Modify the below program and add another public method called isEqual(). This method will determine if two fractions represent the same number (i.e. fraction), and return a Boolean result to indicate this. The method will take a Fraction class object as a parameter to compare with the ‘calling object’. We need to find a way to equate fractions represented as two integers. Modify your client class to test the Fraction class functionality that has been just added. import java.util.ArrayList; import java.util.Scanner; //Define the class Fraction class Fraction { private int n, d; public Fraction() { this.n = this.d = 0; //Initialize the values } public Fraction(int n, int d) { this.n = n; //Initialize the variables this.d = d; } //Define the getter function getNum() that returns the numerator public int getNum() { //Returns numerator return n; } //Define the getter function getDen() that returns the denominator public int getDen() { //Returns denominator return d; } //Define the boolean function isZero() that returns 0 if numerator is 0 and denominator is not equals to zero public boolean isZero() { return(getNum() == 0 && getDen() != 0); } //Define the function getSimplifiedFraction() that returns the simplified fraction public String getSimplifiedFraction() { //Decalre the string variable result to store the result String result = ""; //if the numerator and denominator both are zero if(getNum() == 0 && getDen() == 0) //result is zero result = "0"; //if the numerator is zero and denominator is non-zero else if(isZero()) //result is zero result = "0"; //if the numerator is non-zero and denominator is zero else if(getNum() != 0 && getDen() == 0) //result is Undefined result = "Undefined"; //for a defined and non-zero fraction else { //if the remainder is zero if(getNum() % getDen() == 0) result = (getNum() / getDen()) + ""; //if the numerator and denominator both are greater than zero else if(getNum() < 0 && getDen() < 0) result = (getNum() * -1) + "/" + (getDen() * -1); //if any of them is zero else if(getNum() < 0 || getDen() < 0) { //if the numerator is greater than zero if(getNum() < 0) result = "-" + (getNum() * -1) + "/" + getDen(); // appending a sign. //if the denominator is zero else result = "-" + getNum() + "/" + (getDen() * -1); // appending a sign. } //both are non-zero else result = (getNum() + "/" + getDen()); } //return the result return result; } //Define the method display() that displays the resultant fraction public void display() { //Call and display the fraction System.out.println(getSimplifiedFraction()); } } //Define the class TestFraction class Main { //Define the main method public static void main(String[] args) { //Declare the variables to store the numerator and denominator String res; int num, den; //Declare an ArrayList to store the results ArrayList fractions = new ArrayList<>(); //Create the object for scanner class Scanner sc = new Scanner(System.in); //use the do-while loop to iterate test the conditions do { //Prompts the user to enter the numerator System.out.print("Enter the num: "); //Store the Integer part only num = Integer.parseInt(sc.nextLine().trim()); //If the numerator is less than zero if(num < 0) //Break from loop break; //Prompts the user to enter the denominator System.out.print("Enter the den: "); //store the integer part only den = Integer.parseInt(sc.nextLine().trim()); //Create the object of Fraction class Fraction fraction = new Fraction(num, den); //Add the resultant fractions into the ArrayList fractions.add(fraction); res = fraction.getSimplifiedFraction(); System.out.println("\tFraction added to list as: " + res + "\n"); //Display the resultant fraction }while(res != "0"); //Display all fractions that are stored in the ArrayList System.out.println("\nDISPLAYING ALL FRACTIONS:\n" + "-------------------------"); //Use the loop to display the values stored in the ArrayList for(Fraction fr : fractions) fr.display(); //to print the new line System.out.println(); } }
Modify the below program and add another public method called isEqual(). This method will determine if two fractions represent the same number (i.e. fraction), and return a Boolean result to indicate this. The method will take a Fraction class object as a parameter to compare with the ‘calling object’. We need to find a way to equate fractions represented as two integers. Modify your client class to test the Fraction class functionality that has been just added.
import java.util.ArrayList;
import java.util.Scanner;
//Define the class Fraction
class Fraction
{
private int n, d;
public Fraction()
{
this.n = this.d = 0; //Initialize the values
}
public Fraction(int n, int d)
{
this.n = n; //Initialize the variables
this.d = d;
}
//Define the getter function getNum() that returns the numerator
public int getNum()
{
//Returns numerator
return n;
}
//Define the getter function getDen() that returns the denominator
public int getDen()
{
//Returns denominator
return d;
}
//Define the boolean function isZero() that returns 0 if numerator is 0 and denominator is not equals to zero
public boolean isZero()
{
return(getNum() == 0 && getDen() != 0);
}
//Define the function getSimplifiedFraction() that returns the simplified fraction
public String getSimplifiedFraction()
{
//Decalre the string variable result to store the result
String result = "";
//if the numerator and denominator both are zero
if(getNum() == 0 && getDen() == 0)
//result is zero
result = "0";
//if the numerator is zero and denominator is non-zero
else if(isZero())
//result is zero
result = "0";
//if the numerator is non-zero and denominator is zero
else if(getNum() != 0 && getDen() == 0)
//result is Undefined
result = "Undefined";
//for a defined and non-zero fraction
else
{
//if the remainder is zero
if(getNum() % getDen() == 0)
result = (getNum() / getDen()) + "";
//if the numerator and denominator both are greater than zero
else if(getNum() < 0 && getDen() < 0)
result = (getNum() * -1) + "/" + (getDen() * -1);
//if any of them is zero
else if(getNum() < 0 || getDen() < 0)
{
//if the numerator is greater than zero
if(getNum() < 0)
result = "-" + (getNum() * -1) + "/" + getDen(); // appending a sign.
//if the denominator is zero
else
result = "-" + getNum() + "/" + (getDen() * -1); // appending a sign.
}
//both are non-zero
else
result = (getNum() + "/" + getDen());
}
//return the result
return result;
}
//Define the method display() that displays the resultant fraction
public void display()
{
//Call and display the fraction
System.out.println(getSimplifiedFraction());
}
}
//Define the class TestFraction
class Main
{
//Define the main method
public static void main(String[] args)
{
//Declare the variables to store the numerator and denominator
String res;
int num, den;
//Declare an ArrayList to store the results
ArrayList<Fraction> fractions = new ArrayList<>();
//Create the object for scanner class
Scanner sc = new Scanner(System.in);
//use the do-while loop to iterate test the conditions
do
{
//Prompts the user to enter the numerator
System.out.print("Enter the num: ");
//Store the Integer part only
num = Integer.parseInt(sc.nextLine().trim());
//If the numerator is less than zero
if(num < 0)
//Break from loop
break;
//Prompts the user to enter the denominator
System.out.print("Enter the den: ");
//store the integer part only
den = Integer.parseInt(sc.nextLine().trim());
//Create the object of Fraction class
Fraction fraction = new Fraction(num, den);
//Add the resultant fractions into the ArrayList
fractions.add(fraction);
res = fraction.getSimplifiedFraction();
System.out.println("\tFraction added to list as: " + res + "\n"); //Display the resultant fraction
}while(res != "0");
//Display all fractions that are stored in the ArrayList
System.out.println("\nDISPLAYING ALL FRACTIONS:\n"
+ "-------------------------");
//Use the loop to display the values stored in the ArrayList
for(Fraction fr : fractions)
fr.display();
//to print the new line
System.out.println();
}
}

Step by step
Solved in 4 steps with 7 images

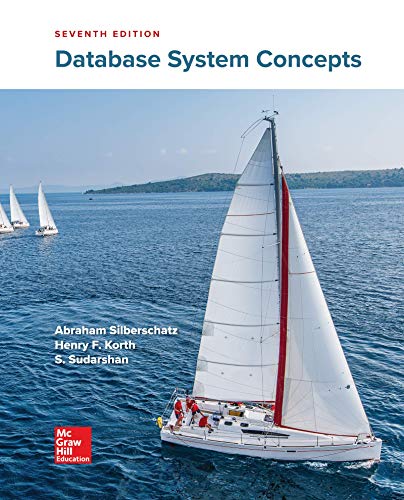
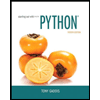
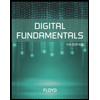
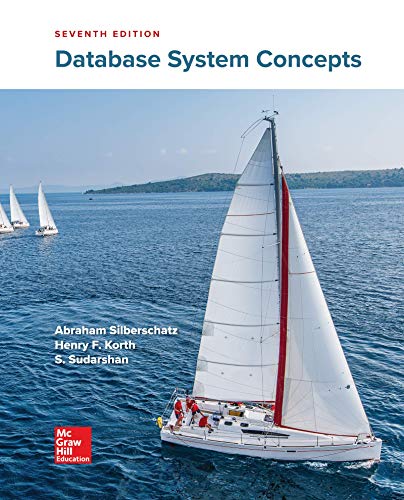
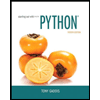
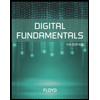
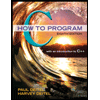
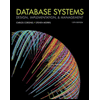
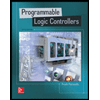