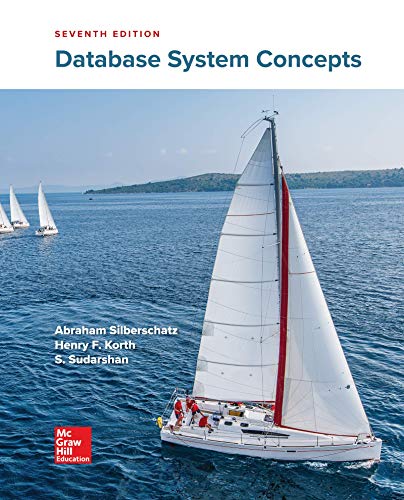
import java.util.*;
public class NameSearcher {
private static List<String> loadFileToList(String filename) throws FileNotFoundException {
List<String> namesList = new ArrayList<>();
File file = new File(filename);
if (!file.exists()) {
throw new FileNotFoundException(filename);
}
try (Scanner scanner = new Scanner(file)) {
while (scanner.hasNextLine()) {
String line = scanner.nextLine().trim();
String[] names = line.split("\\s+");
for (String name : names) {
namesList.add(name.toLowerCase()); // Convert names to lowercase
}
}
}
return namesList;
}
private static Integer searchNameInList(String name, List<String> namesList) {
name = name.toLowerCase(); // Convert user input to lowercase
int index = namesList.indexOf(name);
return index == -1 ? null : index + 1;
}
public static void main(String[] args) {
List<String> boyNames;
List<String> girlNames;
boolean boyFileExists = false;
boolean girlFileExists = false;
try {
boyNames = loadFileToList("Boynames.txt");
boyFileExists = true;
} catch (FileNotFoundException e) {
boyNames = new ArrayList<>();
}
try {
girlNames = loadFileToList("Girlnames.txt");
girlFileExists = true;
} catch (FileNotFoundException e) {
girlNames = new ArrayList<>();
}
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.print("Enter a name to search or type QUIT to exit:\n");
String input = scanner.nextLine().trim();
if (input.equalsIgnoreCase("QUIT")) {
break;
}
Integer boyIndex = searchNameInList(input, boyNames);
Integer girlIndex = searchNameInList(input, girlNames);
if (boyIndex == null && girlIndex == null) {
System.out.println("The name '" + input + "' was not found in either list.");
} else {
if (boyIndex != null && girlIndex != null) {
System.out.println("The name '" + input + "' was found in both lists: boy names (line " + boyIndex + ") and girl names (line " + girlIndex + ").");
} else if (boyIndex != null) {
System.out.println("The name '" + input + "' was found in popular boy names list (line " + boyIndex + ").");
} else {
System.out.println("The name '" + input + "' was found in popular girl names list (line " + girlIndex + ").");
}
}
}
scanner.close();
}
}
Test Case 1
AnnabelleENTER
The name 'Annabelle' was not found in either list.\n
Enter a name to search or type QUIT to exit:\n
xavierENTER
The name 'Xavier' was found in popular boy names list (line 81).\n
Enter a name to search or type QUIT to exit:\n
AMANDAENTER
The name 'Amanda' was found in popular girl names list (line 63).\n
Enter a name to search or type QUIT to exit:\n
jOrdAnENTER
The name 'Jordan' was found in both lists: boy names (line 38) and girl names (line 75).\n
Enter a name to search or type QUIT to exit:\n
quitENTER
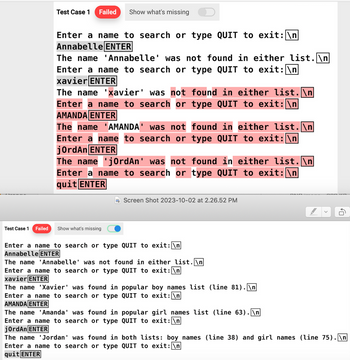
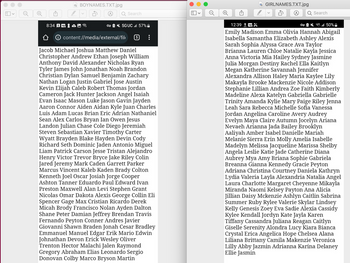

Step by stepSolved in 3 steps with 1 images

- New JAVA code can only be added between lines 9 and 10, as seen in image.arrow_forwardI ran the code and got an error. I even created a main.java file to run the test cases. Please fix the error and provide me the correct code for all parts. Make sure to give the screenshot of the output as well.arrow_forwardLab2.java contains the following code: public final class Lab2 { /** * This is empty by design, Lab2 cannot be instantiated */ private Lab2() { // empty by design } /** * Returns the sum of a consecutive set of numbers from <code> start </code> to <code> end </code>. * * @pre <code> start </code> and <code> end </code> are small enough to let this * method return an int. This means the return value at most requires 4 bytes and * no overflow would happen. * * @param start is an integer number * @param end is an integer number * @return the sum of start + (start + 1) + .... + end */ public static int sum(int start, int end) { //Insert your code here and change the return statement to fit your implementation. return 0; } /** * This method creates a string using the given char * by repeating the character <code> n </code> times. * * @param first is…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
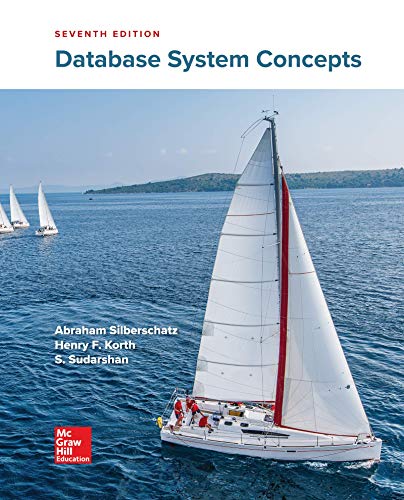
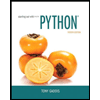
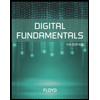
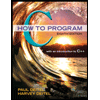
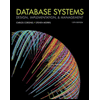
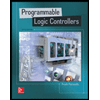