JAVA: Debit Card Reader (interfaces) CashRegister.java is the ONLY FILE the can be edited!!!! Many machines are able to make use of debit cards in order for people to make transactions using the money in their bank accounts. One piece of a debit card's security is its PIN number, which must be entered correctly for a transaction to proceed. The main() program below defines a cash register and a debit card with the pin 1234, and then accepts a PIN number as input. The entered PIN number is passed to the cash register along with the debit card object to verify that the entered PIN was correct. Given main(), a DebitCard class, and a DebitCardReader interface, complete the definition for the CashRegister class. The CashRegister class should provide a definition for the abstract method from the interface it implements. The program will be complete once this abstract method is defined correctly. Ex. If the input is: 1234 the output should be: Transaction succeeded! If the input is: 1111 the output should be: Transaction failed. Templet files: Transaction.java import java.util.Scanner; public class Transaction { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); CashRegister register = new CashRegister(); DebitCard card = new DebitCard(1234); int pin; pin = scnr.nextInt(); if (register.verifyCardPIN(card, pin)) { System.out.println("Transaction succeeded!"); } else { System.out.println("Transaction failed."); } }} DebitCard.java public class DebitCard { private int pin; public DebitCard(int newpin) { pin = newpin; } public int getPIN() { return pin; } public void setPIN(int newpin) { pin = newpin; }} DebitCardReader.java public interface DebitCardReader { public boolean verifyCardPIN(DebitCard card, int pin);} CashRegister.java public class CashRegister implements DebitCardReader {
Oh no! Our experts couldn't answer your question.
Don't worry! We won't leave you hanging. Plus, we're giving you back one question for the inconvenience.
JAVA: Debit Card Reader (interfaces)
CashRegister.java is the ONLY FILE the can be edited!!!!
Many machines are able to make use of debit cards in order for people to make transactions using the money in their bank accounts. One piece of a debit card's security is its PIN number, which must be entered correctly for a transaction to proceed. The main() program below defines a cash register and a debit card with the pin 1234, and then accepts a PIN number as input. The entered PIN number is passed to the cash register along with the debit card object to verify that the entered PIN was correct.
Given main(), a DebitCard class, and a DebitCardReader interface, complete the definition for the CashRegister class. The CashRegister class should provide a definition for the abstract method from the interface it implements. The program will be complete once this abstract method is defined correctly.
Ex. If the input is:
1234the output should be:
Transaction succeeded!If the input is:
1111the output should be:
Transaction failed.import java.util.Scanner;
public class Transaction {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
CashRegister register = new CashRegister();
DebitCard card = new DebitCard(1234);
int pin;
pin = scnr.nextInt();
if (register.verifyCardPIN(card, pin)) {
System.out.println("Transaction succeeded!");
}
else {
System.out.println("Transaction failed.");
}
}
}
DebitCard.java
public class DebitCard {
private int pin;
public DebitCard(int newpin) {
pin = newpin;
}
public int getPIN() {
return pin;
}
public void setPIN(int newpin) {
pin = newpin;
}
}
DebitCardReader.java
public interface DebitCardReader {
public boolean verifyCardPIN(DebitCard card, int pin);
}
CashRegister.java
public class CashRegister implements DebitCardReader {
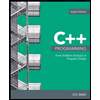
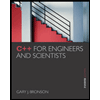
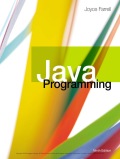
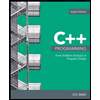
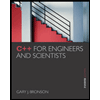
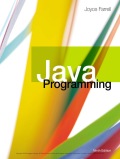
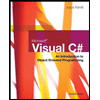
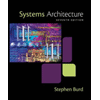