need help with my code i need to ask the user for a filename. Display the oldest car for every manufacturer from that file. If two cars have the same year, compare based on the VIN. my code is not working properly, it is just dipslaying exactly what is in the file that the user inputs
I need help with my code
i need to ask the user for a filename. Display the oldest car for every manufacturer from that file. If two cars have the same year, compare based on the VIN.
my code is not working properly, it is just dipslaying exactly what is in the file that the user inputs
here is the code
import java.io.BufferedReader;
import java.io.FileReader;
import java.util.Comparator;
import java.io.File;
import java.io.FileNotFoundException;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Scanner;
class Car {
private String makeModel;
private int year;
private String VIN;
public Car(String makeModel, int year, String VIN) {
this.makeModel = makeModel;
this.year = year;
this.VIN = VIN;
}
public String getMakeModel() {
return makeModel;
}
public int getYear() {
return year;
}
public String getVIN() {
return VIN;
}
}
class Assignment8_2 {
private static int minIndex(ArrayList<Car> cars, int i, int j) {
if (i == j)
return i;
int k = minIndex(cars, i + 1, j);
if (cars.get(i).getYear() < cars.get(k).getYear()) {
return i;
} else if (cars.get(i).getYear() > cars.get(k).getYear()) {
return k;
} else {
if (cars.get(i).getVIN().compareTo(cars.get(k).getVIN()) < 0) {
return i;
}
return k;
}
}
public static void recursiveSelectionSort(ArrayList<Car> cars, int n, int index) {
if (index == n)
return;
int k = minIndex(cars, index, n - 1);
if (k != index) {
Car temp = cars.get(k);
cars.set(k, cars.get(index));
cars.set(index, temp);
}
recursiveSelectionSort(cars, n, index + 1);
}
}
public class Demo {
public static void main(String[] args) throws FileNotFoundException {
ArrayList<Car> cars = new ArrayList<Car>();
Scanner sc1 = new Scanner(System.in);
System.out.println("Enter filename");
String carFile = sc1.next();
File file = new File(carFile);
try {
Scanner inputFile = new Scanner(file);
cars = new ArrayList<>();
String header = inputFile.nextLine();
while (inputFile.hasNextLine()) {
String line = inputFile.nextLine();
String[] values = line.split("\\s+");
int entries = values.length;
if (entries <= 4) {
System.out.println(line);
continue;
}
int year = Integer.parseInt(values[entries - 2]);
String VIN = values[entries - 1];
String makeModel = "";
for (int i = 0; i < entries - 2; i++) {
makeModel += values[i] + " ";
}
cars.add(new Car(makeModel, year, VIN));
}
inputFile.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
}
assert cars != null && cars.size() >= 1;
Assignment8_2.recursiveSelectionSort(cars, cars.size(), 0);
System.out.println("Oldest cars by make:");
String currentMake = "";
for (Car car : cars) {
if (!currentMake.equals(car.getMakeModel())) {
currentMake = car.getMakeModel();
System.out.printf("%-15s %-20s %d %s\n", currentMake, car.getMakeModel().equals(currentMake) ? "" : car.getMakeModel(), car.getYear(), car.getVIN());
}
}
}
}
it is pulling the information from the file but i need it to display in correct order with results
i.e.THIS IS WHAT NEEDS TO BE DISPLAYED WITH CORRECTED CODE
Enter filename\n
car-list-3.txtENTER
Oldest cars by make\n
Acura Legend 1988 YV1672MK8A2784103\n
Aptera Typ-1 2009 19UUA56952A698282\n
Aston Martin DB9 2006 JTDJTUD36ED662608\n
Audi 5000S 1985 JN1CV6EK1CM209730\n
Austin Mini Cooper 1964 1G6KD57Y86U095255\n
Bentley Continental GT 2006 19UUA655X4A245718\n
BMW 7 Series 1993 1FM5K7B80FG198521\n
Buick LeSabre 1986 5FRYD4H82EB970786\n
Cadillac Fleetwood 1992 WAUSVAFA0AN244068\n
Chevrolet Corvette 1957 WBA3V7C57F5095471\n
Chrysler Town & Country 1994 WBANU53558B610150\n
Daewoo Lanos 1999 2HNYD18412H169358\n
Daihatsu Charade 1992 JH4CL95958C112754\n
Dodge D150 1993 19XFA1F37BE391950\n
Eagle Talon 1993 WUAAU34289N943973\n
Ferrari F430 Spider 2006 5N1AR2MM5EC533806\n
Fiat 500 2012 1G6DL67A880617705\n
Ford Thunderbird 1965 1HGCR2F32DA757676\n
Geo Metro 1992 1G6KE54Y34U777569\n
GMC Suburban 1500 1992 19XFB2F59CE612826\n
Holden VS Commodore 1995 WAUBGBFC7CN714505\n
Honda Civic 1980 JTDKN3DU8A0870976\n
Hummer H1 1999 5FNRL5H20BB474763\n
Hyundai Sonata 2002 JA4AS2AW2DU975492\n
Infiniti Q 1994 WAUDH78E97A027816\n
Isuzu Impulse 1992 WBALM5C5XAE656665\n
........
56 result(s)\n
it is displaying
Enter filename\n
car-list-3.txtENTER
The entry: Volkswagen,GTI,2009,2T1BU4EE3DC621253 is invalid!!\n
The entry: Mazda,Protege,1997,1FTMF1E80AK080813 is invalid!!\n
The entry: Lexus,LS,1999,WUADU78E88N507910 is invalid!!\n
The entry: GMC,Yukon XL 2500,2004,1GKS1EEF3DR855619 is invalid!!\n
The entry: Ford,Club Wagon,1992,JH4CL95994C330559 is invalid!!\n
.........output too long

Step by step
Solved in 3 steps

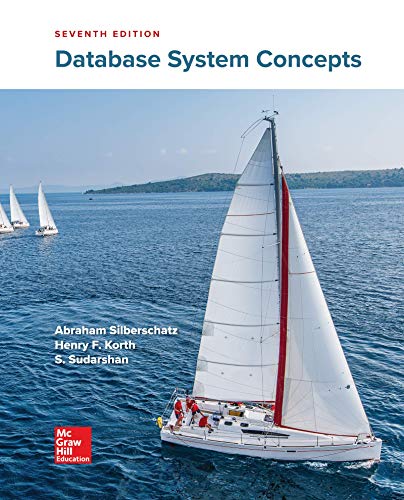
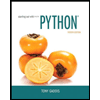
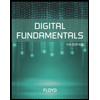
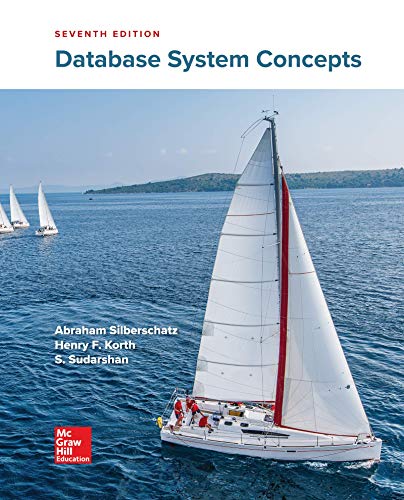
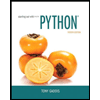
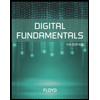
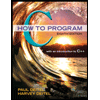
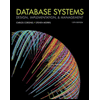
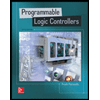