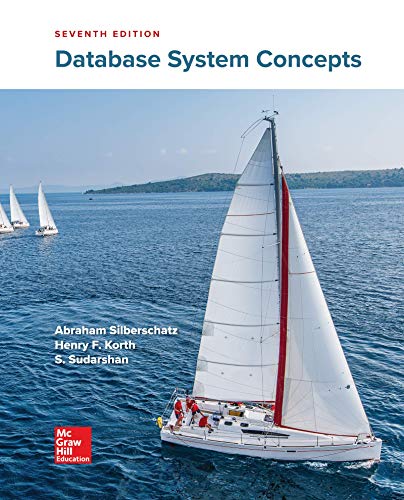
Need help finishing this Java code.
Here's what I need to do (Write a program to create a customer's bill for a company. The company sells only five different products: TV, VCR, Remote Controller, CD Player and Tape Recorder. The unit prices are $400.00, $220, $35.20, $300.00 and $150.00 respectively. The program must read the quantity of each piece of equipment purchased from the keyboard. It then, calculates the cost of each item, the subtotal and the total cost after an 8.25% sales tax. The input data consists of a set of integers representing the quantities of each item sold. These integers must be input into the program in a user- friendly way; that is, the program must prompt the user for each quantity as shown below -
How many TV's were sold? 3
How many VCR's were sold? 5
How many remote controller's were sold? 1
How many CD's were sold? 2
How many Tape Recorder's were sold? 4
The output of the program should print the following data properly formatted:
QTY DESCRIPTION UNIT PRICE TOTAL PRICE
...
SUBTOTAL
TAX
TOTAL
Define constants for the unit prices and the tax rate. Use integer variables to store the quantities for each item. Use floating-point variables to store the total price of each item, the bill subtotal, the tax amount and and the total amount of the bill.
Format the output adequately showing the 4 columns (QTY, DESCRIPTION, UNIT PRICE, TOTAL PRICE) in a single row. Use System.out.printf for output.).
This is my code:
public class Main {
public static void main(String[] args)
{
Scanner scanner = new Scanner
//Use Constants to find unit prices and the tax amount
final double TV_P = 400.00;
final double VCR_P = 220.00;
final double REMOTE_P = 35.20;
final double CD_P = 300.00;
final double TAPE_RECORDER_P = 100.00;
//Use constant to value the tax amount
final double TAX = 0.0825;
//Set the Variables for numbers needed for Inventory
int tvQ, vcrQ, remoteQ, cdQ, tapeQ;
double tvTotal, vcrTotal, remoteTotal, cdTotal, tapeTotal;
double Subtotal, Tax, Total;
// Create inputs for the different quantities
System.out.println("How many Remotes were sold");
remoteq = System.currentTimeMillis();
System.out.println("How many CDs were sold");
cdQ = System.currentTimeMillis();
System.out.println("How many Tape Recorders were sold?");
tapeQ = System.currentTimeMillis();
System.out.println("How many VCR's were sold?");
vcrQ = System.currentTimeMillis();
System.out.println("How many TV's were sold?");
tvQ = system.currentTimeMillis();
// Determine the Total for each item that is given in the quantity
cdTotal = cdQ * CD_P;
vcrTotal = vcrQ * VCR_P;
tvTotal = tvQ * TV_P;
remoteTotal = remoteQ * Remote_P;
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 1 images

- Program must be in Java Body Mass Index (BMI) is one of the criteria that is used to calculate whether one is fit or not basing on a person’s height (m) and weight (kg). The criterion is very simple; if one’s BMI is 25 or greater, it signifies overweight. A BMI less or equal to 18.5 indicates underweight. Lastly, a BMI greater than 30 shows obesity.You have been requested to create a program that that helps the Namibian Defence Forces’ (NDF) Captain to calculates the BMI of a person (BMI = weight/height2) and also determine whether the person has also completed running 10 km in the required time. Men should complete 10km in less than 60 minutes for them to be accepted. Women should do that under 80 minutes. Both males and females are accepted if their BMI is less than 25 and greater than 18.5. For 2018 intake, there are already 6 males selected and 1 female. The values for gender, mass and weight are enter by user inputsSample Run 1Welcome to NDF Captain's Selection…arrow_forwardUsing pythonarrow_forwardChapter 5. PC #2. Retail Price Calculator (page 312) Write a program that asks the user to enter an item’s wholesale cost and its markup percentage. It should then display the item’s retail price. For example: • If an item’s wholesale cost is 5.00 and its markup percentage is 100 percent, then the item’s retail price is 10.00. • If an item’s wholesale cost is 5.00 and its markup percentage is 50 percent, then the item’s retail price is 7.50. The program should have a method named calculateRetail that receives the wholesale cost and the markup percentage as arguments, and returns the retail price of the item. Class name: RetailPriceCalculator Here is a code: please modify this code so it works in Hypergrade when I submit it so it passes all the test casses in Hypergrade. It has to pass all the test casses in Hypergrade becausw when I submit the code in Hypercase it does not run and it says 0 out of 5 casses passed. Please fix this code. I do not need thanks for playing or…arrow_forward
- Python: numpy def serial_numbers(num_players):"""QUESTION 2- You are going to assign each player a serial number in the game.- In order to make the players feel that the game is very popular with a large player base,you don't want the serial numbers to be consecutive. - Instead, the serial numbers of the players must be equally spaced, starting from 1 and going all the way up to 100 (inclusive).- Given the number of players in the system, return a 1D numpy array of the serial numbers for the players.- THIS MUST BE DONE IN ONE LINEArgs:num_players (int)Returns:np.array>> serial_numbers(10)array([1. 12. 23. 34. 45. 56. 67. 78. 89. 100.])>> serial_numbers(12)array([1. 10. 19. 28. 37. 46. 55. 64. 73. 82. 91. 100.])""" # print(serial_numbers(10)) # print(serial_numbers(12))arrow_forwardUse C language to write your program. Show your code in a picture with commentsarrow_forwardFor Beginning Java: Part 1 Write a program to create a customer's bill for a company. The company sells only five different products: TV, VCR, Remote Controller, CD Player and Tape Recorder. The unit prices are $400.00, $220, $35.20, $300.00 and $150.00 respectively. The program must read the quantity of each piece of equipment purchased from the keyboard. It then, calculates the cost of each item, the subtotal and the total cost after an 8.25% sales tax. The input data consists of a set of integers representing the quantities of each item sold. These integers must be input into the program in a user- friendly way; that is, the program must prompt the user for each quantity as shown below - How many TV's were sold? 3 How many VCR's were sold? 5 How many remote controller's were sold? 1 How many CD's were sold? 2 How many Tape Recorder's were sold? 4 The output of the program should print the following data properly formatted: QTY DESCRIPTION UNIT PRICE TOTAL PRICE…arrow_forward
- n this lab, you complete a prewritten Python program for a carpenter who creates personalized house signs. The program is supposed to compute the price of any sign a customer orders, based on the following facts: The charge for all signs is a minimum of $35.00. The first five letters or numbers are included in the minimum charge; there is a $4 charge for each additional character. If the sign is make of oak, add $20.00. No charge is added for pine. Black or white characters are included in the minimum charge; there is an additional $15 charge for gold-leaf lettering.arrow_forwardA company wants to know the percentages of total sales and total expenses attributableto each salesperson. Each person has the following data: name – last name and firstname, sales, and expenses. Write a program that produces a report with a header linecontaining the total sales and total expenses. Following this heading should be a tablewith each salesperson’s name, percentage of total sales, and percentage of totalexpenses sorted by the salesperson’s name. Use a total of 25 records for this project.arrow_forwardA place to buy candy is from a candy machine. A new candy machine is bought for the gym, but it is not working properly. The candy machine has four dispensers to hold and release items sold by the candy machine and a cash register. The machine sells four products — candies, chips, gum, and cookies—each of which is stored in a separate dispenser. You have been asked to write a program for this candy machine so that it can be put into operation. The program should do the following: Show the customer the different products sold by the candy machine Let the customer make the selection Show the customer the cost of the item selected Accept money from the customer Return change Release the item, that is, make the sale Note: java programming, please used given outline code //Implement the blueprint of the Candy Machine import java.util.*; public class CandyMachine { // Each candy machine is made of 1 CashRegister and 4 Dispensers CashRegistercashRegister; Dispensercandy;…arrow_forward
- Help pleasearrow_forwardNeed python help running code and others. Functions are ideal for use in menu-driven programs. When the user selects an item from a menu, the program can call the appropriate function. Write a menu-driven program for Burgers. Display the food menu to a user (Just show the 5 options' names and prices - No need to show the details!) Use numbers for the options and the number "6" to exit. If the user enters "6", your code should not ask any other questions, just show a message like "Thank you, hope to see you again!" Ask the user what he/she wants and how many of them. (Check the user inputs) Keep asking the user until he/she chooses the exit option. Use a data structure to save the quantities of the orders. You should use ArrayLists/Array Bags, or LinkedLists/LinkedBags (one or more of them) to save data. Calculate the price. Ask the user whether he/she is a student or a staff. There is no tax for students and a 9% tax for staff. Add the tax price to the total price.…arrow_forwardChapter 5. PC #2. Retail Price Calculator (page 312) Write a program that asks the user to enter an item’s wholesale cost and its markup percentage. It should then display the item’s retail price. For example: • If an item’s wholesale cost is 5.00 and its markup percentage is 100 percent, then the item’s retail price is 10.00. • If an item’s wholesale cost is 5.00 and its markup percentage is 50 percent, then the item’s retail price is 7.50. The program should have a method named calculateRetail that receives the wholesale cost and the markup percentage as arguments, and returns the retail price of the item. Class name: RetailPriceCalculator Here is a working code but please fix it so it will in Hypergrade which as all the test casses. I DO NOT NEED THANK YOU IN THE PROGRAM. IT HAS TO PASS ALL THE TEST CASSES PLEASE. THANK YOU!!!!!: import java.util.*;class RetailPriceCalculator{ // creating a method public static double calculateRetail(double wholesale,double…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
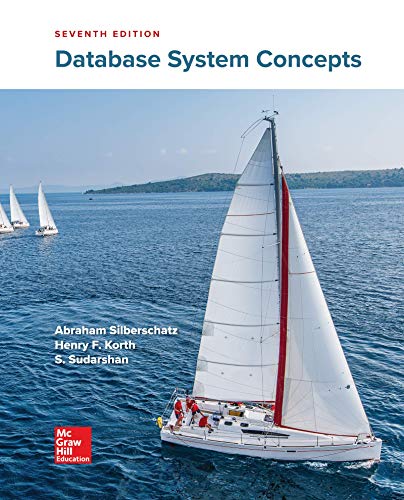
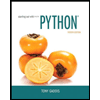
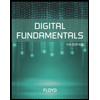
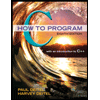
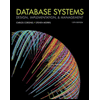
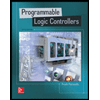