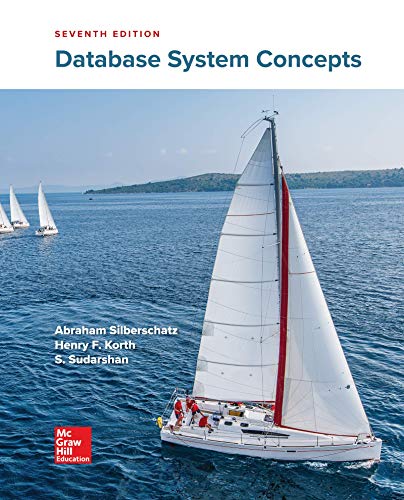
import java.io.File;
import java.io.FileNotFoundException;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner keyboard = new Scanner(System.in);
String fileName;
System.out.println("Please enter the file name or type QUIT to exit:");
fileName = keyboard.nextLine().trim();
while (!fileName.equalsIgnoreCase("QUIT")) {
File file = new File(fileName);
if (file.exists()) {
int wordCount = countWords(file);
System.out.println("Total number of words: " + wordCount + "\n");
break; // Exit after successful word count
} else {
System.out.println("File: " + fileName + " does not exist.");
System.out.println("Please enter the file name again or type QUIT to exit:");
fileName = keyboard.nextLine().trim();
}
}
keyboard.close();
}
public static int countWords(File file) {
int wordCount = 0;
try {
Scanner inputFile = new Scanner(file);
while (inputFile.hasNext()) {
// Remove leading and trailing whitespaces
String line = inputFile.nextLine().trim();
if(!line.isEmpty()) {
String[] words = line.split("\\s+");
wordCount += words.length;
}
}
inputFile.close();
} catch (FileNotFoundException e) {
// File existence already validated
// catch exception for completeness
e.printStackTrace();
}
return wordCount;
}
}
input1.txt
this is a test
input2.txt
this
is
a
test
input3.txt
Empty
input4txt
this this
is is
a a
test test
Test Case 1
input1.txtENTER
Total number of words: 4\n
Test Case 2
input2.txtENTER
Total number of words: 4\n
Test Case 3
input3.txtENTER
Total number of words: 0\n
Test Case 4
input4.txtENTER
Total number of words: 8\n
Test Case 5
input5.txtENTER
File: input5.txt does not exist.\n
Please enter the file name again or type QUIT to exit:\n
input1.txtENTER
Total number of words: 4\n
Test Case 6
qUitENTER
Test Case 7
input5.txtENTER
File: input5.txt does not exist.\n
Please enter the file name again or type QUIT to exit:\n
quItENTER
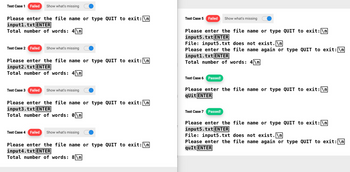
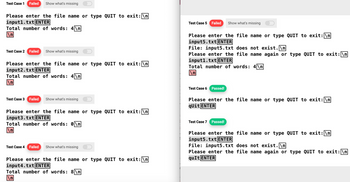

Step by stepSolved in 4 steps with 8 images

- >> classicVinyls.cpp For the following program, you will use the text file called “vinyls.txt” attached to this assignment. The file stores information about a collection of classic vinyls. The records in the file are like the ones on the following sample: Led_Zeppelin Led_Zeppelin 1969 1000.00 The_Prettiest_Star David_Bowie 1973 2000.00 Speedway Elvis_Presley 1968 5000.00 Spirit_in_the_Night Bruce_Springsteen 1973 5000.00 … Write a declaration for a structure named vinylRec that is to be used to store the records for the classic collection system. The fields in the record should include a title (string), an artist (string), the yearReleased (int), and an estimatedPrice(double). Create the following…arrow_forwardPython S3 Get File In the Python file, write a program to get all the files from a public S3 bucket named coderbytechallengesandbox. In there there might be multiple files, but your program should find the file with the prefix and cb - then output the full name of the file. You should use the boto3 module to solve this challenge. You do not need any access keys to access the bucket because it is public. This post might help you with how to access the bucket. Example Output ob name.txt Browse Resources Search for any help or documentation you might need for this problem. For exampler array indexing, Ruby hash tables, etc.arrow_forwardJava Program ASAP ************This program must work in hypergrade and pass all the test cases.********** Here is a working program. Please modidy this program so it passes the test cases down below. I have provided the correct test case as a screenshot. For Test Case 1 first print out Please enter the file name or type QUIT to exit:\ then you type text1.txtENTER and it displays Stop And Smell The Roses./n there needs to be nothing after that. For test case 2 first print out Please enter the file name or type QUIT to exit: then you type txt1.txtENTER then it reads out File 'txt1.txt' is not found.\n Then it didplays Please re-enter the file name or type QUIT to exit:\n after the test file is not found. then you type in text1.txt and it displays stop and smell the roses.\n. For test case 3 first print out Please enter the file name or type QUIT to exit: then you type text2.txtENTER and it displays A true rebel you are! Everyone was impressed. You'll do well to continue in…arrow_forward
- JAVA PROGRAM ASAP The program does not run in hypergrade can you please MODIFY THIS program ASAP BECAUSE it does not pass all the test cases when I upload it to hypergrade. I have provided the correct test cases as a screenshot as well as the failed test cases. It says 0 out of 4 passed. The program must pass the test case when uploaded to Hypergrade. Files data are down below: text1.txt StopAndSmellTheRoses. text2.txt ATrueRebelYouAre!EveryoneWasImpressed.You'llDoWellToContinueInTheSameSpirit.PleaseExplainABitMoreInTheWayOfFootnotes.FromTheGivenTextIt'sNotClearWhatAreWeReadingAbout. import java.io.BufferedReader;import java.io.FileReader;import java.io.IOException;import java.util.Scanner;class Main { // Driver code public static void main(String[] args) { // scanner object used to take user input Scanner sc = new Scanner(System.in); // loop iterates until user enters "quit" or "QUIT" while (true) { System.out.print("Please enter…arrow_forwardplease save number random and file and when print on monitor read from file and every num in single linearrow_forwardJAVA PROGRAM Chapter 4. Homework Assignment (read instructions carefully) Write a program that asks the user for the name of a file. The program should read all the numbers from the given file and display the total and average of all numbers in the following format (three decimal digits): Total: nnnnn.nnn Average: nnnnn.nnn Class name: FileTotalAndAverage double_input1.txt double_input2.txt PLEASE MODIFY THIS CODE, SO WHEN I UPLOAD IT TO HYPERGRADE IT PASSES ALL THE TEST CASES, BECAUSE WHEN I UPLOAD IT TO HYPERGRADE IT DOES NOT PASS THE TEST CASES. IT HAS TO PASS ALL THE TEST CASES. I PROVIDED THE CORRECT OUTPUT AS A SCREENSHOT AS A REFERRENCE. import java.io.BufferedReader;import java.io.FileReader;import java.io.IOException;import java.util.InputMismatchException;import java.util.Scanner;public class FileTotalAndAverage { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); String fileName; do {…arrow_forward
- Creates a sales receipt, displays the receipt entries and totals, and saves the receipt entries to a file .Prompt the user to enter the - Item Name Item Quantity Item Price Display the item name, the quantity, and item price, and the extended price (Item Quantity multiplied by Item Price) after the entry is made Save the item name, quantity, item price, and extended price to a file When you create the file, prompt the user for the name they want to give the file Separate the items saved with commas Each entry should be on a separate line in the text file Ask the user if they have more items to enter Once the user has finished entering items Close the file with the items entered Display the sales total If the sales total is more than $100 Calculate and display a 10% discount Calculate and display the sales tax using 8% as the sales tax rate The sales tax should be calculated on the sales total after the discount Display the total for the sales receiptarrow_forwardUsing a Counter-Controlled whileLoop Summary In this lab, you use a counter-controlled while loop in a Java program provided for you. When completed, the program should print the numbers 0 through 10, along with their values multiplied by 2 and by 10. The data file contains the necessary variable declarations and some output statements. Instructions Ensure the file named Multiply.java is open. Write a counter-controlled while loop that uses the loop control variable to take on the values 0 through 10. Remember to initialize the loop control variable before the program enters the loop. In the body of the loop, multiply the value of the loop control variable by 2 and by 10. Remember to change the value of the loop control variable in the body of the loop. Execute the program by clicking Run. Record the output of this program.arrow_forwardpythonarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
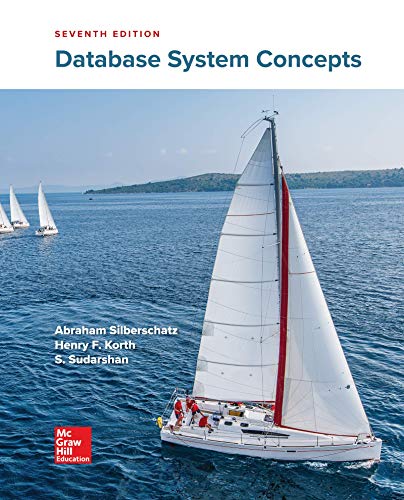
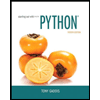
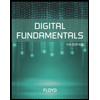
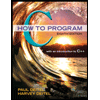
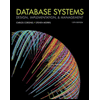
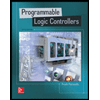