Modify C++ code. 1-if numberoftries used by user but not guess the correct answer print You can;t guess the correct random number and then print on second line" The correct random number is ------- "this. 2-Then ask from user if you want to play again or not if not then excute the program. Code: //included header file for basic input output stream #include //included header file for file stream #include //included header file to use rand() function #include //included header file to use time() function #include //included namespace using namespace std; //function to generate random number int generateRandom(); void tooLow(); void tooHigh(); void guessedCorrect(); void checkGuess(int guess, int randomNumber); void guessGame(); int generateRandom() { //use srand() function to change the random number after each execution srand((unsigned int) time(0)); //generated a random number between 1-50 through rand() function int randomNumber = ((rand() % 50)+1); //return random number to main() return randomNumber; } //function tooLow() void tooLow() { //print statement to print the message cout << "You have guessed too low." << endl; } //function tooHigh() void tooHigh() { //print statement to print the message cout << "You have guessed too high." << endl; } //function guessedCorrect() void guessedCorrect() { char choice; //print statement to print the message cout << "You have guessed correct." << endl; cout << "\nDo you want to play again (Y or N): " << endl; cin >> choice; switch (choice) { case 'y': guessGame(); break; case 'n': exit(0); } cout << endl; } //function to check guess void checkGuess(int guess, int randomNumber) { //If the users guesses below the number if (guess < randomNumber) //call a function tooLow() tooLow(); //If they guess over that number else if(guess > randomNumber) //call a function tooHigh() tooHigh(); //else statement else //call a function guessedCorrect() guessedCorrect(); } //function to start game void guessGame() { //welcome message cout << "------------Welcome to the game------------" << endl; //variable declaration int guess, level, trial = 0, numberOfTries; cout << "Enter level of game: " << endl; cout << "Press 1 for easy level" << endl; cout << "Press 2 for medium level" << endl; cout << "Press 3 for hard level" << endl; //input level cin >> level; //switch level switch (level) { //case 1 case 1: //assign numberOfTries to 8 numberOfTries = 8; //break break; //case 2 case 2: //assign numberOfTries to 5 numberOfTries = 5; //break break; //case 3 case 3: //assign numberOfTries to 2 numberOfTries = 2; //break break; //default case default: //display message "Wrong choice entered." cout << "Wrong choice entered." << endl; } //while loop run until numberOfTries //calling generateRandom int randomNumber = generateRandom(); while (trial < numberOfTries) { cout << "Enter your guess: "; //input number from user cin >> guess; //calling checkGuess() checkGuess(guess, randomNumber); //increment trial trial++; } } //main function int main() { int randomNumber; //declared variable guess int pin, filePin; int i = 0; //created file ifstream file; //opening file file.open("gamePin.txt"); //input pin from user while (i < 3) { cout << "Enter pin: "; cin >> pin; //extract pin from file file >> filePin; //compare user pin and pin in file if (pin == filePin) { //calling guessGame() guessGame(); break; } //else statement else { //display message "Wrong pin" cout << "Wrong pin" << endl; i++; } } return 0; }
Modify C++ code. 1-if numberoftries used by user but not guess the correct answer print You can;t guess the correct random number and then print on second line" The correct random number is ------- "this. 2-Then ask from user if you want to play again or not if not then excute the program. Code: //included header file for basic input output stream #include //included header file for file stream #include //included header file to use rand() function #include //included header file to use time() function #include //included namespace using namespace std; //function to generate random number int generateRandom(); void tooLow(); void tooHigh(); void guessedCorrect(); void checkGuess(int guess, int randomNumber); void guessGame(); int generateRandom() { //use srand() function to change the random number after each execution srand((unsigned int) time(0)); //generated a random number between 1-50 through rand() function int randomNumber = ((rand() % 50)+1); //return random number to main() return randomNumber; } //function tooLow() void tooLow() { //print statement to print the message cout << "You have guessed too low." << endl; } //function tooHigh() void tooHigh() { //print statement to print the message cout << "You have guessed too high." << endl; } //function guessedCorrect() void guessedCorrect() { char choice; //print statement to print the message cout << "You have guessed correct." << endl; cout << "\nDo you want to play again (Y or N): " << endl; cin >> choice; switch (choice) { case 'y': guessGame(); break; case 'n': exit(0); } cout << endl; } //function to check guess void checkGuess(int guess, int randomNumber) { //If the users guesses below the number if (guess < randomNumber) //call a function tooLow() tooLow(); //If they guess over that number else if(guess > randomNumber) //call a function tooHigh() tooHigh(); //else statement else //call a function guessedCorrect() guessedCorrect(); } //function to start game void guessGame() { //welcome message cout << "------------Welcome to the game------------" << endl; //variable declaration int guess, level, trial = 0, numberOfTries; cout << "Enter level of game: " << endl; cout << "Press 1 for easy level" << endl; cout << "Press 2 for medium level" << endl; cout << "Press 3 for hard level" << endl; //input level cin >> level; //switch level switch (level) { //case 1 case 1: //assign numberOfTries to 8 numberOfTries = 8; //break break; //case 2 case 2: //assign numberOfTries to 5 numberOfTries = 5; //break break; //case 3 case 3: //assign numberOfTries to 2 numberOfTries = 2; //break break; //default case default: //display message "Wrong choice entered." cout << "Wrong choice entered." << endl; } //while loop run until numberOfTries //calling generateRandom int randomNumber = generateRandom(); while (trial < numberOfTries) { cout << "Enter your guess: "; //input number from user cin >> guess; //calling checkGuess() checkGuess(guess, randomNumber); //increment trial trial++; } } //main function int main() { int randomNumber; //declared variable guess int pin, filePin; int i = 0; //created file ifstream file; //opening file file.open("gamePin.txt"); //input pin from user while (i < 3) { cout << "Enter pin: "; cin >> pin; //extract pin from file file >> filePin; //compare user pin and pin in file if (pin == filePin) { //calling guessGame() guessGame(); break; } //else statement else { //display message "Wrong pin" cout << "Wrong pin" << endl; i++; } } return 0; }
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter15: Recursion
Section: Chapter Questions
Problem 20PE
Related questions
Question
Modify C++ code.
1-if numberoftries used by user but not guess the correct answer print You can;t guess the correct random number and then print on second line" The correct random number is ------- "this.
2-Then ask from user if you want to play again or not if not then excute the program.
Code:
//included header file for basic input output stream
#include <iostream>
//included header file for file stream
#include <fstream>
//included header file to use rand() function
#include <cstdlib>
//included header file to use time() function
#include <ctime>
//included namespace
using namespace std;
//function to generate random number
int generateRandom();
void tooLow();
void tooHigh();
void guessedCorrect();
void checkGuess(int guess, int randomNumber);
void guessGame();
int generateRandom()
{
//use srand() function to change the random number after each execution
srand((unsigned int) time(0));
//generated a random number between 1-50 through rand() function
int randomNumber = ((rand() % 50)+1);
//return random number to main()
return randomNumber;
}
//function tooLow()
void tooLow()
{
//print statement to print the message
cout << "You have guessed too low." << endl;
}
//function tooHigh()
void tooHigh()
{
//print statement to print the message
cout << "You have guessed too high." << endl;
}
//function guessedCorrect()
void guessedCorrect()
{
char choice;
//print statement to print the message
cout << "You have guessed correct." << endl;
cout << "\nDo you want to play again (Y or N): " << endl;
cin >> choice;
switch (choice)
{
case 'y':
guessGame();
break;
case 'n':
exit(0);
}
cout << endl;
}
//function to check guess
void checkGuess(int guess, int randomNumber)
{
//If the users guesses below the number
if (guess < randomNumber)
//call a function tooLow()
tooLow();
//If they guess over that number
else if(guess > randomNumber)
//call a function tooHigh()
tooHigh();
//else statement
else
//call a function guessedCorrect()
guessedCorrect();
}
//function to start game
void guessGame()
{
//welcome message
cout << "------------Welcome to the game------------" << endl;
//variable declaration
int guess, level, trial = 0, numberOfTries;
cout << "Enter level of game: " << endl;
cout << "Press 1 for easy level" << endl;
cout << "Press 2 for medium level" << endl;
cout << "Press 3 for hard level" << endl;
//input level
cin >> level;
//switch level
switch (level)
{
//case 1
case 1:
//assign numberOfTries to 8
numberOfTries = 8;
//break
break;
//case 2
case 2:
//assign numberOfTries to 5
numberOfTries = 5;
//break
break;
//case 3
case 3:
//assign numberOfTries to 2
numberOfTries = 2;
//break
break;
//default case
default:
//display message "Wrong choice entered."
cout << "Wrong choice entered." << endl;
}
//while loop run until numberOfTries
//calling generateRandom
int randomNumber = generateRandom();
while (trial < numberOfTries)
{
cout << "Enter your guess: ";
//input number from user
cin >> guess;
//calling checkGuess()
checkGuess(guess, randomNumber);
//increment trial
trial++;
}
}
//main function
int main()
{
int randomNumber;
//declared variable guess
int pin, filePin;
int i = 0;
//created file
ifstream file;
//opening file
file.open("gamePin.txt");
//input pin from user
while (i < 3)
{
cout << "Enter pin: ";
cin >> pin;
//extract pin from file
file >> filePin;
//compare user pin and pin in file
if (pin == filePin)
{
//calling guessGame()
guessGame();
break;
}
//else statement
else
{
//display message "Wrong pin"
cout << "Wrong pin" << endl;
i++;
}
}
return 0;
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
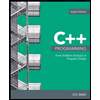
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
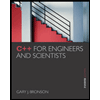
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
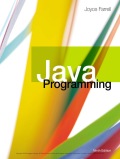
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
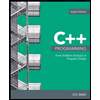
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
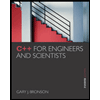
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
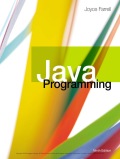
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT