Java Programming Language only: I am asking this questio because I want to compare it to the code that I have and to see if I am in the right check or does it make sense. Thank you! Design and implement a GUI program to convert a positive number given in one base to another base. For this problem, assume that both bases are less than or equal to 10. Consider the sample data: number = 2010, base = 3, and new base = 4. In this case, first convert 2010 in base 3 into the equivalent number in base 10 as follows: 2 * 3^3 + 0 * 3^2 + 1 * 3 + 0 = 54 + 0 + 3 + 0 = 57 To convert 57 to base 4, you need to finde the remainders obtained by dividing by 4, as shown in the following; 57 % 4 = 1, quotient = 14 14 % 4 2, quotient = 3 3 % 4 =3, quotient = 0. Therefore, 57 in base 4 is 321. Here is my code: String inputnumber; int base; //int outputbase; int newbase; System.out.println("Enter the base number to convert from: "); base = console.nextInt(); System.out.println("The base number you want to convert from is: " + base); System.out.println("Enter the number: "); inputnumber = console.next(); System.out.println("The number you entered is: " + inputnumber); System.out.println("Enter the new base to convert to: "); newbase = console.nextInt(); System.out.println("The new base you want to convert to is: " + newbase); // System.out.println("The number " + inputnumber + " in base " + base // + "is" + + " in" + newbase); if (base < 0 || base >= 11) { System.out.println("You entered an invalid base. The base should be between 1 and 10"); } int x; x = inputnumber.length(); int number = Integer.parseInt(inputnumber); int sum = 0; for (int i = 0; i < x; i++) { sum = sum + number%10 * (int)Math.pow(base, i); number = (number - number%10)/10;} System.out.println(sum); String reverse = " "; String output = " "; int i ; int y ; do { y = sum % newbase; reverse += String.valueOf(y); sum = (sum / newbase) ; // i++; // System.out.print(y); } while (sum > 0 ); for (i = reverse.length() - 1; i > 0; i--) { output += reverse.charAt(i); }System.out.println("The number " + inputnumber + " in base " + base + " is " + output + " in base " + newbase) ; System.out.println();
Java
Design and implement a GUI program to convert a positive number given in one base to another base. For this problem, assume that both bases are less than or equal to 10. Consider the sample data:
number = 2010, base = 3, and new base = 4. In this case, first convert 2010 in base 3 into the equivalent number in base 10 as follows:
2 * 3^3 + 0 * 3^2 + 1 * 3 + 0 = 54 + 0 + 3 + 0 = 57
To convert 57 to base 4, you need to finde the remainders obtained by dividing by 4, as shown in the following;
57 % 4 = 1, quotient = 14
14 % 4 2, quotient = 3
3 % 4 =3, quotient = 0. Therefore, 57 in base 4 is 321.
Here is my code:
String inputnumber;
int base;
//int outputbase;
int newbase;
System.out.println("Enter the base number to convert from: ");
base = console.nextInt();
System.out.println("The base number you want to convert from is: " + base);
System.out.println("Enter the number: ");
inputnumber = console.next();
System.out.println("The number you entered is: " + inputnumber);
System.out.println("Enter the new base to convert to: ");
newbase = console.nextInt();
System.out.println("The new base you want to convert to is: " + newbase);
// System.out.println("The number " + inputnumber + " in base " + base
// + "is" + + " in" + newbase);
if (base < 0 || base >= 11) {
System.out.println("You entered an invalid base. The base should be between 1 and 10");
}
int x;
x = inputnumber.length();
int number = Integer.parseInt(inputnumber);
int sum = 0;
for (int i = 0; i < x; i++)
{ sum = sum + number%10 * (int)Math.pow(base, i);
number = (number - number%10)/10;}
System.out.println(sum);
String reverse = " "; String output = " ";
int i ;
int y ;
do {
y = sum % newbase;
reverse += String.valueOf(y);
sum = (sum / newbase) ;
// i++;
// System.out.print(y);
}
while (sum > 0 );
for (i = reverse.length() - 1; i > 0; i--)
{
output += reverse.charAt(i);
}System.out.println("The number " + inputnumber + " in base " + base
+ " is " + output + " in base " + newbase) ;
System.out.println();

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

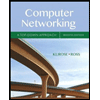
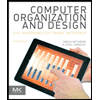
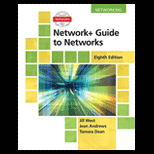
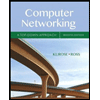
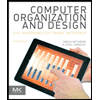
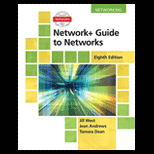
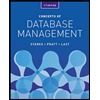
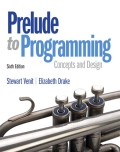
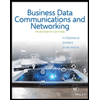