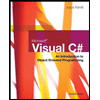
Concept explainers
The following is code for a disc golf program written in C++:
player.h:
#ifndef PLAYER_H
#define PLAYER_H
#include <string>
#include <iostream>
class Player {
private:
std::string courses[20]; // Array of course names
int scores[20]; // Array of scores
int gameCount; // Number of games played
public:
Player(); // Constructor
void CheckGame(const std::string& courseName, int gameScore);
void ReportPlayer(int playerId) const;
};
#endif // PLAYER_H
player.cpp:
#include "player.h"
#include <iomanip>
Player::Player() : gameCount(0) {}
void Player::CheckGame(const std::string& courseName, int gameScore) {
for (int i = 0; i < gameCount; ++i) {
if (courses[i] == courseName) {
// If course has been played, check for minimum score
if (gameScore < scores[i]) {
scores[i] = gameScore; // Update to new minimum score
}
return; // Exit after finding the course
}
}
// If course not found, add a new course and score
if (gameCount < 20) {
courses[gameCount] = courseName;
scores[gameCount] = gameScore;
++gameCount;
}
}
void Player::ReportPlayer(int playerId) const {
if (gameCount == 0) {
std::cout << "Player P" << playerId << " has no games\n";
} else {
std::cout << "Player P" << playerId << "\n";
for (int i = 0; i < gameCount; ++i) {
std::cout << "\t" << courses[i] << " " << scores[i] << "\n";
}
}
}
main.cpp:
#include <iostream>
#include <string>
#include <algorithm>
#include <limits>
#include "player.h"
int main() {
Player players[10]; // Array to hold players
std::string playerCode;
int inId, inScore;
std::string inCourse;
int maxPlayerIndex = -1; // Track the highest player ID
while (true) {
std::cout << "Enter player code or Q to quit: ";
std::cin >> playerCode;
// Check for quit input
if (playerCode == "q" || playerCode == "Q") break;
// Validate player code
if (playerCode.length() != 2 || playerCode[0] != 'P' || playerCode[1] < '0' || playerCode[1] > '9') {
std::cout << "Invalid player code. Please enter P0-P9.\n";
continue;
}
inId = playerCode[1] - '0'; // Extract player ID
if (inId < 0 || inId > 9) {
std::cerr << "Invalid player ID detected.\n";
continue;
}
maxPlayerIndex = std::max(maxPlayerIndex, inId); // Update the highest player ID
std::cin.ignore(std::numeric_limits<std::streamsize>::max(), '\n');
// Input course name
std::cout << "Enter course name: ";
std::getline(std::cin, inCourse);
// Input game score
std::cout << "Enter game score: ";
std::cin >> inScore;
std::cin.ignore(std::numeric_limits<std::streamsize>::max(), '\n');
std::cout << std::endl;
// Register the game for the player
players[inId].CheckGame(inCourse, inScore);
}
// Generate a report for all players
std::cout << "\nReport\n";
for (int i = 0; i <= maxPlayerIndex; ++i) {
players[i].ReportPlayer(i);
}
return 0;
}
Edit the program to allow both uppercase (as in P2) and lowercase (as in p2) inputs

Step by stepSolved in 2 steps

- // volunteer.h #include class Volunteer { public: Volunteer () { } std::string Name () void SetName (const private: std::string name_; }; const { return name_; } std::string& name) { name_ = name; }arrow_forwardsnack.h #include <string>#include <iostream>#include <vector>class Snack{public:Snack(std::string, float, bool);int set_price(float);void set_expired(bool);void set_name(std::string);std::string name() const{return name_;}float price() const{return price_;}bool expired() const{return expr_;}int how_many_for_ten();private:std::string name_;float price_;bool expr_;};void SortByPrice(std::vector<Snack>&,bool); main.cpp #include <iostream>using namespace std; #include "snack.h" int main(){ Snack snicker=Snack("Snickers",2.0,false); std::cout<<snicker.name()<<" "<<snicker.price()<<" "<<snicker.expired()<<std::endl; Snack twix=Snack("Twix",3.0,false); std::cout<<twix.name()<<" "<<twix.price()<<" "<<twix.expired()<<std::endl; Snack mars=Snack("Mars",1.5,false); std::cout<<mars.name()<<" "<<mars.price()<<" "<<mars.expired()<<std::endl;…arrow_forwardint class Inventory { std::vector<Item*> m_Items; static unsigned int m_ItemsMade; void CreateItem() { std::string name = "Item " + std::to_string(m_ItemsMade); m_Items.push_back(new Item(name.c_str(), 100 * m_ItemsMade)); ++m_ItemsMade; } public: Inventory() { CreateItem(); CreateItem(); CreateItem(); } void Print() const { size_t* nSize = new size_t(m_Items.size()); std::cout << "_____INVENTORY_____\n"; for (unsigned int i = 0; i < *nSize; ++i) { m_Items[i]->Print(); } } }; GetValidatedInt(const char* strMessage, int nMinimumRange = 0, int nMaximumRange = 0); This function should first display the provided message to the screen then use cin to get an int from the user. Error check and validate to ensure a legal integer was entered. If not, clear the cin buffer using clear() and ignore() and try again (Note: the buffer still needs to be cleared even if this step was successful). If a legal integer was entered, check its value to see if it is within the…arrow_forward
- #include <string>#include <iostream>#include <vector>class Snack{public:Snack(std::string, float, bool);int set_price(float);void set_expired(bool);void set_name(std::string);std::string name() const{return name_;}float price() const{return price_;}bool expired() const{return expr_;}int how_many_for_ten();private:std::string name_;float price_;bool expr_;};void SortByPrice(std::vector<Snack>&,bool); Task: Define the public function int Snack::set_price(float price) : If the value passed by price is smaller than 0 the function should return -2. If the value of price is greater or equal to 0, proceed as follows: If the value passed by price is smaller than the current value of price_, the function should assign price to price_ and return -1. If the value passed by price is larger than the current value of price_, the function should assign price to price_ and return 1. If price is equal to price_, the function should assign price to price_ and return…arrow_forward#include <string>#include <iostream>#include <vector>class Snack{public:Snack(std::string, float, bool);int set_price(float);void set_expired(bool);void set_name(std::string);std::string name() const{return name_;}float price() const{return price_;}bool expired() const{return expr_;}int how_many_for_ten();private:std::string name_;float price_;bool expr_;};void SortByPrice(std::vector<Snack>&,bool); Task: Implement a function working with Snack objects that are stored using a vector. It has the following signature: void SortByPrice(std::vector<Snack>& list_ref, bool asc). This function should sort the vector list_ref based on the price_ of the Snacks. The sorting should be done in the following order: Ascending if asc is true Descending if asc is false. In both cases if variables have the same value for price_, the sorting should be done based on the name_ in ascending order.arrow_forwardclass Artist{StringsArtist(const std::string& name="",int age=0):name_(name),age_(age){}std::string name()const {return name_;}void set_name(const std::string& name){name_=name;}int age()const {return age_;}void set_age(int age){age_=age;}virtual std::string perform(){return std::string("");}private:int age_;std::string name_;};class Singer : public Artist{public:Singer():Artist(){};Singer(const std::string& name,int age,int hits);int hits() const{return hits_;}void set_hits(int hit);std::string perform();int operator+(const Singer& rhs);int changeme(std::string name,int age,int hits);Singer combine(const Singer& rhs);private:int hits_=0;};int FindOldestandMostSuccess(std::vector<Singer> list); Implement the function Singer Singer::combine(const Singer& rhs):It should create a new Singer object, by calling the constructor with the following values: For name it should pass a combination of be the name of the calling object followed by a '+' and then…arrow_forward
- class Artist{StringsArtist(const std::string& name="",int age=0):name_(name),age_(age){}std::string name()const {return name_;}void set_name(const std::string& name){name_=name;}int age()const {return age_;}void set_age(int age){age_=age;}virtual std::string perform(){return std::string("");}private:int age_;std::string name_;};class Singer : public Artist{public:Singer():Artist(){};Singer(const std::string& name,int age,int hits);int hits() const{return hits_;}void set_hits(int hit);std::string perform();int operator+(const Singer& rhs);int changeme(std::string name,int age,int hits);Singer combine(const Singer& rhs);private:int hits_=0;};int FindOldestandMostSuccess(std::vector<Singer> list); Task: Implement the function int Singer::changeme(std::string name,int age,int hits) : This function should check if the values passed by name, age and hits are different than those stored. And if this is the case it should change the values. This should be done by…arrow_forwardclass Currency { protected: int whole; int fraction; virtual std::string get_name() = 0; public: Currency() { whole = 0; fraction = 0; } Currency(double value) { if (value < 0) throw "Invalid value"; whole = int(value); fraction = std::round(100 * (value - whole)); } Currency(const Currency& curr) { whole = curr.whole; fraction = curr.fraction; } /* This algorithm gets the whole part or fractional part of the currency Pre: whole, fraction - integer numbers Post: Return: whole or fraction */ int get_whole() { return whole; } int get_fraction() { return fraction; } /* This algorithm adds an object to the same currency Pre: object (same currency) Post: Return: */ void set_whole(int w) { if (w >= 0) whole = w; } void set_fraction(int f) { if (f >= 0 && f <…arrow_forwardThe following is code for a disc golf program written in C++: // player.h #ifndef PLAYER_H #define PLAYER_H #include <string> #include <iostream> class Player { private: std::string courses[20]; // Array of course names int scores[20]; // Array of scores int gameCount; // Number of games played public: Player(); // Constructor void CheckGame(int playerId, const std::string& courseName, int gameScore); void ReportPlayer(int playerId) const; }; #endif // PLAYER_H // player.cpp #include "player.h" #include <iomanip> Player::Player() : gameCount(0) {} void Player::CheckGame(int playerId, const std::string& courseName, int gameScore) { for (int i = 0; i < gameCount; ++i) { if (courses[i] == courseName) { // If course has been played, then check for minimum score if (gameScore < scores[i]) { scores[i] = gameScore; // Update to new minimum…arrow_forward
- C++arrow_forwardIn C++ struct myGrades { string class; char grade; }; Declare myGrades as an array that can hold 5 sets of data and then set a class string in each position as follows: “Math” “Computers” “Science” “English” “History” and give each class a letter gradearrow_forwardDate class to use: #ifndef DATE_H_ #define DATE_H_ #include <iostream> #include <iomanip> using namespace std; class Date { friend ostream &operator<<( ostream &, const Date & ); private: int day; int month; int year; static const int days[]; // array of days per month void helpIncrement(); // utility function for incrementing date public: Date(int=1, int=1, int=0); void setDate(int,int,int); bool leapYear( int ) const; // is date in a leap year? bool endOfMonth( int ) const; // is date at the end of month? Date &operator++(); // prefix increment operator Date operator++( int ); // postfix increment operator const Date &operator+=( int ); // add days, modify object bool operator<(const Date&) const; void showdate(); }; const int Date::days[] = { 0, 31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31 }; Date::Date(int d, int m, int y) { day = d; month = m; year = y; // initialize static member at file scope; one classwide copy } // set month,…arrow_forward
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
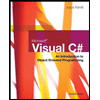
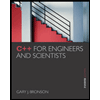
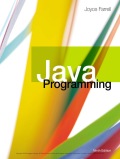