1) UDP Echo Server Receiving and Displaying Messages: The UDP Echo server should be able to receive messages sent by clients. For each received message, it should display the address from which the message was received and the content of the message. Echoing Messages Back: After receiving a message, the server should echo it back to the client who sent it. It should print the message that was sent and the address to which it was sent. 2) UDP Echo Client User Input: The UDP Echo client should interact with the user. It should ask the user to input a message that they want to send to the server and specify the number of times they want to send this message. Sending Messages: Using a Python loop, the client should send the user-specified message the specified number of times to the server's IP address and port.
UDP Echo Application
A UDP Echo program is a simple network application that demonstrates the basic
principles of UDP communication. In this program, a server listens for incoming UDP
packets from clients, and when it receives a packet, it echoes (sends back) the same
packet to the client. The client sends a message to the server, and the server returns the
same message. It's often used for testing network connectivity and understanding the
fundamentals of UDP communication.
(Answer the following question using python)
Implement both the server and client components of this UDP Echo program while
adhering to the features outlined below. Please ensure that your implementation follows
good coding practices and provides clear prompts and feedback to the user, making it
user-friendly and robust. Please refer to the class example to guide your implementation.
1) UDP Echo Server
- Receiving and Displaying Messages: The UDP Echo server should be able to
receive messages sent by clients. For each received message, it should display the
address from which the message was received and the content of the message. - Echoing Messages Back: After receiving a message, the server should echo it
back to the client who sent it. It should print the message that was sent and the
address to which it was sent.
2) UDP Echo Client
- User Input: The UDP Echo client should interact with the user. It should ask the
user to input a message that they want to send to the server and specify the
number of times they want to send this message. - Sending Messages: Using a Python loop, the client should send the user-specified
message the specified number of times to the server's IP address and port.



Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

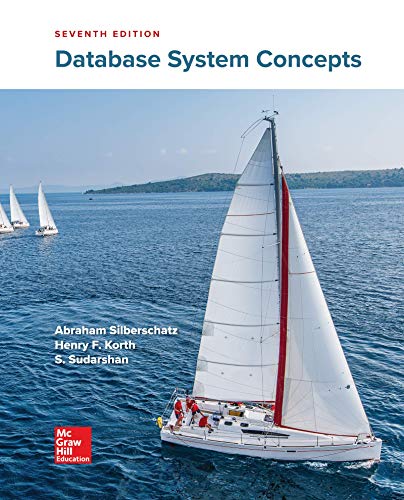
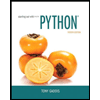
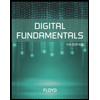
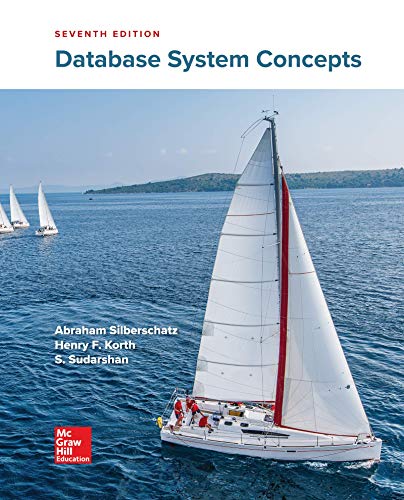
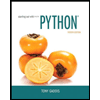
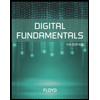
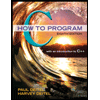
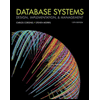
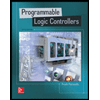