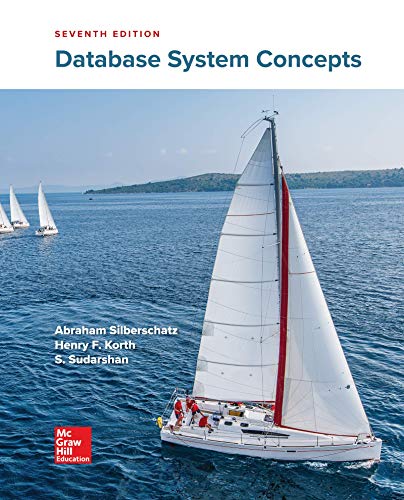
import java.io.*;
import java.util.ArrayList;
import java.util.Scanner;
public class ArrayOperations {
// Method to calculate the total of array elements
public static double getTotal(double[] array) {
double total = 0;
for (double value : array) {
total += value;
}
return total;
}
// Method to calculate the average of array elements
public static double getAverage(double[] array) {
return getTotal(array) / array.length;
}
// Method to find the highest value in the array
public static double getHighest(double[] array) {
double highest = array[0];
for (double value : array) {
if (value > highest) {
highest = value;
}
}
return highest;
}
// Method to find the lowest value in the array
public static double getLowest(double[] array) {
double lowest = array[0];
for (double value : array) {
if (value < lowest) {
lowest = value;
}
}
return lowest;
}
// Main function
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
// Prompt the user for the filename
System.out.print("Enter the filename: ");
String filename = scanner.nextLine();
// Read data from the file and perform calculations
try (Scanner fileReader = new Scanner(new File(filename))) {
ArrayList<Double> arrayList = new ArrayList<>();
// Read file and populate ArrayList
while (fileReader.hasNextDouble()) {
arrayList.add(fileReader.nextDouble());
}
// Convert ArrayList to array
double[] array = new double[arrayList.size()];
for (int i = 0; i < arrayList.size(); i++) {
array[i] = arrayList.get(i);
}
// Display results by calling helper methods
System.out.println("Total: " + getTotal(array));
System.out.println("Average: " + getAverage(array));
System.out.println("Highest: " + getHighest(array));
System.out.println("Lowest: " + getLowest(array));
} catch (FileNotFoundException e) {
System.out.println("File: " + filename + " does not exist.");
} finally {
// Close the scanner
scanner.close();
}
}
}
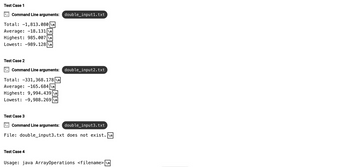
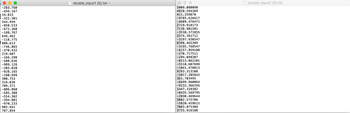

Step by stepSolved in 3 steps with 2 images

- Please solve this computer science problem.arrow_forwardJAVA ARRAY MANIPULATION QUESTION: make a method called manipulator that takes a double array as parameter and modifies it. The method will modify the array then print the array. The method must modify the existing array, it CAN NOT create a different array as the solution. The manipulator method will be a void method. Remember: To get an average of 2 digits you add them together then divide by 2. Average of x and y = (x+y)/2 Modifications the method will do: For every 2 consecutive elements in the array, both the elements are replaced by their average. If the number of elements in an array is odd don't modify the last number of the array. Basically, you find the average of 2 consecutive elements then replace both of the elements with that value. Examples: Array = {2.0,3.0} will be changed to {2.5,2.5} when passed to the manipulator method Array = {2.0,3.0,45} will be changed to {2.5,2.5,45} when passed to the manipulator method Array = {2.0,3.0,45,55} will be changed to…arrow_forwardLw//. Two-Dimensional ArrayInstruction:Create a java program that generates elements (randomly from 10 – 75) of a 2-dimensional array (5x5) using the Random Class then perform the following:1) Output the array elements2) Output the sum of prime numbers in the array3) Output the elements in the main diagonal.4) Output the sum of the elements below the diagonal.5) Output the sum of the elements above the diagonal.6) Output the odd numbers below the diagonal.7) Output the even numbers above the diagonal. NOTE: Please do not use the array methods available on the internet. Use the for loop, while loop or do-while loop statement in your codes..arrow_forward
- Can you please answer the following question. The program is in JavaScript and must use readlineSync. Thank you! Create a file named dedup.js. Write a program that removes duplicates from an array. The program should be able to read an unspecified number of numbers input by the user. The user should enter a negative number when they are done entering scores. The program should remove all duplicates from the array and then iterate over the new array and display it. Create a file named dedeup_2.js. Modify your dedup program and prompt the user to enter a number n. Remove all elements from the array that occur more than n times.arrow_forward3. Complete the program Student Records that simulates a student records system. The program reads a list of student ids, names, and GPAS from a file exam.txt, and stores the information in parallel array lists ids, names, and gpas. Sample input (exam.txt): 132 Woody 4.1 465 Buzz 3.1 443 Rex 2.8 124 Hamm 3.3 337 Jessie 4.2 Your program will display a GPA report. You must include a method named reportByGPA (...) that accepts the required array lists and minimum GPA and displays the list of students that meet the criteria. Sample output: Enter the minimum GPA: 4.0 Students with GPA of 4.0 or higher 132 Woody, GPA: 4.1 337 Jessie, GPA: 4.2 end of program import java.util.ArrayList; import java.util.Scanner; import java.io.File; import java.io.FileNotFoundException; public class Student Records { public static void main(String[] args) throws FileNotFoundException ( Scanner f = new Scanner (new File ("exam.txt")); Scanner kb = new Scanner (System.in); ArrayList ids = new ArrayList ();…arrow_forwardProgramming language: Processing from Java Question attached as photo Topic: Use of Patial- Full Arraysarrow_forward
- Debugging challenge: Consider the code example below. This program is designed to compare the contents of two arrays: if they are equal, the program should display "TRUE," if the arrays are not equal, the program should display "FALSE". However, even when the arrays have the same value, the program always prints "FALSE", regardless of the values in arrays a and b. What is going on here? What about the program causes the comparison to always fail? int a[4] {1, 2, 3, 4}; int b[4] = {1, 2, 3, 4}; if (a == b) { display ("TRUE"); else { display ("FALSE");arrow_forwardIn c++, Declare a constant “ SIZE = 5 ” Declare an array of ints , of size SIZE . Initializethat array to have the values 10, 12, 15, 19, 6 .– Write a loop that calculates and prints out the minimum value of the array.– Write a loop that calculates and prints out the maximum value of the array.– Write a loop that calculates and prints out the average value of the array.arrow_forward2arrow_forward
- Problem Description - JAVA PROGRAMMING Use a Two-dimensional (3x3) array to solve the following problem: Write an application that inputs nine numbers, each of which is between 1 and 10, inclusive. Display the array after the user inputs each value. Rotate/flip the array by changing places. Make the rows columns and vice versa. You have to move the elements to their new locations. Remember to validate the input and display an error message if the user inputs invalid data. Documentation and the screenshot(s) of the results. Example: 1 2 3 4 5 6 7 8 9 the result will be : 1 4 7 2 5 8 3 6 9arrow_forwardWrite 3 functions: Write int readNumbersIntoArray(int numbers[], int size); // Reads up to size numbers or a non-numeric and puts them in the array. // Returns how many numbers read. Write int minOrMax(int numbers[], int size, bool Max); // Returns the minimum or the maximum value in the array depending on "Max". // If "Max" is true, then it returns the maximum. // If "Max" is false, then it returns the minimum. Write double trimmedAvg(int numbers[], int size); // Returns the trimmed average of the array. // A trimmed average is a normal average but leaves out the first instance of // the smallest and largest value in the array when doing the calculation. // HINT: use calls to minOrMax() to get the largest and smallest number. Write int main(): // Prompt the user for between 3 and 20 numbers and use readNumbersIntoArray() to // read them into an array. Print an error message and exit if less than three numbers. // Print out the min, max, and trimmedAverage of the array using calls…arrow_forwardA file USPopulation.txt contains the population of the US starting in year 1950 and then each subsequent record has the population for the following year. USPopulation.txtDownload USPopulation.txt Write a program that uses an array with the file that displays these in a menu and then produces the results. This is not an Object Oriented Program but should be a procedural program, calling methods to do the following: 1: Displays the year and the population during that year 2. The average population during that time period (Add up the populations of all records and divide by the number of years). 3. The year with the greatest increase in population - print the year and that population and that amount. To figure this out, compare the difference in population before of say year 1950 and 1951, store that difference somewhere. Compare 1951 with 1952, find that difference. Is that difference greater than the stored difference? If so, move that the the maximum place. 4.…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
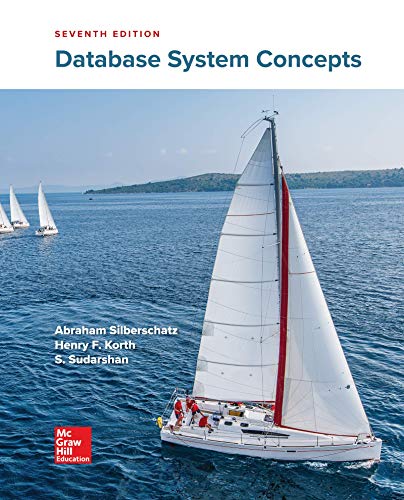
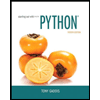
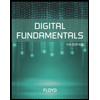
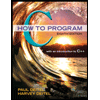
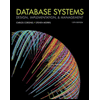
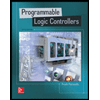