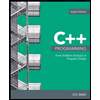
It is possible to sort an array of n values using pipeline of n filter processes.
The first process inputs all the values one at a time, keep the minimum, and passes the others
on to the next process. Each filter does the same thing; it receives a stream of values from the previous process, keep the smallest, and passes the others to the next process. Assume each process has local storage for only two values--- the next input value and the minimum it has seen so far.
(a) Developcode for filter processes. Declare the channels and use asynchronous message passing.
Hint:Define an array of channels value[n] (int), and a set of filter processes Filter[i = 0 to
n-1]. Each process Filter[i] (where 0 <= i <= n-2) receives a stream of integers through channel
value[i], keeps the smallest, and sends all other integers to channel value[i+1]. The last process
Filter[n-1] receives only one integer through channel value[n-1] and does not need to send any
integer further.

Step by stepSolved in 2 steps with 1 images

- How is an array stored in main memory? How is a linked list stored in main memory? What are their comparative advantages and disadvantages? Give examples of data that would be best stored as an array and as a linked list.arrow_forwardThe implementation of a queue in an array, as given in this chapter, uses the variable count to determine whether the queue is empty or full. You can also use the variable count to return the number of elements in the queue. On the other hand, class linkedQueueType does not use such a variable to keep track of the number of elements in the queue. Redefine the class linkedQueueType by adding the variable count to keep track of the number of elements in the queue. Modify the definitions of the functions addQueue and deleteQueue as necessary. Add the function queueCount to return the number of elements in the queue. Also, write a program to test various operations of the class you defined.arrow_forward(Program) Write a program that tests the effectiveness of the rand() library function. Start by initializing 10 counters, such as zerocount, onecount, twocount, and so forth, to 0. Then generate a large number of pseudorandom integers between 0 and 9. Each time 0 occurs, increment zerocount; when 1 occurs, increment onecount; and so on. Finally, display the number of 0s, 1s, 2s, and so on that occurred and the percentage of time they occurred.arrow_forward
- (Practice) Although the total number of bytes varies from computer to computer, memory sizes of millions and billions of bytes are common. In computer language, the letter M representsthe number 1,048,576, which is 2 raised to the 20th power, and G represents 1,073,741,824, which is 2 raised to the 30th power. Therefore, a memory size of 4 MB is really 4 times 1,048,576 (4,194,304 bytes), and a memory size of 2 GB is really 2 times 1,073,741,824 (2,147,483,648 bytes). Using this information, calculate the actual number of bytes in the following: a. A memory containing 512 MB b. A memory consisting of 512 MB words, where each word consists of 2 bytes c. A memory consisting of 512 MB words, where each word consists of 4 bytes d. A thumb drive that specifies 2 GB e. A disk that specifies 4 GB f. A disk that specifies 8 GBarrow_forward(Data processing) The answers to a true-false test are as follows: T T F F T. Given a twodimensional answer array, in which each row corresponds to the answers provided on one test, write a function that accepts the two-dimensional array and number of tests as parameters and returns a one-dimensional array containing the grades for each test. (Each question is worth 5 points, so the maximum possible grade is 25.) Test your function with the following data:arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrLINUX+ AND LPIC-1 GDE.TO LINUX CERTIF.Computer ScienceISBN:9781337569798Author:ECKERTPublisher:CENGAGE L
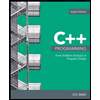
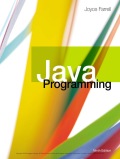
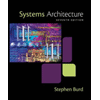
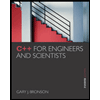
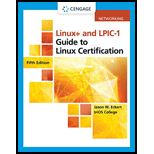