Java programming. A method signature for a method consists of all elements of the method except the body. That is, a method signature consists of the privacy, (non-)static, return datatype, method name, and formal parameters. Consider the ceiling method as an example. public static int ceiling (double num) { return num <= 0 ? (int) num: (int) num + 1; } The method signature of the ceiling method is the first line of the method: public static int ceiling (double num). In this example, we note that ceiling is static because it is a standalone method and does not require an object to invoke (since we are not acting on an instance of a class). For parts a - d, give the method signature described by the scenario. a) A method in class String that returns the reversed version of the current String. b) A method that returns the maximum of two given integers. c) A method that returns true or false if the input integer is an even number. d) A default constructor for class Table.
Java programming.
A method signature for a method consists of all elements of the method except the body. That is, a method signature consists of the privacy, (non-)static, return datatype, method name, and formal parameters. Consider the ceiling method as an example.
public static int ceiling (double num)
{
return num <= 0 ? (int) num: (int) num + 1;
}
The method signature of the ceiling method is the first line of the method: public static int ceiling (double num). In this example, we note that ceiling is static because it is a standalone method and does not require an object to invoke (since we are not acting on an instance of a class).
For parts a - d, give the method signature described by the scenario.
a) A method in class String that returns the reversed version of the current String.
b) A method that returns the maximum of two given integers.
c) A method that returns true or false if the input integer is an even number.
d) A default constructor for class Table.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

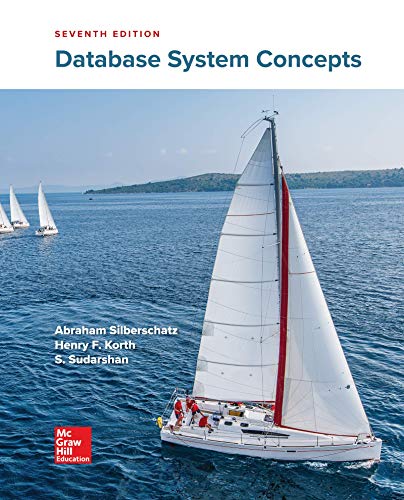
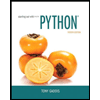
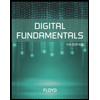
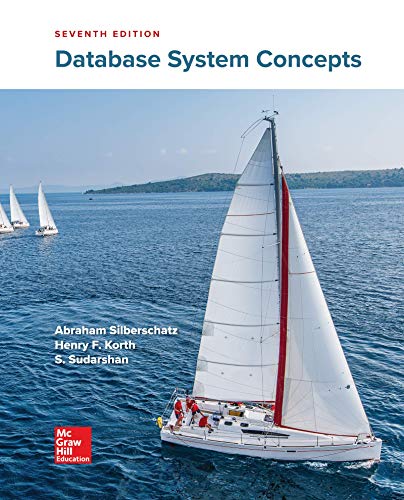
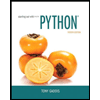
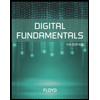
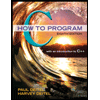
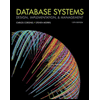
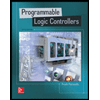