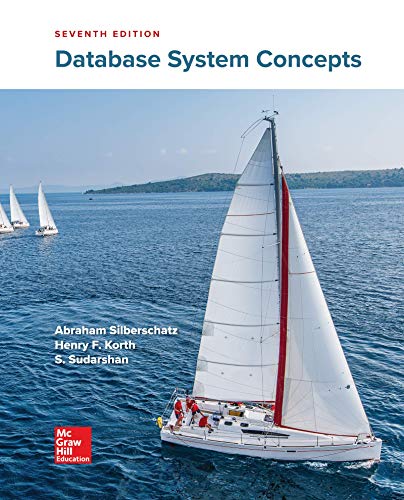
Define an Animal method that updates the time to complete a particular behavior based on its name.
Choose one of the following from the above choices (A, B, C or D within the code):
public class Animal {
// Other instance variables, constructors and methods
// omitted for brevity
// A
public void updateTimeTakenForBehavior(String behaviorName, int timeToComplete) {
for(int i = 0; i < this.behaviorList.length; i++) {
Behavior tempBehavior = this.behaviorList[i];
if( tempBehavior.getBehaviorName().equals(behaviorName)) {
tempBehavior.setTimeToComplete(timeToComplete);
}
}
}
// B
public void updateTimeTakenForBehavior(String behaviorName, int timeToComplete) {
for(int i = 0; i < this.behaviorList.length; i++) {
Behavior tempBehavior = this.behaviorList[i];
if( !tempBehavior.getBehaviorName().equals(behaviorName) ) {
tempBehavior.setTimeToComplete(timeToComplete);
}
}
}
// C
public void updateTimeTakenForBehavior(String behaviorName, int timeToComplete) {
for(int i = 0; i < this.behaviorList.LENGTH(); i++) {
Behavior tempBehavior = this.behaviorList[i];
if( tempBehavior.getBehaviorName().equals(behaviorName) ) {
tempBehavior.setTimeToComplete(timeToComplete);
}
}
}
// D
public void updateTimeTakenForBehavior(String behaviorName, int timeToComplete) {
for(int i = this.behaviorList.length; i < this.behaviorList.length; i++) {
Behavior tempBehavior = this.behaviorList[i];
if( tempBehavior.getBehaviorName().equals(behaviorName) ) {
tempBehavior.setTimeToComplete(timeToComplete);
}
}
}
}

Step by stepSolved in 2 steps

- Find the error(s) in the following code snippet and explain how to correct it (them): 1 class Example { 2 public: Example(int y = 3 10) : data (y) {} 4 int getIncrementedData() const { return ++data; 7 } 8. 9 private: 10 int data; 11 };arrow_forward2-7 Given the code below, which lines are valid and invalid.arrow_forwardpublic class process{ static int result; public static int addition(int a, int b) { result = a + b; return result; } public static int subtraction(int a, int b) { result = a-b; return result; } public static int multiplication(int a, int b) { result = a*b; return result; } public static void main(String[] args) { System.out.print("Enter First Number :"); Scanner s = new Scanner(System.in); int a = s.nextint(); System.out.print("Enter Second Number:"); Scanner p = new Scanner(System.in); int b = p.nextint(); addition(a,b); System.out.printin("First Number :" +a); System.out.println("Second Number :" +b); System.out.printin("Addition Result :" +result): substruction(a,b); System.out .printin("Subtraction Result:" +result); multiplaction(a,b); System.out.printin("Multiplication Result "+result); !3! What does the above program do? Explain it.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
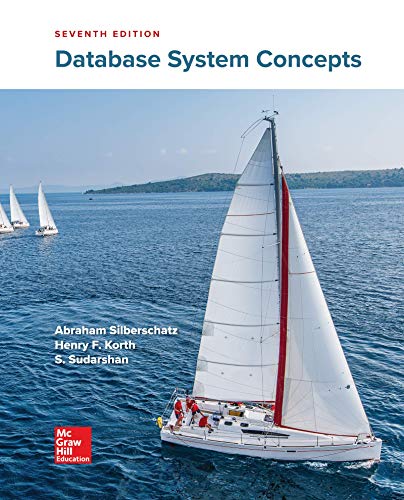
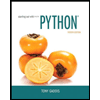
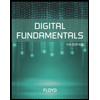
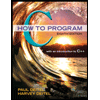
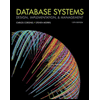
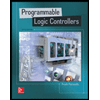