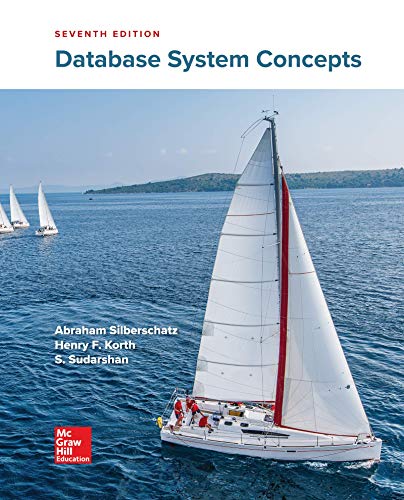
Please help me with this problem. Thank you
Testing Your MergeSorter:
I have given you a very limited set of JUnit tests to help determine if your implementation is correct. You need to add at least 5 more JUnit tests for the mergeSort method that test typical and edge cases. You are welcome to create more than five JUnit tests, but as long as you create and pass five JUnit tests
package divideandconquer;
import static org.junit.Assert.*;
import org.junit.Before;
import org.junit.BeforeClass;
import org.junit.Test;
public class MergeSorterTest {
@BeforeClass
public static void setUpBeforeClass() throws Exception {
}
@Before
public void setUp() throws Exception {
}
@Test
public void test() {
int[] nums = new int[]{4,3,2,1};
int[] sorted = new int[] {1,2,3,4};
MergeSorter.mergeSort(nums);
assertArrayEquals(sorted,nums);
}
}

To add at least 5 more JUnit tests for the mergeSort
method, you can test the following cases:
- An array with duplicated elements. This test will check if the implementation can handle arrays with duplicated elements and sort them correctly.
@Test
public void testDuplicatedElements() {
int[] nums = new int[]{4,3,3,2,1};
int[] sorted = new int[] {1,2,3,3,4};
MergeSorter.mergeSort(nums);
assertArrayEquals(sorted,nums);
}
Step by stepSolved in 3 steps

- Is there a consequence for adding a new key-value pair to an existing map entry?arrow_forwardYou will also have to write Student Tests that test the following in the provided file.i. Adding element(s).ii. Get elements at index – (1) with a valid index and (2) with an invalid indexiii. Test equality of two StringArrayLists (of size 0, 2)iv. Test if a StringArrayList contains a String (test both true and false cases)v.Test removing a String from a StringArrayList.arrow_forwardCreate more methods for ParseDoWhile, ParseDelete, and ParseReturn where all of the Node data structure correct, parses correctly, throws exceptions with good error messages. Make sure to write block of codes for these three methods.arrow_forward
- You will implement some methods in the UndirectedGraph class. The UndirectedGraph class contains two nested classes, Vertex and Node, you should NOT change these classes. The graph will store a list of all the vertices in the graph. Each vertex has a pointer to its adjacent vertices. To get started, import the starter file, Undirected.java into the graphs package you create in a new Java Project. Please do not change any of the method signatures in the class, but you can add any helper methods you deem necessary. Implement the methods described below. You are free to test your code however you prefer. Vertex Class (DO NOT EDIT)The vertex class holds information about the vertices in the graph. It has an int val, a Vertex next that refers to the next vertex in the list of vertices (not necessarily an adjacent vertex), and a Node edge that starts the list of adjacent vertices. Node Class (DO NOT EDIT)This is a simple class to represent an adjacency list of a vertex in the graph.…arrow_forwardWhen sorting objects using the Comparable interface, what happens if two objects have the same comparison value in the compareTo() method?arrow_forwardPlease Code in JAVA. Type the code out neatly. Add comments . (No need for long comments). Dont add any unnecesary code or comments. Do what the attached file says. And call it a day. Thanks !arrow_forward
- You should create a method and call it findTotalleaves (); this method will find all of the nodes that are considered to be leaves, which are nodes that do not have any BNode dependents. The public function must not take any arguments, but the private method must accept as a parameter a BNode that represents the current root.arrow_forwardComplete the docstring using the information attached (images):def upgrade_stations(threshold: int, num_bikes: int, stations: List["Station"]) -> int: """Modify each station in stations that has a capacity that is less than threshold by adding num_bikes to the capacity and bikes available counts. Modify each station at most once. Return the total number of bikes that were added to the bike share network. Precondition: num_bikes >= 0 >>> handout_copy = [HANDOUT_STATIONS[0][:], HANDOUT_STATIONS[1][:]] >>> upgrade_stations(25, 5, handout_copy) 5 >>> handout_copy[0] == HANDOUT_STATIONS[0] True >>> handout_copy[1] == [7001, 'Lower Jarvis St SMART / The Esplanade', \ 43.647992, -79.370907, 20, 10, 10] True """arrow_forwardPlease make sure to follow what is given!!!arrow_forward
- in my code there is a syntax error. i am trying to take out each character from inputted string and store each character into a linked list. i keep getting a syntax error stating that "non static method insertFirst(char) cannot be referenced in a static context" I have no idea why this is happening and i need help. I cannot change anything in the link and linkedlist objects i have instructions to rely rigidly to the textbooks code and i cannot change the data types. i would prefer you to explain the problem and solution in words. language is in Java CODE MAIN FUNCTION import java.util.Scanner;/*** Write a description of class test here.** @author (your name)* @version (a version number or a date)*/public class test{// instance variables - replace the example below with your ownpublic static void main(String[] args){Scanner input = new Scanner(System.in);linkedList list = new linkedList();char character;System.out.println("please enter a string.");String inString =…arrow_forwardcan you give me the code to the following questionarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
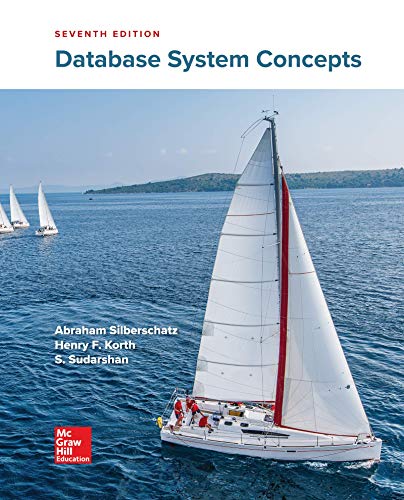
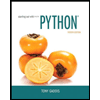
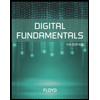
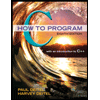
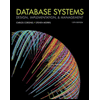
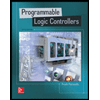