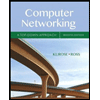
It's Halloween Night again! Michael, as old as he is, is still in love with
Halloween. This time around, he's gone trick-or-treating at Dan's house.
Dan's always been generous with candies. He's prepared a big bowl filled with n
candy bars. The i-th candy bar has k[i] ingredients, which are written on its
wrapper.
Michael is greedy and wants to take as many candy bars as possible.
However, he's allergic to m ingredients c[0], c[1], ..., c[m-1]. If a candy bar has
any of these m ingredients in it, Michael can't eat it so he won't take it.
Given a description of the candy bars and the ingredients that Michael is allergic
to, output the number of candy bars he will take.
## Input:
- The input consists of n+2 lines.
- The first line of input contains a single integer n.
- The next n lines contain the ingredients of the candy bars. Each line describes a
single candy bar as a list of space-separated ingredients that comprise the candy
bar.
- Finally, the last line contains a space-separated list of the ingredients Michael
is allergic to (i.e. the information from the list c of length m).
## Output:
Print a single integer, the number of candy bars Michael takes.
## Constraints:
- 1 <= n, m, k[i] <= 100
- Every ingredient is a non-empty string of lowercase english letters of length at
most 7.
- No candy bar has the same ingredient listed twice.
- No ingredient appears twice in the list of ingredients Michael is allergic to.
## Time Limit:
Your program has to finish running within 4 seconds on any valid input.
## Sample Input 1
```
3
sugar cocoa salt
salt celery poison bleach
almonds
celery carrots
```
## Sample Output 1
```
2
```
## Sample 1 Explanation
- There are three candy bars. They have 3, 4, and 1 ingredients each respectively.
- Michael is allergic to celery and carrots. This means that he won't take the
second candy bar as it contains celery.
- The other 2 candy bars are fine for him, so he'll take them.
## Sample Input 2
```
5
a b c
b d f
g d e f
c
a b c
b c
```
## Sample Output 2
```
1
```
"""

Step by stepSolved in 2 steps with 1 images

- A variation of the classic Dining Philosophers problem is the one where the philosophers get a "ticket", i.e. a number that identifies their priority. The philosophers are supposed to eat based on this priority, e.g. if the last one to dine had a ticket value of 7, then the next one to be allowed to dine, regardless if he has to wait for the release of one or two forks, is the one with a ticket value of 8. Each philosopher is supposed to go through the following sequence of actions: think () getTicket () getForks () eat () releaseForks () : should be implemented in a monitor : replace with a random delay in [0, 1]sec : should be implemented in a monitor : should be implemented in a monitor : replace with a random delay in [0, 1]sec You program should be able to work for a variable number of philosophers that are specified in the command-line. E.g. $ ./deli 10 should run the program for 10 philosophers. Your output should be similar to the following: Philosopher 1 got ticket 0…arrow_forwardSee below for how to lock: After being unlocked, only things with higher numbers can be locked again. Any time can be used to open a lock. There are no other locks used. Show how the process doesn't ensure that things will be sent in order.arrow_forwardin Javaarrow_forward
- A bandit armed with a revolver approached a quaker as he strolled down a rural road. A robbery was in progress, and he said, "Your money or your life!" My buddy answered, "I can't give you my money because then I'd be helping you do evil, but exchange is legal, so here, take my pocketbook in return for your gun." The robber accepted the purse, but the Quaker put the pistol to his head and said, "Now friend, give me back my pocketbook or the weapon may go off fire," as recounted by the thief.arrow_forwardIn the algorithmic world, the steps are very similar, but we can use simple loops to dowhat we are doing manually.This would translate to a set of steps as below:Step 1: Open a new spreadsheetStep 2: Create the header row in every worksheetStep 3: For each workbook from a storeFor each worksheet in the workbookRead rows 2 to last row that contains dataMove this row to the current rowAdvance to next rowNext worksheetNext workbookStep 4: Save workbook Write code for given algrithm.arrow_forwardAssign to maxSum the max of (numA, numB) PLUS the max of (numY, numZ). Use just one statement. Hint: Call findMax() twice in an expression. - I dont understand what I am doing wrong here, I keep getting an error code saying that non-static findMax(numA, numB) cannot be referenced from a static context. Anything helps! import java.util.Scanner; public class SumOfMax {public double findMax(double num1, double num2) {double maxVal; // Note: if-else statements need not be understood to// complete this activityif (num1 > num2) { // if num1 is greater than num2,maxVal = num1; // then num1 is the maxVal.}else { // Otherwise,maxVal = num2; // num2 is the maxVal.}return maxVal;} public static void main(String [] args) {double numA = 5.0;double numB = 10.0;double numY = 3.0;double numZ = 7.0;double maxSum = 0.0; // Use object maxFinder to call the methodSumOfMax maxFinder = new SumOfMax(); maxSum= (findMax(numA,numB)+findMax(numY,numZ)); System.out.print("maxSum is: " + maxSum);}}arrow_forward
- Help for number 3?arrow_forwardWrite a java program to print the Floyd's triangle after taking the number of rows from the user.arrow_forwardA band of thieves comes up with a series of rules for hiding their loot so that they are always able to find it. The rules are as follows: If the house is next to a lake, then the treasure does not go in the kitchen If the tree in the front yard is an elm, then the treasure is in the kitchen The tree in the front yard is an elm or the treasure is buried under the flagpole If the tree in the backyard is an oak then the treasure is in the garage. You observe that the house where the treasure is hidden is next to a lake. Where is the treasure? discrete matharrow_forward
- vWhere can I find out data's secrets?arrow_forwardWrite a Java program that reads a tic-tac-toe board and determines who has won the game or if it is a tie. You may assume that the game is over. Input X - O - X O O - X Output X wins Input O X O X O X X O X Output Tiearrow_forwardHi, this picture is the homework I have and it was on this site so I paid to see the answers, but it only answered question a. Can you show me how to solve the rest of the tasks?arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
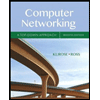
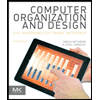
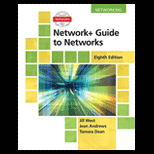
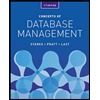
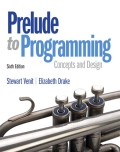
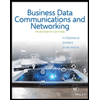