Miles to track laps One lap around a standard high-school running track is exactly 0.25 miles. Write a program that takes a number of miles as input, and outputs the number of laps. Output each floating-point value with two digits after the decimal point, which can be achieved as follows: print('%0.2f' % your_value) Ex: If the input is: 1.5 the output is: 6.00 Ex: If the input is: 2.2 the output is: 8.80 Your program must define and call the following function: def miles_to_laps(user_miles) Define the function miles_to_laps taking the variable user_miles Return the value of dividing userMiles by 0.25 In main Declare variable user_miles as a double Input user_miles Assign to num_laps the value returned from miles_to_laps passing in user_miles Output num_laps My code to point: def miles_to_laps(user_miles): laps = user_miles * 4 return(laps) user_miles = float laps = float(miles_to_laps(user_miles) if __name__ == '__main__':
Miles to track laps One lap around a standard high-school running track is exactly 0.25 miles. Write a program that takes a number of miles as input, and outputs the number of laps. Output each floating-point value with two digits after the decimal point, which can be achieved as follows: print('%0.2f' % your_value) Ex: If the input is: 1.5 the output is: 6.00 Ex: If the input is: 2.2 the output is: 8.80 Your program must define and call the following function: def miles_to_laps(user_miles)
Define the function miles_to_laps taking the variable user_miles
Return the value of dividing userMiles by 0.25
In main
Declare variable user_miles as a double
Input user_miles
Assign to num_laps the value returned from miles_to_laps passing in user_miles
Output num_laps
My code to point:
def miles_to_laps(user_miles):
laps = user_miles * 4
return(laps)
user_miles = float
laps = float(miles_to_laps(user_miles)
if __name__ == '__main__':

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

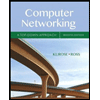
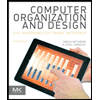
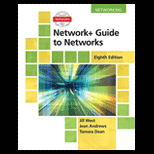
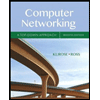
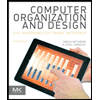
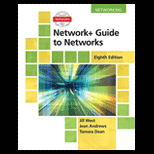
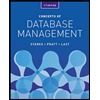
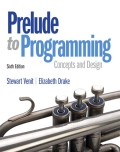
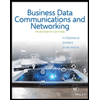