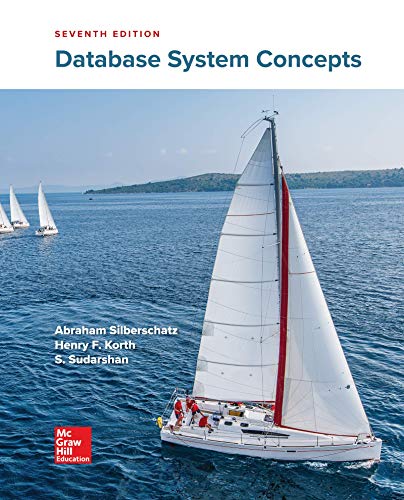
Magic 8 Ball Lab
Introduction For this lab, you are going to build a simple magic eight-ball, so you no longer have to make any choices. On that note, if you haven’t, you should watch Interstate 60. All joking aside, you will build a 15 sided magic 8-ball, just for simplicity (an actual one has 20 sides for choices). If you are curious about all the choices, you can read up more on them here. Also, if you need a refresher on if statements, go here. Step 1 - Reading the code First take a look at the provided code. You should change the comments to include your name at the top of the file. Furthermore, you will notice all the functions are ‘stubbed’ out for you. This means we have provided the function signature, and a dummy return value that you will replace completely. We have also provided one function in its entirety, so you can use it as a sample/template to look at.
def get_positive_answer(answer):
ans = "You may rely on it."
if answer == 0:
ans = "As I see it, yes."
elif answer == 1:
ans = "Signs point to yes."
elif answer == 2:
ans ="Outlook good."
elif answer == 3:
ans = "Without a doubt."
return ans
There are also some lines under that method commented out. You should uncomment them and see what happens. In all languages it is good to test frequently, and often, and this is exactly what we are doing here. We have provided sample tests throughout the code that you should feel free to use, and add others.
This program requires input, so in the Enter program input (optional) box enter the word yes or no. For now, we suggest just putting no (or n) in the box, until you have completed the lab.
Step 2 - get_negative_answer(answer)
get_negative_answer is just like get_positive_answer however the tone of the answer is negative instead of positive. We completed get_positive_answer, but you need to complete get_negative_answer. Right now, it is stubbed out with
You will have five options that can be assigned to ans, using if/elif statements. You can follow the model above provided in get_positive_answer, or come up with your own. In either case, the following options need to be returned based on the ‘number’ of answer. Make sure to match spacing and punctuation exactly!
- 0 - Don’t count on it.
- 1 - My reply is no.
- 2 - My sources say no.
- 3 - Outlook not so good.
- 4 (or higher) - Very doubtful.
Step 3 - get_no_answer(answer)
Similar to Step 2, build the if-statement for get_no_answer(answer) using the options below.
- 0 - Reply hazy, try again.
- 1 - Ask again later.
- 2 - Better not tell you now.
- 3 - Cannot predict now.
- 4 (or higher) - Concentrate and ask again.
Step 4 - get_answer(category, answer)
You have worked on three functions (two really) that take in a single parameter to return an answer. Each of those functions are specific to a category. This function determines which of the three functions to call.
- If the category is less than 24, call and store the returned value of get_negative_answer(answer) passing in answer as the parameter.
- If the category is between 24 and 73 (including 73), call and store the returned value of get_no_answer(answer) passing in answer as the parameter.
- If the category is greater than or equal to 74, call and store the returned value of get_positive_answer(answer) passing in answer as the parameter.
You will then return the answer provided. (there may be different orders you can make the checks in)
def a_func_with_if(val1, val2): ans = None if val1 < 0: ans = out_of_range(val2) elif val1 > 100: ans = out_of_range(val2) else: ans = "In Range!" return ans def out_of_range(val2): return "Not really doing anything with {}".format(val2)
Step by stepSolved in 2 steps

- Create the design for determining if a point is inside, on, or outside of the rectangle. Drawing out the rectangle and labeling the coordinates of all points will help you to visualize the problem. Once the design has been completed, write a program that prompts the user for the x and y coordinates for the lower left hand corner of a rectangle as well as the height and the width of the rectangle. After that, you will need to get the coordinates for the point from the user as well. Once all inputs have been entered by the user, determine if the point is inside the rectangle, on the rectangle, or outside the rectangle. If the point is inside the rectangle, print "inside the rectangle". If the point is on the rectangle, print "on the rectangle". If the point is outside of the rectangle, print "outside the rectangle". Example output: Enter the x coordinate of the lower left hand corner of the rectangle: 1 Enter the y coordinate of the lower left hand corner of the rectangle: 1 Enter the…arrow_forwardIt is given 12 balls that are all the same weight except for one that is heavier or lighter. Additionally, it is provided with a two-pan balance. In each scenario, the balance is used. You may place any number of the 12 balls on the left pan and the same number on the right pan and press a button to begin weighing; three alternative results exist: the weights are equal, the balls on the left are heavier, or the balls on the left are lighter. Develop a technique for determining which ball is the odd one out and whether it is heavier or lighter than the others using the fewest feasible uses of the balance. Solve the weighing issue for the situation of 39 balls, one of which is known to be strange.arrow_forwardMastermindIn this assignment, you will program the well-known game Mastermind. In Mastermind, player 1creates a pattern consisting of a pre-approved number of colored pins. Then player 2 has to guessthis pattern in as few turns as possible. Each turn, player 2 can guess what the pattern mightbe. Player 1 will then tell player 2 how many correct colors were guessed, and how many of thosecorrectly guessed colors were guessed in the right place. Player 2 can then use this information toimprove their guess for the next turn. When player 2 guesses the correct pattern, the game ends.Write a program that acts as player 1 of the Mastermind game. This program will generate a(random) pattern, and will play according to the game’s rules, with a human acting as player 2.The program should stop when the user guesses the pattern.Additionally, the program has to conform with the following specifications:1. Because of practical reasons, the pattern will consist of capital letters instead of colors.2.…arrow_forward
- 2) scrooge.py Scrooge McDuck is letting you into his vault full of pennies, nickels, dimes and quarters. He will let you play the following game: you can pick 8 coins at random, and if they sum to at least a dollar, you win, and can keep your money! But if you lose, you don't get to keep the money, AND have to pay Scrooge a dollar. (Each coin you pick is equally likely to be a penny, nickel, dime, or quarter.) Your job: using a simulation, estimate 1. the probability that you win (meaning your coins sum to more than a dollar), and 2. if you play this game over and over, the average amount you will win (where the amount you win in a game could be negative). Here is a bit more direction: you should simulate 100,000 plays of this game. Each simulated play should involve picking 8 different random numbers - each one should be .01, .05, .10, or .25, with each value equally likely - which represent the 8 coins being chosen; these should be summed each time. As you proceed through these…arrow_forward1. A Different Angle by CodeChum Admin It says here on the map that the secret treasure is behind this wall. The only writings on the wall are a bunch of numbers that don’t mean anything. There’s gotta be a way to get through this wall… hmmm.. What if we look at the numbers from a different angle? Quick, help me turn the numbers to 90 degrees counterclockwise! Input 1. Number of rows Constraints The value is guaranteed to be >= 3 and <= 100. Sample 3 2. Number of columns Constraints The value is guaranteed to be >= 3 and <= 100. Sample 3 3. Elements Description The elements of the multidimensional array depending on the number of rows and columns Constraints All elements are non-negative and are all less than 100. Sample 1·2·3 4·5·6 7·8·9 Output The first line will contain a message prompt to input the number of rows. The second line will contain a message prompt to input the number of columns. The succeeding lines will contain message…arrow_forward1. Simplify the following logic equation: F = ab'c + abcd' + ab'cd' + acd Also draw the circuit before and after simplifications, and comment on savings on number of gates. % savings (number of gates required before modification – number of gates required with simplest form)* 100/(number of gates required after modification)arrow_forward
- Blue-Eyed Island: A bunch of people are living on an island, when a visitor comes with a strangeorder: all blue-eyed people must leave the island as soon as possible. There will be a flight out at8:00pm every evening. Each person can see everyone else's eye color, but they do not know theirown (nor is anyone allowed to tell them). Additionally, they do not know how many people haveblue eyes, although they do know that at least one person does. How many days will it take theblue-eyed people to leave?arrow_forwardCounting the growth rings of a tree is a good way to tell the age of a tree. Each growth ring counts as one year. Use a Canvas widget to draw how the growth rings of a 5-year-old tree might look. Then, using the create_text method, number each growth ring starting from the center and working outward with the age in years associated with that ring.arrow_forwardActive buzzer project: Write an algorithm for the below code for all steps. Then write a conclusion (test&debug) for the code. Also, write comments for the code explaining each line.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
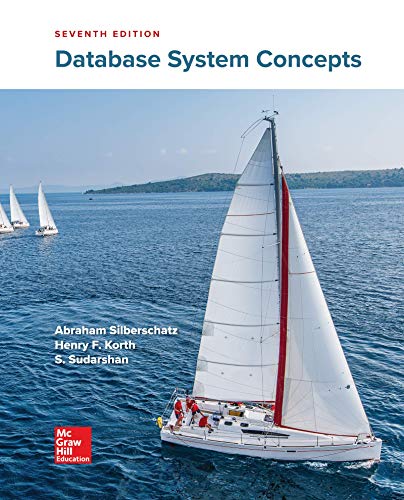
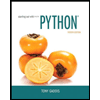
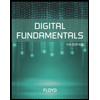
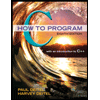
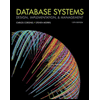
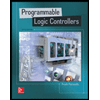