For this assignment, you are to use the started code provided with QUEUE Container Adapter methods and provide the implementation of a requested functionality outlined below. Scenario: A local restaurant has hired you to develop an application that will manage customer orders. Each order will be put in the queue and will be called on a first come first served bases. Develop the menu driven application with the following menu items: Add order Next order Previous order Delete order Order Size View order list View current order Order management will be resolved by utilization of an STL-queue container’s functionalities and use of the following Queue container adapter functions: enQueue: Adds the order in the queue DeQueue: Deletes the order from the queue Peek: Returns the order that is top in the queue without removing it IsEmpty: checks do we have any orders in the queue Size: returns the number of orders that are in the queue While adding a new order in the queue, the program will be capable of collecting the following order information: Name on order Order description Order total Order tip Date of order The started code: #include #include #include using namespace std; class patient { / /Creates class for patient variable public: string firstName; string insuranceType; string lastName; string ssn; string address; string visitDate; }; queue p; void enqueue() //Enqueues patient details from console input { patient pa; cout << "Please enter Patient First Name:"; cin >> pa.firstName; cout << "Please enter Patient Last Name:"; cin >> pa.lastName; cout << "Please enter Patient insurance type:"; cin >> pa.insuranceType; cout << "Please enter Patient SSN:"; cin >> pa.ssn; cout << "Please enter Patient Address:"; cin.ignore(); getline(cin, pa.address); cout << "Please enter Patient Date of Visit:"; cin >> pa.visitDate; p.push(pa); } patient dequeue() //Removes patient from queue { patient pa; if (!p.empty()) { pa = p.front(); p.pop(); } return pa; } void peek() //Returns current patient at the front of the queue { if (!p.empty()) { patient tmp = p.front(); cout << "Patient Name is " << tmp.firstName<< endl; } } int main() { int x = 0; int n; patient last; while (x == 0) { //Prompts for Menu Selection while selection = 0, which is defaulted to 0 cout << "Welcome to Dental Associates of Kansas City" << endl; cout << "**************************************************" << endl; cout << "To get started, please select an option from the menu below:" << endl; cout << "1. Add patient" << endl; cout << "2. Next patient" << endl; cout << "3. Previous patient" << endl; cout << "4. Delete patient" << endl; cout << "5. View current" << endl; cout << "6. Exit this program" << endl; cout << "Enter the number of the action you would like to perform: "; cin >> n; if (n == 1) { enqueue(); } else if (n == 2) { last = dequeue(); peek(); } else if (n == 3) { cout << last.firstName << endl; } else if (n == 4) { last = dequeue(); } else if (n == 5) { peek(); } else if (n == 6) { exit(0); } } }
For this assignment, you are to use the started code provided with QUEUE Container Adapter methods and provide the implementation of a requested functionality outlined below.
Scenario:
A local restaurant has hired you to develop an application that will manage customer orders. Each order will be put in the queue and will be called on a first come first served bases.
Develop the menu driven application with the following menu items:
- Add order
- Next order
- Previous order
- Delete order
- Order Size
- View order list
- View current order
Order management will be resolved by utilization of an STL-queue container’s functionalities and use of the following Queue container adapter functions:
- enQueue: Adds the order in the queue
- DeQueue: Deletes the order from the queue
- Peek: Returns the order that is top in the queue without removing it
- IsEmpty: checks do we have any orders in the queue
- Size: returns the number of orders that are in the queue
While adding a new order in the queue, the program will be capable of collecting the following order information:
- Name on order
- Order description
- Order total
- Order tip
- Date of order
The started code:
#include <iostream>
#include <queue>
#include <string>
using namespace std;
class patient {
/ /Creates class for patient variable
public:
string firstName;
string insuranceType;
string lastName;
string ssn;
string address;
string visitDate;
};
queue <patient> p;
void enqueue()
//Enqueues patient details from console input
{
patient pa;
cout << "Please enter Patient First Name:";
cin >> pa.firstName;
cout << "Please enter Patient Last Name:";
cin >> pa.lastName;
cout << "Please enter Patient insurance type:";
cin >> pa.insuranceType;
cout << "Please enter Patient SSN:";
cin >> pa.ssn;
cout << "Please enter Patient Address:";
cin.ignore();
getline(cin, pa.address);
cout << "Please enter Patient Date of Visit:";
cin >> pa.visitDate; p.push(pa);
}
patient dequeue()
//Removes patient from queue
{
patient pa;
if (!p.empty()) {
pa = p.front();
p.pop();
}
return pa;
}
void peek()
//Returns current patient at the front of the queue
{
if (!p.empty()) {
patient tmp = p.front();
cout << "Patient Name is " << tmp.firstName<< endl;
}
}
int main() {
int x = 0;
int n;
patient last;
while (x == 0) {
//Prompts for Menu Selection while selection = 0, which is defaulted to 0
cout << "Welcome to Dental Associates of Kansas City" << endl; cout << "**************************************************" << endl;
cout << "To get started, please select an option from the menu below:" << endl;
cout << "1. Add patient" << endl;
cout << "2. Next patient" << endl;
cout << "3. Previous patient" << endl;
cout << "4. Delete patient" << endl;
cout << "5. View current" << endl;
cout << "6. Exit this program" << endl; cout << "Enter the number of the action you would like to perform: ";
cin >> n; if (n == 1) {
enqueue();
} else if (n == 2) {
last = dequeue();
peek();
} else if (n == 3) {
cout << last.firstName << endl;
} else if (n == 4) {
last = dequeue();
} else if (n == 5) {
peek();
} else if (n == 6) {
exit(0);
}
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

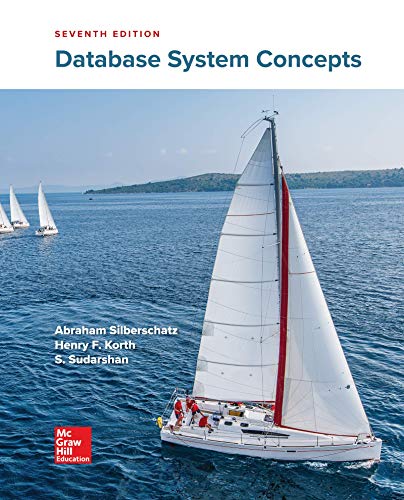
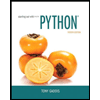
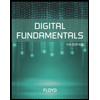
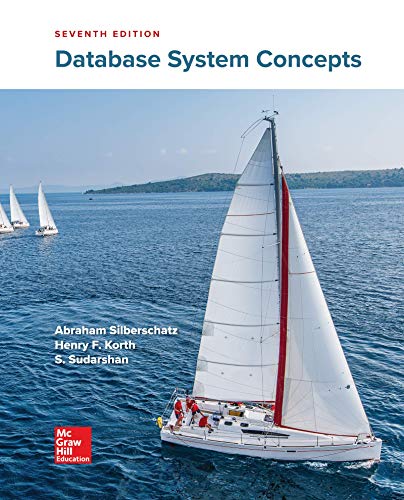
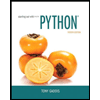
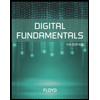
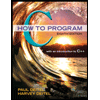
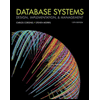
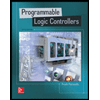