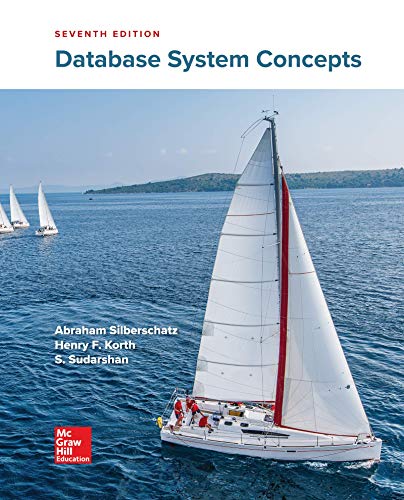
Concept explainers
Need help python
"
Write an Array Data Structure which has a constructor, "len", "str", "itr", "getitem" and "setitem" methods.
Then create an object of this Array class with 6 elements in it. Fill the object of this class with numbers from 3 to 8.
print the array and show the length of the array object."
Here is the array code
"""
File: arrays.py
An Array is a restricted list whose clients can use
only [], len, iter, and str.
To instantiate, use
<variable> = array(<capacity>, <optional fill value>)
The fill value is None by default.
"""
class Array(object):
"""Represents an array."""
def __init__(self, capacity, fillValue = None):
"""Capacity is the static size of the array.
fillValue is placed at each position."""
self.items = list()
for count in range(capacity):
self.items.append(fillValue)
def __len__(self):
"""-> The capacity of the array."""
return len(self.items)
def __str__(self):
"""-> The string representation of the array."""
return str(self.items)
def __iter__(self):
"""Supports traversal with a for loop."""
return iter(self.items)
def __getitem__(self, index):
"""Subscript operator for access at index."""
return self.items[index]
def __setitem__(self, index, newItem):
"""Subscript operator for replacement at index."""
self.items[index] = newItem

Step by stepSolved in 4 steps with 1 images

- Programming language: Processing from Java Question attached as photo Topic: Use of Patial- Full Arraysarrow_forwardWhat’s the difference between an array and Vector?arrow_forwardNO REGEX OR ANYTHING COMPLICATED PLEASE.. USE BASIC APPROACH Create a program in Python that reads data from Breakfast Menu (https://www.w3schools.com/xml/simple.xml) and builds parallel arrays for the menu items, with each array containing the menu item name, description, calories, and price, respectively. After reading the data and building the arrays, display the menu items similar to the following: name - description - calories - priceAt the bottom, display the total number of items on the menu, the average number of calories per item, and the average price per item similar to: 0 items - 0 average calories - $0.00 average price You may either read the page using Internet processing methods, or you may download and save the page and then read the data from the saved file. You must process the data using string functions (no XML libraries). must use separate subroutines/functions/methods to implement each type of processing, and include error handling for missing or invalid…arrow_forward
- 1. Array splitting in Java Create an integer array with 10 integer numbers, initialize the array arbitrarily but make sure there are both positive and negative integers. Then generate two arrays out of the original array, one array with all natural numbers (positive numbers or zeros) and another one with all negative numbers. Print out the number of elements and the detailed elements in each array.arrow_forwardI NEED HELP PLEASE NO REGEX OR ANYTHING COMPLICATED PLEASE.. USE BASIC APPROACH Create a program in Python that reads data from Breakfast Menu (https://www.w3schools.com/xml/simple.xml) and builds parallel arrays for the menu items, with each array containing the menu item name, description, calories, and price, respectively. After reading the data and building the arrays, display the menu items similar to the following: name - description - calories - priceAt the bottom, display the total number of items on the menu, the average number of calories per item, and the average price per item similar to: 0 items - 0 average calories - $0.00 average price You may either read the page using Internet processing methods, or you may download and save the page and then read the data from the saved file. You must process the data using string functions (no XML libraries). must use separate subroutines/functions/methods to implement each type of processing, and include error handling for…arrow_forwardProgram: Using a multidimensional array, create a triangular-shaped array. You will ask the user how many lines they want to see and then create the array, fill it, and then print it. You will fill the array with one 1 in the first row, two 2’s in the second row, etc. This should work for any integer that the user enters. (Just because I am starting on 1 does not mean row 0 was skipped.) You must: use a loop to create the array shape. You must: use nested for loops to fill the array and to print the values back to the screen. Your program should print as shown below. Example Output: How many lines would you like in your triangle? >>>9 1 2 2 3 3 3 4 4 4 4 5 5 5 5 5 6 6 6 6 6 6 7 7 7 7 7 7 7 8 8 8 8 8 8 8 8 9 9 9 9 9 9 9 9 9 If your code looks like the code below, it is not what I’m asking for. The code below is making a square multidimensional array, not a triangular one. The code below is just leaving certain spots empty so that it looks like a triangle. I will ask you…arrow_forward
- I NEED HELP PLEASE NO REGEX OR ANYTHING COMPLICATED PLEASE.. USE BASIC APPROACH Create a program in Python that reads data from Breakfast Menu (https://www.w3schools.com/xml/simple.xml) and builds parallel arrays for the menu items, with each array containing the menu item name, description, calories, and price, respectively. After reading the data and building the arrays, display the menu items similar to the following: name - description - calories - priceAt the bottom, display the total number of items on the menu, the average number of calories per item, and the average price per item similar to: 0 items - 0 average calories - $0.00 average price You may either read the page using Internet processing methods, or you may download and save the page and then read the data from the saved file. You must process the data using string functions (no XML libraries). must use separate subroutines/functions/methods to implement each type of processing, and include error handling for…arrow_forwardCreate a program called array.py that has a function that takes four integer arguments. Those arguments should be put into an Numpy array. The function will have two print statements. The first will print the type of the array you create (which should be <class ‘numpy.ndarray’>). The second will print the multiplication of the four items in your array.arrow_forwardThe Lo Shu Magic Square is a grid with 3 rows and 3 columns shown below. The Lo Shu Magic Square has the following properties: The grid contains the numbers 1 – 9 exactly The sum of each row, each column and each diagonal all add up to the same number. This is shown below: Write a program that simulates a magic square using 3 one dimensional parallel arrays of integer type. Do not use two-dimensional array. Each one the arrays corresponds to a row of the magic square. The program asks the user to enter the values of the magic square row by row and informs the user if the grid is a magic square or not. Processing Requirements - c++ Use the following template to start your project: #include<iostream> using namespace std; // Global constants const int ROWS = 3; // The number of rows in the array const int COLS = 3; // The number of columns in the array const int MIN = 1; // The value of the smallest number const int MAX = 9; // The value of the largest number //…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
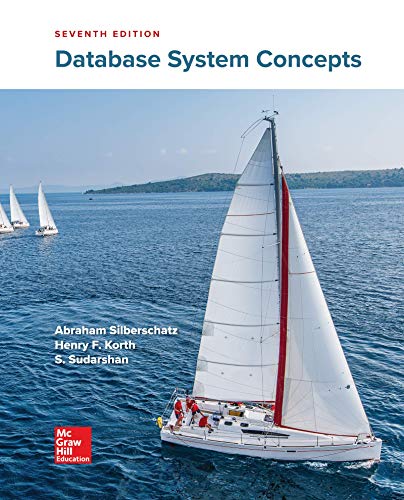
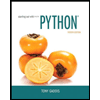
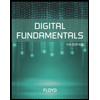
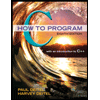
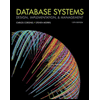
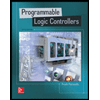