JAVA PROGRAMMING Arrays are useful to process lists. A top-level domain (TLD) name is the last part of an Internet domain name like .com in example.com. A core generic top-level domain (core gTLD) is a TLD that is either .com, .net, .org, or .info. A restricted top-level domain is a TLD that is either .biz, .name, or .pro. A second-level domain is a single name that precedes a TLD as in apple in apple.com. The following program repeatedly prompts for a domain name, and indicates whether that domain name consists of a second-level domain followed by a core gTLD. Valid core gTLD's are stored in an array. For this program, a valid domain name must contain only one period, such as apple.com, but not support.apple.com. The program ends when the user presses just the Enter key in response to a prompt. 1-Run the program and enter domain names to validate. 2-Extend the program to also recognize restricted TLDs using an array, and statements to validate against that array. The program should also report whether the TLD is a core gTLD or a restricted gTLD. Run the program again. import java.util.Scanner; public class GtldValidation { public static void main (String [ ] args) { Scanner scnr = new Scanner(System.in); // Define the list of valid core gTLDs String [ ] validCoreGtld = { ".com", ".net", ".org", ".info" }; // FIXME: Define an array named validRestrictedGtld that has the names // of the restricted domains, .biz, .name, and .pro String inputName = ""; String searchName = ""; String theGtld = ""; boolean isValidDomainName = false; boolean isCoreGtld = false; boolean isRestrictedGtld = false; int periodCounter = 0; int periodPosition = 0; int i = 0; System.out.println("\nEnter the next domain name ( to exit): "); inputName = scnr.nextLine(); while (inputName.length() > 0) { searchName = inputName.toLowerCase(); isValidDomainName = false; isCoreGtld = false; isRestrictedGtld = false; // Count the number of periods in the domain name periodCounter = 0; for (i = 0; i < searchName.length(); ++i) { if (searchName.charAt(i) == '.') { ++periodCounter; periodPosition = i; } } // If there is exactly one period that is not at the start // or end of searchName, check if the TLD is a core gTLD or a restricted gTLD if ((periodCounter == 1) && (searchName.charAt(0) != '.') && (searchName.charAt(searchName.length() - 1) != '.')) { isValidDomainName = true; } if (isValidDomainName) { // Extract the Top-level Domain name starting at the period's position. Ex: // If searchName = "example.com", the next statement extracts ".com" theGtld = searchName.substring(periodPosition); i = 0; while ((i < validCoreGtld.length) && (!isCoreGtld)) { if(theGtld.equals(validCoreGtld[i])) { isCoreGtld = true; } else { ++i; } } // FIXME: Check to see if the gTLD is not a core gTLD. If it is not, // check to see whether the gTLD is a valid restricted gTLD. // If it is, set isRestrictedGtld to true } System.out.print("\"" + inputName + "\" "); if (isValidDomainName) { System.out.print("is a valid domain name and "); if (isCoreGtld) { System.out.println("has a core gTLD of \"" + theGtld + "\"."); } else if (isRestrictedGtld) { System.out.println("has a restricted gTLD of \"" + theGtld + "\"."); } else { System.out.println("does not have a core gTLD."); // FIXME update message } } else { System.out.println("is not a valid domain name."); } System.out.println("\nEnter the next domain name ( to exit): "); inputName = scnr.nextLine(); } return; } }
JAVA
Arrays are useful to process lists.
A top-level domain (TLD) name is the last part of an Internet domain name like .com in example.com. A core generic top-level domain (core gTLD) is a TLD that is either .com, .net, .org, or .info. A restricted top-level domain is a TLD that is either .biz, .name, or .pro. A second-level domain is a single name that precedes a TLD as in apple in apple.com.
The following program repeatedly prompts for a domain name, and indicates whether that domain name consists of a second-level domain followed by a core gTLD. Valid core gTLD's are stored in an array. For this program, a valid domain name must contain only one period, such as apple.com, but not support.apple.com. The program ends when the user presses just the Enter key in response to a prompt.
1-Run the program and enter domain names to validate.
2-Extend the program to also recognize restricted TLDs using an array, and statements to validate against that array. The program should also report whether the TLD is a core gTLD or a restricted gTLD. Run the program again.
import java.util.Scanner;
public class GtldValidation {
public static void main (String [ ] args) {
Scanner scnr = new Scanner(System.in);
// Define the list of valid core gTLDs
String [ ] validCoreGtld = { ".com", ".net", ".org", ".info" };
// FIXME: Define an array named validRestrictedGtld that has the names
// of the restricted domains, .biz, .name, and .pro
String inputName = "";
String searchName = "";
String theGtld = "";
boolean isValidDomainName = false;
boolean isCoreGtld = false;
boolean isRestrictedGtld = false;
int periodCounter = 0;
int periodPosition = 0;
int i = 0;
System.out.println("\nEnter the next domain name (<Enter> to exit): ");
inputName = scnr.nextLine();
while (inputName.length() > 0) {
searchName = inputName.toLowerCase();
isValidDomainName = false;
isCoreGtld = false;
isRestrictedGtld = false;
// Count the number of periods in the domain name
periodCounter = 0;
for (i = 0; i < searchName.length(); ++i) {
if (searchName.charAt(i) == '.') {
++periodCounter;
periodPosition = i;
}
}
// If there is exactly one period that is not at the start
// or end of searchName, check if the TLD is a core gTLD or a restricted gTLD
if ((periodCounter == 1) &&
(searchName.charAt(0) != '.') &&
(searchName.charAt(searchName.length() - 1) != '.')) {
isValidDomainName = true;
}
if (isValidDomainName) {
// Extract the Top-level Domain name starting at the period's position. Ex:
// If searchName = "example.com", the next statement extracts ".com"
theGtld = searchName.substring(periodPosition);
i = 0;
while ((i < validCoreGtld.length) && (!isCoreGtld)) {
if(theGtld.equals(validCoreGtld[i])) {
isCoreGtld = true;
}
else {
++i;
}
}
// FIXME: Check to see if the gTLD is not a core gTLD. If it is not,
// check to see whether the gTLD is a valid restricted gTLD.
// If it is, set isRestrictedGtld to true
}
System.out.print("\"" + inputName + "\" ");
if (isValidDomainName) {
System.out.print("is a valid domain name and ");
if (isCoreGtld) {
System.out.println("has a core gTLD of \"" + theGtld + "\".");
}
else if (isRestrictedGtld) {
System.out.println("has a restricted gTLD of \"" + theGtld + "\".");
}
else {
System.out.println("does not have a core gTLD."); // FIXME update message
}
}
else {
System.out.println("is not a valid domain name.");
}
System.out.println("\nEnter the next domain name (<Enter> to exit): ");
inputName = scnr.nextLine();
}
return;
}
}

Step by step
Solved in 4 steps with 5 images

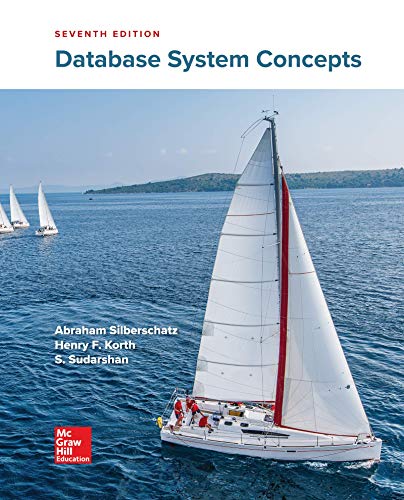
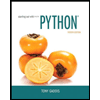
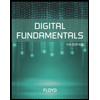
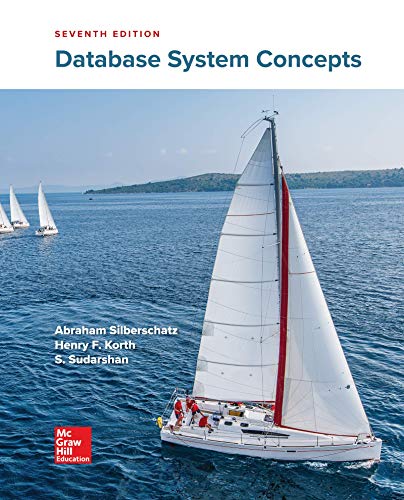
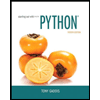
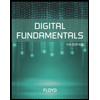
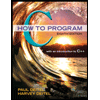
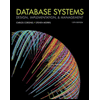
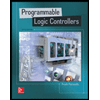