],["Honey Crisp Apples",90,21,0,0],["Granny Smith Apples",52,11.4,0.2,0.3],["Red Delicious Apples",80,17,0,0],["Braeburn Apples",71.7,16,0.5,0.1],["Golden Delicious Apples",130,29,1,0],["Jonagold",130.7,34,1,0],["Cripps Pink Apple",80,18,0,0],["Empire Apples",80,17,0,0],["Produce Cortland Apples",70,6,1,5],["Jazz Apples",53.8,12,0.6,0.5],["Cameo Apples", 80,22,0,0]["Winesap Apples",80,22,0,0],["Rome Apples",80,22,0,0],["Ambrosia Apples",90,17,0.4,0],] print(":Type of Apple : Calories : Carbs (in GRAMS) : Protein (in GRAMS) : Fat (in GRAMS) :") for item in appleList:print(":",item[0]," "*(9-len(item[0])),":",item[1]," "*(13-len(item[1])),":",item[2]," "*(4-len(str(item[2]))),":")arrow_forwardNeed help writing the following method: getCourseSize() – returns the number of students registered in the course (not in the waitlist). Your code should maintain the public size variable that keeps track of the number of students registered. Note that a successful put() and remove() will only change the size field if the number of students that are registered changes. public class Course { public String code; public int capacity; public SLinkedList<Student>[] studentTable; public int size; public SLinkedList<Student> waitlist; public Course(String code) { this.code = code; this.studentTable = new SLinkedList[10]; this.size = 0; this.waitlist = new SLinkedList<Student>(); this.capacity = 10; } public Course(String code, int capacity) { this.code = code; this.studentTable = new SLinkedList[capacity]; this.size = 0; this.waitlist = new SLinkedList<>();…arrow_forward
- import random; #importing random module aGrades=[]; #list to store grades of all tests for i in range(0,7):aGrades.append(random.randrange(0,100)); #generating 7 random values for testsexamCount=len(aGrades)-1; #examCount is a variable to store count of all exams except last exam totalPoints=sum(aGrades)-aGrades[len(aGrades)-1]; #totalPoints is a variable to store sum of all scores excluding last exam testAverage=(totalPoints)/examCount; #testAverage is a variable used to store the average of scores except last exam finalAverage= (testAverage*0.6)+(aGrades[len(aGrades)-1]*0.4); #finalAverage is a variable to store weighted average of the tests print(aGrades); #printing list of gradesprint(testAverage); #printing test averageprint(finalAverage); #printing final average How do i rewrite the Grades List programs above using a loop instead of referencing the elements of the lists by index.arrow_forwardStrings all_colors and updated_value are read from input. Perform the following tasks: Split all_colors into tokens using a comma (',') as the separator and assign color_list with the result. Replace the first element in color_list with updated_value.arrow_forwardUsing java, answer the following questions about the code below. 1) Create a new function or method that checks to see if an int parameter is present in the list. 2) Create a main() method or JUnit tests to show that your list code functions as intended. (Code) private class Nodeint data;Node next;Node(int data) {this.data = data;this.next = null;}}private Node first;private Node last; public void addToEnd(int data){ Node newNode = new Node(data);if (first == null){first = newNode;last = newNode;} else {last.next = newNode;last = newNode;}}public void printList(){ Node current = first;while (current != null){System.out.print(current.data + " ");current = current.next;}System.out.println();}public static void main(String[] args){node list = new node();list.addToEnd(2);list.addToEnd(4);list.addToEnd(6);list.printList();}}arrow_forward
- please fix code to match "enter patients name in lbs" thank you import java.util.LinkedList;import java.util.Queue;import java.util.Scanner; interface Patient { public String displayBMICategory(double bmi); public String displayInsuranceCategory(double bmi);} public class BMI implements Patient { public static void main(String[] args) { /* * local variable for saving the patient information */ double weight = 0; String birthDate = "", name = ""; int height = 0; Scanner scan = new Scanner(System.in); String cont = ""; Queue<String> patients = new LinkedList<>(); // do while loop for keep running program till user not entered q do { System.out.print("Press Y for continue (Press q for exit!) "); cont = scan.nextLine(); if (cont.equalsIgnoreCase("q")) { System.out.println("Thank you for using BMI calculator"); break;…arrow_forwardCreate a for-each loop that outputs all elements in the role collection of Student objects. What is necessary for that loop to function?arrow_forwardplease complete this method /** * Checks whether an item is inside the set. * @param item to be checked * @return true iff the set contains the item */public boolean contains(T item) {}arrow_forward
- Part 2: Sorting the WorkOrders via dates Another error that will still be showing is that there is not Comparable/compareTo() method setup on the WorkOrder class file. That is something you need to fix and code. Implement the use of the Comparable interface and add the compareTo() method to the WorkOrder class. The compareTo() method will take a little work here. We are going to compare via the date of the work order. The dates of the WorkOrder are saved in a MM-DD-YYYY format. There is a dash '-' in between each part of the date. You will need to split both the current object's date and the date sent through the compareTo() parameters. You will have three things to compare against. You first need to check the year. If the years are the same value then you need to go another step to check the months, otherwise you compare them with less than or greater than and return the corresponding value. If you have to check the months it would be the same for years. If the months are the same you…arrow_forwardPlease create a driver class (java) that contains only a main method which should: Create a new CustomerList obiect Prompt the user to enter a file name Call the getCustomer List method with the file name that the user entered While (true) { Prompt user to enter command; if (command is "a") { Prompt user for customerNumber firstName lastName balance; Create a customerRecord and store it in the database; } else if (command is "f") { Prompt the user to enter a customer number and then displays the corresponding record on the screen; } else if (command is "g") { Prompt the user to enter a file name to save the latest update; Save the information in the database to the file specified by the user; Terminate program; } else { Display error message: } }arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
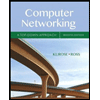
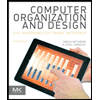
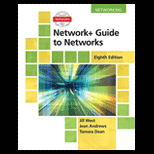
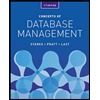
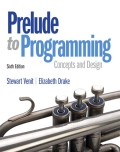
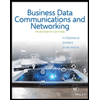