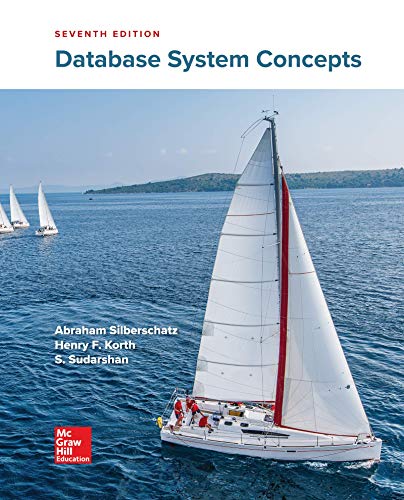
Concept explainers
15.11 LAB: Sorting user IDs C++
Given a main() that reads user IDs (until -1), complete the Quicksort() and Partition() functions to sort the IDs in ascending order using the Quicksort
Ex. If the input is:
kaylasimmsthe output is:
julia
#include <string>
#include <
#include <iostream>
using namespace std;
// TODO: Write the partitioning algorithm - pick the middle element as the
// pivot, compare the values using two index variables l and h (low and high),
// initialized to the left and right sides of the current elements being sorted,
// and determine if a swap is necessary
int Partition(vector<string> &userIDs, int i, int k) {
}
// TODO: Write the quicksort algorithm that recursively sorts the low and
// high partitions
void Quicksort(vector<string> &userIDs, int i, int k) {
}
int main(int argc, char* argv[]) {
vector<string> userIDList;
string userID;
cin >> userID;
while (userID != "-1") {
userIDList.push_back(userID);
cin >> userID;
}
// Initial call to quicksort
Quicksort(userIDList, 0, userIDList.size() - 1);
for (size_t i = 0; i < userIDList.size(); ++i) {
cout << userIDList.at(i) << endl;;
}
return 0;
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- 11.10 LAB: All permutations of names C++ Write a program that lists all ways people can line up for a photo (all permutations of a list of strings). The program will read a list of one word names (until -1), and use a recursive method to create and output all possible orderings of those names, one ordering per line. When the input is: Julia Lucas Mia -1 then the output is (must match the below ordering): Julia Lucas Mia Julia Mia Lucas Lucas Julia Mia Lucas Mia Julia Mia Julia Lucas Mia Lucas Julia Please help with //TODO #include <vector>#include <string>#include <iostream> using namespace std; // TODO: Write method to create and output all permutations of the list of names.void AllPermutations(const vector<string> &permList, const vector<string> &nameList) { } int main(int argc, char* argv[]) {vector<string> nameList;vector<string> permList;string name; // TODO: Read in a list of names; stop when -1 is read. Then call…arrow_forward5.12 LAB: User-Defined Functions: Adjust list by normalizing CORAL LANGUAGE ONLY PLEASE When analyzing data sets, such as data for human heights or for human weights, a common step is to adjust the data. This can be done by normalizing to values between 0 and 1, or throwing away outliers. Define a function named getMinimumInt that takes an integer array as a parameter and returns the smallest value. Then write a main program that reads five integers as input, stores the integers in an array, and calls getMinimumInt() with the array as an argument. The main program then outputs the normalized array by subtracting the returned smallest value from all the values in the array. Ex: If the input is: 30 50 10 70 65 function getMinimumInt() returns 10, and the program output is: 20 40 0 60 55 For coding simplicity, follow every output value by a space, even the last one. Your program should define and use a function:Function getMinimumInt(integer array(?) userVals) returns integer minInt…arrow_forwardA1.1.6 (a) create the recursive function BinaryGCd (a,b) based on the following pseudo code # recursive pseudo-code def BinaryGCF (a,b): arrange order so ab if a=0: if a and b are even: else if a is even: else if b is even: else: done, b is GCF GCF (a,b) = 2*GCF (a/2, b/2) GCF (a,b) = GCF (a/2, b) GCF (a,b) GCF (a,b) In [ ]: def BinaryGCF (a,b): GCF (a,b/2) GCF (a,b-a) (b) demonstrate that it gives the same results as pfGCD (a,b) and EuclidGCD (a, b) from A1.5. "enter the recursive code below" for a,b in [(660,350), (99,53), (30,84)]: # demonstrate all GCD functions give the same result 13arrow_forward
- C++ please 3.9.3: Populating a vector with a for loop. Write a for loop to populate vector userGuesses with NUM_GUESSES integers. Read integers using cin. Ex: If NUM_GUESSES is 3 and user enters 9 5 2, then userGuesses is {9, 5, 2}.arrow_forward8.16 LAB: Mileage tracker for a runner C++ Given the MileageTrackerNode class, complete main() to insert nodes into a linked list (using the InsertAfter() function). The first user-input value is the number of nodes in the linked list. Use the PrintNodeData() function to print the entire linked list. DO NOT print the dummy head node. Ex. If the input is: 3 2.2 7/2/18 3.2 7/7/18 4.5 7/16/18 the output is: 2.2, 7/2/18 3.2, 7/7/18 4.5, 7/16/18 Main.cpp #include "MileageTrackerNode.h"#include <string>#include <iostream>using namespace std; int main (int argc, char* argv[]) {// References for MileageTrackerNode objectsMileageTrackerNode* headNode;MileageTrackerNode* currNode;MileageTrackerNode* lastNode; double miles;string date;int i; // Front of nodes listheadNode = new MileageTrackerNode();lastNode = headNode; // TODO: Read in the number of nodes // TODO: For the read in number of nodes, read// in data and insert into the linked list // TODO: Call the…arrow_forward10.1 Write a template function that returns the average of all the elements of an array. Thearguments to the function should be the array name and the size of the array (type int). In main (),exercise the function with arrays of type int, long, and double. 10.2 Create a function called amax() that returns the value of the largest element in an array. Thearguments to the function should be the address of the array and its size. Make this function into atemplate so it will work with an array of any numerical type. Write a main () program that appliesthis function to arrays of various types.arrow_forward
- 15.14 LAB: Binary search Binary search can be implemented as a recursive algorithm. Each call makes a recursive call on one-half of the list the call received as an argument. Complete the recursive function BinarySearch() with the following specifications: Parameters: a target integer a vector of integers lower and upper bounds within which the recursive call will search Return value: the index within the vector where the target is located -1 if target is not found The template provides the main program and a helper function that reads a vector from input. The algorithm begins by choosing an index midway between the lower and upper bounds. If target == integers.at(index) return index If lower == upper, return -1 to indicate not found Otherwise call the function recursively on half the vector parameter: If integers.at(index) < target, search the vector from index + 1 to upper If integers.at(index) > target, search the vector from lower to index - 1 The vector…arrow_forwardWrite in c programming language . 8.11arrow_forward6.34 LAB: Contact list C++ A contact list is a place where you can store a specific contact with other associated information such as a phone number, email address, birthday, etc. Write a program that first takes as input an integer N that represents the number of word pairs in the list to follow. Word pairs consist of a name and a phone number (both strings). That list is followed by a name, and your program should output the phone number associated with that name. Ex: If the input is: 3 Joe 123-5432 Linda 983-4123 Frank 867-5309 Frank the output is: 867-5309 Your program must define and call the following function. The return value of GetPhoneNumber is the phone number associated with the specific contact name.string GetPhoneNumber(vector<string> nameVec, vector<string> phoneNumberVec, string contactName) Hint: Use two vectors: One for the string names, and the other for the string phone numbers.arrow_forward
- 8.13 LAB: Library book sorting Two sorted lists have been created, one implemented using a linked list (LinkedListLibrary linkedListLibrary) and the other implemented using the built-in Vector class (VectorLibrary vectorLibrary). Each list contains 100 books (title, ISBN number, author), sorted in ascending order by ISBN number. Complete main() by inserting a new book into each list using the respective LinkedListLibrary and VectorLibrary InsertSorted() methods and outputting the number of operations the computer must perform to insert the new book. Each InsertSorted() returns the number of operations the computer performs. Ex: If the input is: The Catcher in the Rye 9787543321724 J.D. Salinger the output is: Number of linked list operations: 1 Number of vector operations: 1 Which list do you think will require the most operations? Why? Main.cpp: #include "LinkedListLibrary.h"#include "VectorLibrary.h"#include "BookNode.h"#include "Book.h"#include <fstream>#include…arrow_forward8.16 LAB: Mileage tracker for a runner C++ Given the MileageTrackerNode class, complete main() to insert nodes into a linked list (using the InsertAfter() function). The first user-input value is the number of nodes in the linked list. Use the PrintNodeData() function to print the entire linked list. DO NOT print the dummy head node. Ex. If the input is: 3 2.2 7/2/18 3.2 7/7/18 4.5 7/16/18 the output is: 2.2, 7/2/18 3.2, 7/7/18 4.5, 7/16/18 I need the //TODO completed Main.cpp #include "MileageTrackerNode.h" #include <string> #include <iostream> using namespace std; int main (int argc, char* argv[]) { // References for MileageTrackerNode objects MileageTrackerNode* headNode; MileageTrackerNode* currNode; MileageTrackerNode* lastNode; double miles; string date; int i; // Front of nodes list headNode = new MileageTrackerNode(); lastNode = headNode; // TODO: Read in the number of nodes cin >> i int p = i; currNode…arrow_forward15.11 LAB: Sorting user IDs C++ Given a main() that reads user IDs (until -1), complete the Quicksort() and Partition() functions to sort the IDs in ascending order using the Quicksort algorithm, and output the sorted IDs one per line. Ex. If the input is: kaylasimms julia myron1994 kaylajones -1 the output is: julia kaylajones kaylasimms myron1994 I can only change the //TODO and please answer in C++ #include <string>#include <vector>#include <iostream> using namespace std; // TODO: Write the partitioning algorithm - pick the middle element as the// pivot, compare the values using two index variables l and h (low and high),// initialized to the left and right sides of the current elements being sorted,// and determine if a swap is necessaryint Partition(vector<string> &userIDs, int i, int k) { } // TODO: Write the quicksort algorithm that recursively sorts the low and// high partitionsvoid Quicksort(vector<string> &userIDs, int i, int k) {…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
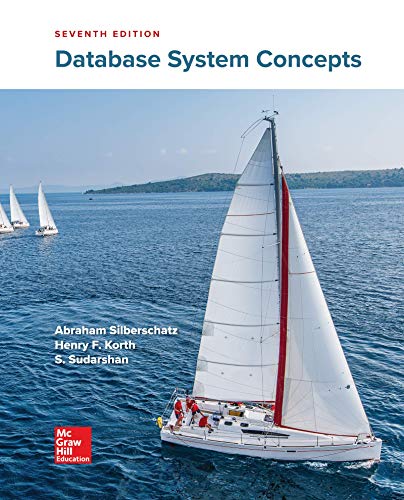
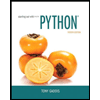
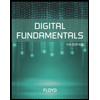
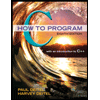
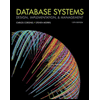
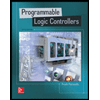