n someone help me with this problem in C++ In this exercise, you will work with 3 classes: Shape, Triangle and Rectangle. The first class is the class Shape. The Shape class has two float type properties: center_x and center_y. The Shape class also has the following methods: a constructor Shape(), that will set center_x and center_y to zero. set/get functions for the two attributes 1) You need to implement two additional functions for Shape: setCenter(float x, float y), that wi
can someone help me with this problem in C++
In this exercise, you will work with 3 classes: Shape, Triangle and Rectangle.
The first class is the class Shape. The Shape class has two float type properties: center_x and center_y. The Shape class also has the following methods:
- a constructor Shape(), that will set center_x and center_y to zero.
- set/get functions for the two attributes
1) You need to implement two additional functions for Shape:
- setCenter(float x, float y), that will set the new center and print:
Figure moved to [<center_x>, <center_y>]
- draw(), that will print:
Drawing Figure at [<center_x>, <center_y>]
2) You will have to implement another class, called Triangle, which inherits from the Shape class. The Triangle class has one int attribute: side. The Triangle class has the following methods:
- a constructor that will receive one int parameters (side)
- set/get for its attribute
- setCenter(float x, float y), that will set the new center and print:
Triangle moved to [<center_x>, <center_y>]
- draw(), that will print the following message and draw the triangle depending on the size of the side:
Drawing Triangle at [<center_x>, <center_y>] * ** *** //example of side = 3
3) You will have to implement another class, called Rectangle, which inherits from the Shape class. The Rectangle class has two int attributes: base and height. The Rectangle class has the following methods:
- a constructor that will receive two int parameters (base, height)
- set/get for its attributes
- setCenter(float x, float y), that will set the new center and print:
Rectangle moved to [<center_x>, <center_y>]
- draw(), that will print the following message and draw the triangle depending on the size of the base and height:
Drawing Rectangle at [<center_x>, <center_y>] **** **** **** //example of base = 4 and height = 3
Use polymorphism to make sure each object is drawn correctly!
here is the code I have so far
main.cpp
#include <iostream>
#include "Shape.h"
#include "Rectangle.h"
#include "Triangle.h"
using namespace std;
//Do not edit main.cpp
void move(Shape* fig, float x, float y);
int main() {
Shape* figure = new Shape();
Rectangle* rectangle = new Rectangle(6, 5);
Triangle* triangle = new Triangle(3);
move(figure, 6, 2);
cout << endl;
move(rectangle, 4, 6);
cout << endl;
move(triangle, 2, 9);
return 0;
}
void move(Shape* fig, float x, float y){
fig->setCenter(x, y);
fig->draw();
}
Triangle.CPP
#include <iostream>
#include "Triangle.h"
using namespace std;
//Constructor
//...
void Triangle::setSide(int s){
//...
}
int Triangle::getSide(){
//...
}
/*
setCenter(float x, float y)
draw()
*/
shape.h
#ifndef SHAPE_H
#define SHAPE_H
using namespace std;
class Shape{
public:
Shape();
void setX(float x);
void setY(float y);
float getX();
float getY();
//setCenter(float x, float y);
//draw();
private:
float center_x;
float center_y;
};
#endif
shape.cpp
#include <iostream>
#include "Shape.h"
using namespace std;
Shape::Shape(){
center_x = 0;
center_y = 0;
}
void Shape::setX(float x){
center_x = x;
}
void Shape::setY(float y){
center_y = y;
}
float Shape::getX(){
return center_x;
}
float Shape::getY(){
return center_y;
}
/*
setCenter(float x, float y)
draw()
*/
triangle.h
#ifndef TRIANGLE_H
#define TRIANGLE_H
#include "Shape.h"
using namespace std;
class Triangle: public Shape{
public:
//...
private:
//...
};
#endif
rectangle.h
#ifndef RECTANGLE_H
#define RECTANGLE_H
#include "Shape.h"
using namespace std;
class Rectangle: public Shape{
public:
//...
private:
//...
};
#endif
rectangle.cpp
#include <iostream>
#include "Rectangle.h"
using namespace std;
//Constructor
//...
void Rectangle::setBase(int b){
//...
}
void Rectangle::setHeight(int h){
//...
}
int Rectangle::getBase(){
//...
}
int Rectangle::getHeight(){
//...
}
/*
setCenter(float x, float y)
draw()
*/

Trending now
This is a popular solution!
Step by step
Solved in 8 steps with 1 images

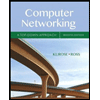
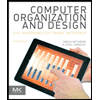
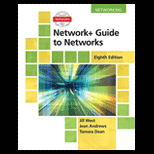
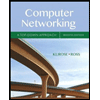
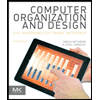
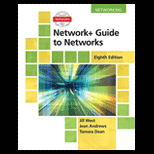
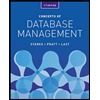
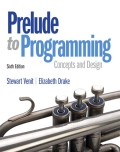
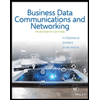