Java program: 1. Create a class named Rectangle that has instance variables height and width. Provide a constructor that initializes the instance variables based on parameter values, getter and setter methods for the instance variables, a toString method, and a method named computeSurfaceArea(), that returns the surface area of the rectangle. 2. Create a child class named RectPrism that contains an additional instance variable named depth. Provide a constructor, getter and setter methods for the new instance variable, and a method named computeVolume(), that returns the volume of the rectangular prism. Override the toString() and the computeSurfaceArea() methods. 3. Write an application called Demo, that instantiates a rectangle and a rectangular prism, and tests all the methods.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Java program:
1. Create a class named Rectangle that has instance variables height and width.
Provide a constructor that initializes the instance variables based on parameter
values, getter and setter methods for the instance variables, a toString method,
and a method named computeSurfaceArea(), that returns the surface area of
the rectangle.
2. Create a child class named RectPrism that contains an additional instance
variable named depth. Provide a constructor, getter and setter methods for the
new instance variable, and a method named computeVolume(), that returns
the volume of the rectangular prism. Override the toString() and the
computeSurfaceArea() methods.
3. Write an application called Demo, that instantiates a rectangle and a
rectangular prism, and tests all the methods.
Make sure you indent and comment your code based on the examples in the
textbook. Don’t forget to include your name, the course number, title of the
assignment, and today’s date.

Step by step
Solved in 4 steps with 1 images

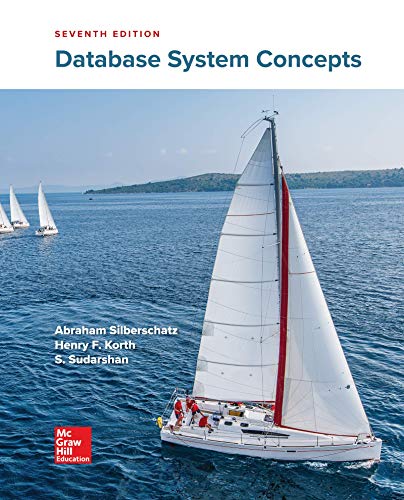
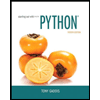
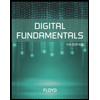
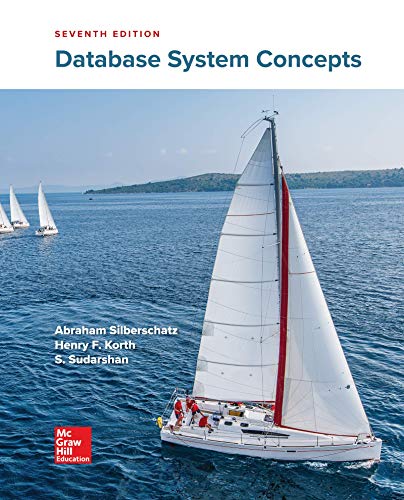
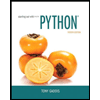
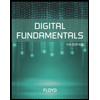
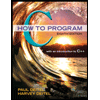
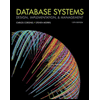
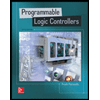