mystr is "numberlike" if: - mystr is not the empty string - the first character in mystr is a digit - the count of '.' in mystr is zero or one (can't have more than one decimal point in a number) - every character in mystr is a digit ('0', '1', '2', ..., '9') or a decimal point ('.') Write a simple python code in python to define the function is_numberlike(mystr), which takes a string parameter and returns a boolean result. If mystr is numberlike, the function returns True; otherwise, the function returns False. Hint: Check the first three requirements with decision statements, then use a for loop to iterate over mystr and check the every character is a digit or a dot
mystr is "numberlike" if:
- mystr is not the empty string
- the first character in mystr is a digit
- the count of '.' in mystr is zero or one (can't have more than one decimal point in a number)
- every character in mystr is a digit ('0', '1', '2', ..., '9') or a decimal point ('.')
Write a simple python code in python to define the function is_numberlike(mystr), which takes a string parameter and returns a boolean result.
If mystr is numberlike, the function returns True; otherwise, the function returns False.
Hint: Check the first three requirements with decision statements, then use a for loop to iterate over mystr and check the every character is a digit or a dot

The Python code is given below with code and output screenshot
Happy to help you ?
Step by step
Solved in 4 steps with 2 images

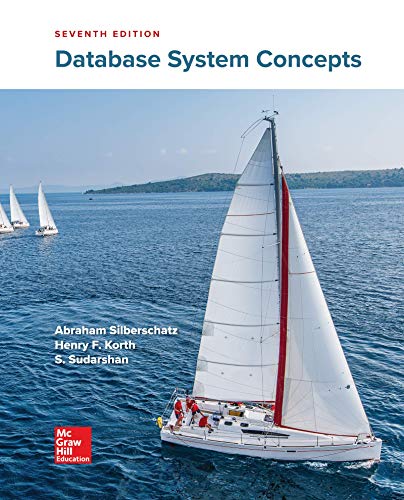
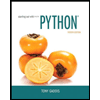
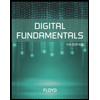
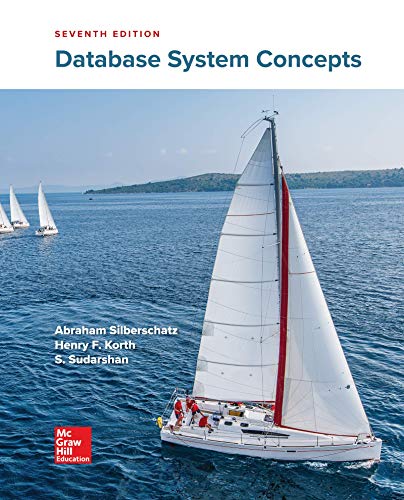
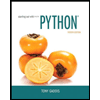
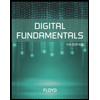
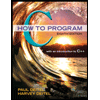
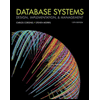
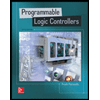