n C++ Design a program for a coffee shop. Make a menu driven program that allows the user to select items from a menu and add them to an order. Once the user is satisfied with their order, they can output a sales receipt. Display the receipt on the console and save the receipt in a txt file: qbc_sales_receipt.txt. . This coffee shop menu includes Coffee 1.85 Tea 1.05 Milk 1.25 Donut 2.25 Bagel 2.75 After each item is added to order, display a message for the user indicating the item name and total price (so 2 items at 2.50 each display 5.00) The program ends when the user prints their sales receipt The sales receipt should contain: -Line item for grand total -Line item for sales tax which is 8% -Line items with quantity, item name, and item price total If nothing is ordered, display “No receipt required. Thanks!” Modular Functions Requirement: main, displayMenu, displaySalesReceipt Input validation required The final display should read something like this Would you like to place an order? (y/n): y ITEM Price ******************** 1. Coffee 1.85 2. Tea 1.05 3. Milk 1.25 4. Donut 2.25 5. Bagel 2.75 ******************** Please make a selection: 2 How many Tea would you like: 4 4 of Tea added to order for 4.2 Would you like to continue ordering? (y/n): y ITEM Price ******************** 1. Coffee 1.85 2. Tea 1.05 3. Milk 1.25 4. Donut 2.25 5. Bagel 2.75 ******************** Please make a selection: 1 How many Coffee would you like: 3 3 of Coffee added to order for 5.55 Would you like to continue ordering? (y/n): n SALES RECEIPT ******************** 4 Tea $4.2 3 Coffee $5.55 ******************** Subtotal: $9.75 Tax Total: $0.78 ******************** Total: $10.53
In C++
Design a program for a coffee shop. Make a menu driven program that allows the user to select items from a menu and add them to an order. Once the user is satisfied with their order, they can output a sales receipt. Display the receipt on the console and save the receipt in a txt file: qbc_sales_receipt.txt. . This coffee shop menu includes
Coffee 1.85
Tea 1.05
Milk 1.25
Donut 2.25
Bagel 2.75
After each item is added to order, display a message for the user indicating the item name and total price (so 2 items at 2.50 each display 5.00)
The program ends when the user prints their sales receipt
The sales receipt should contain:
-Line item for grand total
-Line item for sales tax which is 8%
-Line items with quantity, item name, and item price total
If nothing is ordered, display “No receipt required. Thanks!”
Modular Functions Requirement: main, displayMenu, displaySalesReceipt
Input validation required
The final display should read something like this
Would you like to place an order? (y/n): y
ITEM Price
********************
1. Coffee 1.85
2. Tea 1.05
3. Milk 1.25
4. Donut 2.25
5. Bagel 2.75
********************
Please make a selection: 2
How many Tea would you like: 4
4 of Tea added to order for 4.2
Would you like to continue ordering? (y/n): y
ITEM Price
********************
1. Coffee 1.85
2. Tea 1.05
3. Milk 1.25
4. Donut 2.25
5. Bagel 2.75
********************
Please make a selection: 1
How many Coffee would you like: 3
3 of Coffee added to order for 5.55
Would you like to continue ordering? (y/n): n
SALES RECEIPT
********************
4 Tea $4.2
3 Coffee $5.55
********************
Subtotal: $9.75
Tax Total: $0.78
********************
Total: $10.53

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

How to turn this code that it accepts string input for the choice of drink instead of using int?
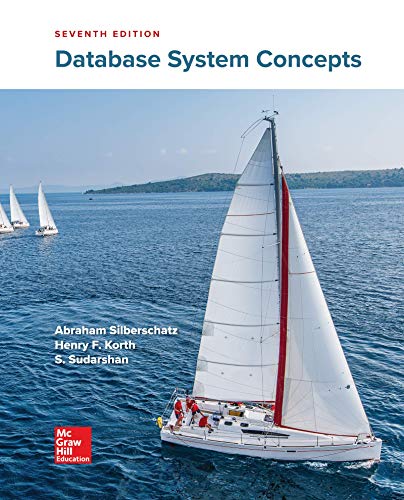
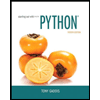
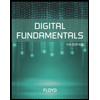
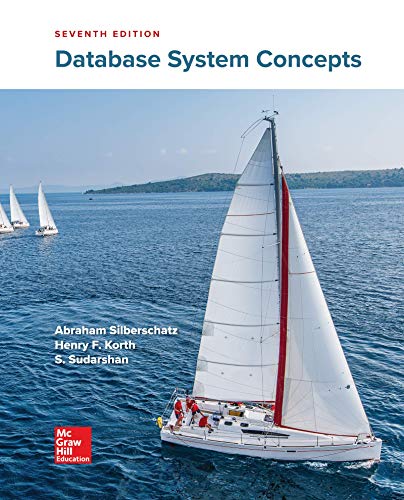
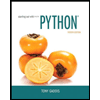
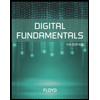
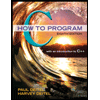
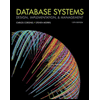
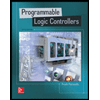