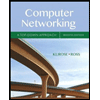
Need help in writing a program which is a java calculator. Use only procedural constructs of the Java programming language.
You may use all the following: - the Main class since, - Scanner class with all its methods for input and output, - Array and String classes with all their methods
Must do conversions:
- Binary to hexadecimal and vice versa
- Arithmetic to binary and vice versa
- Arithmetic to hexadecimal and vice versa
Please use IntelliJ idea or eclipse.
I have the code already done for:
- Binary to decimal
- Decimal to binary
- Hexadecimal to decimal
- Decimal to hexadecimal
Please use this and add it to your code to print out the calculator values:
public static void main(String[] args) {
//String binary = toBinaryString(number);
Scanner input = new Scanner(System.in);
System.out.println("Number Conversion in java \n");
// Display the menu
System.out.println("1.\t Decimal to Binary");
System.out.println("2.\t Decimal to Hexadecimal");
System.out.println("3.\t Binary to Decimal");
System.out.println("4.\t Hexadecimal to Decimal \n");
System.out.println("Your choice?");
//Get user's choice
int choice = -1;
while (choice < 0 || choice > 4) {
choice = input.nextInt();
switch (choice) {
case1:
System.out.println("\nEnter Decimal Number");
break;
case2:
System.out.println("\nEnter Decimal Number");
break;
case3:
System.out.println("\nEnter Binary");
break;
case4:
System.out.println("\nEnter Hexadecimal");
break;
default:
System.out.println("\nInvalid choice. Please choose a number between 1 and 4.");
break;
}
}
if (choice == 1) {
int number = input.nextInt();
String binary = toBinaryString(number);
binary = recursive(number);
System.out.printf("Decimal to Binary (%d) = %s", number, binary);
}
else if (choice == 2) {
int number2 = input.nextInt();
String hexadecimal = toHexString(number2);
hexadecimal = recursiveDecHex(number2);
System.out.printf("Decimal to Hexadecimal (%d) = %s ", number2, hexadecimal);
}
else if (choice == 3 ) {
String binary2 = input.next();
int decimal = toDecimalUsingParseInt(binary2);
decimal = recursiveBin(binary2);
System.out.printf("\n2. Binary to decimal - recursive(%s) = %d ", binary2, decimal);
} else {
String hex = input.next();
int decimal = toHexUsingParseInt(hex);
decimal = recursiveHexDec(hex);
System.out.printf("Hexadecimal to Decimal (%s) = %d ", hex, decimal);
}
input.close();
}
private static String toBinaryString(int number) {
return Integer.toBinaryString(number);
}
private static String toHexString(int number) {
return Integer.toHexString(number);
}
private static int toDecimalUsingParseInt(String binaryNumber) {
return Integer.parseInt(binaryNumber, 2);
}
private static int toHexUsingParseInt(String number) {
return Integer.parseInt(number, 16);
}
private static String recursive(int number) {
StringBuilder builder = new StringBuilder();
if (number > 0) {
String binaryNumber = recursive(number / 2);
int digit = number % 2;
builder.append(binaryNumber + digit);
}
return builder.toString();
}
private static String recursiveDecHex(int number) {
StringBuilder builder = new StringBuilder();
if (number > 0) {
String hexNumber = recursiveDecHex(number / 16);
String hexCode = "0123456789ABCDEF";
int hexDigit = number % 16;
builder.append(hexNumber + hexCode.charAt(hexDigit));
}
return builder.toString();
}
private static int recursiveBin(String binaryNumber) {
int decimal = 0;
int length = binaryNumber.length();
if (length > 0) {
String substring = binaryNumber.substring(1);
int digit = Character.getNumericValue(binaryNumber.charAt(0));
decimal = digit * (int) Math.pow(2, length - 1) + recursiveBin(substring);
}
return decimal;
}
private static int recursiveHexDec(String hexNumber) {
int decimal = 0;
String hexCode = "0123456789ABCDEF";
hexNumber = hexNumber.toUpperCase();
int length = hexNumber.length();
if (length > 0) {
char ch = hexNumber.charAt(0);
int digit = hexCode.indexOf(ch);
String substring = hexNumber.substring(1);
decimal = digit * (int) Math.pow(16, length - 1) + recursiveHexDec(substring);
}
return decimal;
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 4 images

- please quickly thanks ! use javaarrow_forwardMath 130 Java programming Dear Bartleby, I am a student beginning in computer science. May I have some assistance on this lab assignment please? Thank you.arrow_forwardInstructor note: This lab is part of the assignment for this chapter. This lab uses two Java files, LabProgram.java and SimpleCar.java. The SimpleCar class has been developed and provided to you already. You don't need to change anything in that class. Your job is to use the SimpleCar class to complete the specified tasks in the main() method of LabProgram.java Given two integers that represent the miles to drive forward and the miles to drive in reverse as user inputs, create a SimpleCar object that performs the following operations: Drives input number of miles forward Drives input number of miles in reverse Honks the horn Reports car status The SimpleCar class is found in the file SimpleCar.java. Ex: If the input is: 100 4 the output is: beep beep Car has driven: 96 milesarrow_forward
- Do not attempt, if you are using other's work. I'll give multiple dislikes for plagiarism and also will report your account. Give unique answer others are wrong. Question: Write a Java program that takes user input for two numbers, multiplies them, and prints the result. Ensure that your program is user-friendly, handling different data types, and providing meaningful output. Submission Guidelines: Use appropriate variable types for user input and the result. Implement input validation to handle unexpected user inputs. Print a clear and informative message along with the result.arrow_forwardQ1. Write two Java classes for calculating the power m" using the recursive method as follow: Class A A. Define two private variables in the class: names n and m. B. Define a constructor takes two parameters m and n and assign them to the class variables. C. Define setter and getter methods. D. Define a recursive method which take two parameters n, m and calculates the power using recursion. Class B: A. Define the main method. B. Define an object of class A and call its constructor which takes two parameters C. Call all the defined methods in Class A. Send the values 4,5 when call the recursion method. Attach File Browse My Computerarrow_forwardFor the code in java below it shows a deck of 52 cards and asks the name of the two players and makes both players draw five cards from the deck. What I want to be added onto the code is the possibility for Player A too chose whatever card they want from his/her 5 cards and Player B has to chose two cards from his/her 5 cards that equal in value of Player A's card. I also want the cards that have been used to be replaced by different cards in the deck of 52 cards and that game to go on until the deck runs out of cards. Main class code: import java.util.ArrayList; import java.util.Scanner; import java.util.List; import java.util.Random; class Main { publicstaticvoid main(String[] args) { // card game, two players, take turns. String[] suits = {"Hearts", "Clubs", "Spades", "Diamonds"}; String[] numbers = {"A", "2", "3", "4", "5", "6", "7", "8", "9", "10", "J", "Q", "K"}; for(String oneSuit : suits){ for(String num : numbers){ System.out.println(oneSuit + " " + num); } }…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
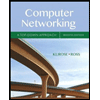
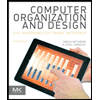
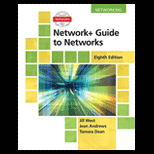
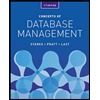
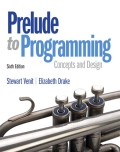
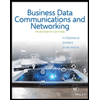