(Intro to Java) Tracking Customers This program will read in a series of names, along with an associated gender, from an input file of unknown length. The program uses a while loop to read in each name from the file by using the hasNextLine() method from the Scanner class. Please use a while loop for practice, although you could also write this assignment using a for loop to read from the file. As you read in each name, you will store it in an array, along with a title (Mr. or Ms. or Mx.) dependent on the stated gender. The first line of each file will contain the number of names contained in the file (hint: this is the same structure as the input file in your assignment 18.2). Additionally, this program should have two methods, as follows: The first method: The method is named extractInitial It takes a String parameter It extracts the first letter (initial) from the String parameter It returns a char for the initial. Note: You must write a complete Javadoc for this method to receive full credit. The second method: The method is named printArray It takes an array of Strings as a parameter It has a second String parameter which is a file name It opens up a new PrintWriter and connects it to the file passed in as a parameter It prints out the array to the file using a for loop It closes the PrintWriter It returns nothing Hint: You will need to update the method signature to throw an IOException. Note: You must write a complete Javadoc for this method to receive full credit. Part of the trick of this assignment will be to determine the appropriate time to call each method. Copy and paste the starter code below into a new file called TrackCustomers.java /** * @author * */ import java.util.Scanner; //add an import statement here public class TrackCustomers { /** * Write the javadoc comment for extractInitial here */ public static char extractInitial(String customer) { return '\0'; //replace this line when you write the method } /** * Write the javadoc comment for printArray here */ public static void printArray(String[] customers) //update signature of method { return; } public static void main(String[] args) { String first_name, last_name, gender; final int ARRAY_SIZE; final String INPUT_FILE; final String OUTPUT_FILE; System.out.println("Welcome to Customer Tracker!\n"); //Use a while loop and hasNextLine() - required! } } Sample output: Welcome to Customer Tracker! Enter the name of the input file: customers1.txt Enter the name of the output file: updated.txt Thank you! The customer information has been written to updated.txt! Please open the file to see the information. Note that the user should be able to enter any files names they want. The above file names are just an example. Example input file: 15 Barbara Stanton F Timoteo Martinez M Ally Gu O Xing Xiao M Dung Kim F Tanya White F Alan Ngo M Abir Fadel M Nataly Luna F Charles Atkinson M Stacey Cahill O Stephanie Teatro F Ping Li M June Park F Binh Nguyen M Corresponding output file: Ms. B. Stanton Mr. T. Martinez Mx. A. Gu Mr. X. Xiao Ms. D. Kim Ms. T. White Mr. A. Ngo Mr. A. Fadel Ms. N. Luna Mr. C. Atkinson Mx. S. Cahill Ms. S. Teatro Mr. P. Li Ms. J. Park Mr. B. Nguyen Example input file: 19 Jiming Wu M James Brown M Liana Perez F Xing Li M Stacey Cahill O Mohammed Abbas M Kumari Chakrabarti F Shakil Smith M Jung Ahrin O Pedro Martinez M Madison Wong O Tamara White F Alvin Ngo M Abir Fadel M Brad Feinman M Xiaohang Yue M Triana Brown F Anh Bao F Katiya Ivankova F Corresponding output file: Mr. J. Wu Mr. J. Brown Ms. L. Perez Mr. X. Li Mx. S. Cahill Mr. M. Abbas Ms. K. Chakrabarti M. S. Smith Mx. J. Ahrin Mr. P. Martinez Mx. M. Wong Ms. T. White Mr. A. Ngo Mr. A. Fadel Mr. B. Feinman Mr. X. Yue Ms. T. Brown Ms. A. Bao Ms. K. Ivankova
(Intro to Java) Tracking Customers This program will read in a series of names, along with an associated gender, from an input file of unknown length. The program uses a while loop to read in each name from the file by using the hasNextLine() method from the Scanner class. Please use a while loop for practice, although you could also write this assignment using a for loop to read from the file. As you read in each name, you will store it in an array, along with a title (Mr. or Ms. or Mx.) dependent on the stated gender. The first line of each file will contain the number of names contained in the file (hint: this is the same structure as the input file in your assignment 18.2). Additionally, this program should have two methods, as follows: The first method: The method is named extractInitial It takes a String parameter It extracts the first letter (initial) from the String parameter It returns a char for the initial. Note: You must write a complete Javadoc for this method to receive full credit. The second method: The method is named printArray It takes an array of Strings as a parameter It has a second String parameter which is a file name It opens up a new PrintWriter and connects it to the file passed in as a parameter It prints out the array to the file using a for loop It closes the PrintWriter It returns nothing Hint: You will need to update the method signature to throw an IOException. Note: You must write a complete Javadoc for this method to receive full credit. Part of the trick of this assignment will be to determine the appropriate time to call each method. Copy and paste the starter code below into a new file called TrackCustomers.java /** * @author * */ import java.util.Scanner; //add an import statement here public class TrackCustomers { /** * Write the javadoc comment for extractInitial here */ public static char extractInitial(String customer) { return '\0'; //replace this line when you write the method } /** * Write the javadoc comment for printArray here */ public static void printArray(String[] customers) //update signature of method { return; } public static void main(String[] args) { String first_name, last_name, gender; final int ARRAY_SIZE; final String INPUT_FILE; final String OUTPUT_FILE; System.out.println("Welcome to Customer Tracker!\n"); //Use a while loop and hasNextLine() - required! } } Sample output: Welcome to Customer Tracker! Enter the name of the input file: customers1.txt Enter the name of the output file: updated.txt Thank you! The customer information has been written to updated.txt! Please open the file to see the information. Note that the user should be able to enter any files names they want. The above file names are just an example. Example input file: 15 Barbara Stanton F Timoteo Martinez M Ally Gu O Xing Xiao M Dung Kim F Tanya White F Alan Ngo M Abir Fadel M Nataly Luna F Charles Atkinson M Stacey Cahill O Stephanie Teatro F Ping Li M June Park F Binh Nguyen M Corresponding output file: Ms. B. Stanton Mr. T. Martinez Mx. A. Gu Mr. X. Xiao Ms. D. Kim Ms. T. White Mr. A. Ngo Mr. A. Fadel Ms. N. Luna Mr. C. Atkinson Mx. S. Cahill Ms. S. Teatro Mr. P. Li Ms. J. Park Mr. B. Nguyen Example input file: 19 Jiming Wu M James Brown M Liana Perez F Xing Li M Stacey Cahill O Mohammed Abbas M Kumari Chakrabarti F Shakil Smith M Jung Ahrin O Pedro Martinez M Madison Wong O Tamara White F Alvin Ngo M Abir Fadel M Brad Feinman M Xiaohang Yue M Triana Brown F Anh Bao F Katiya Ivankova F Corresponding output file: Mr. J. Wu Mr. J. Brown Ms. L. Perez Mr. X. Li Mx. S. Cahill Mr. M. Abbas Ms. K. Chakrabarti M. S. Smith Mx. J. Ahrin Mr. P. Martinez Mx. M. Wong Ms. T. White Mr. A. Ngo Mr. A. Fadel Mr. B. Feinman Mr. X. Yue Ms. T. Brown Ms. A. Bao Ms. K. Ivankova
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
(Intro to Java)
Tracking Customers
- This program will read in a series of names, along with an associated gender, from an input file of unknown length.
- The program uses a while loop to read in each name from the file by using the hasNextLine() method from the Scanner class.
- Please use a while loop for practice, although you could also write this assignment using a for loop to read from the file.
- As you read in each name, you will store it in an array, along with a title (Mr. or Ms. or Mx.) dependent on the stated gender.
- The first line of each file will contain the number of names contained in the file (hint: this is the same structure as the input file in your assignment 18.2).
- Additionally, this program should have two methods, as follows:
- The first method:
- The method is named extractInitial
- It takes a String parameter
- It extracts the first letter (initial) from the String parameter
- It returns a char for the initial.
- Note: You must write a complete Javadoc for this method to receive full credit.
- The second method:
- The method is named printArray
- It takes an array of Strings as a parameter
- It has a second String parameter which is a file name
- It opens up a new PrintWriter and connects it to the file passed in as a parameter
- It prints out the array to the file using a for loop
- It closes the PrintWriter
- It returns nothing
- Hint: You will need to update the method signature to throw an IOException.
- Note: You must write a complete Javadoc for this method to receive full credit.
- Part of the trick of this assignment will be to determine the appropriate time to call each method.
- Copy and paste the starter code below into a new file called TrackCustomers.java
/**
* @author
*
*/
import java.util.Scanner;
* @author
*
*/
import java.util.Scanner;
//add an import statement here
public class TrackCustomers
{
/**
* Write the javadoc comment for extractInitial here
*/
public static char extractInitial(String customer)
{
return '\0'; //replace this line when you write the method
}
/**
* Write the javadoc comment for printArray here
*/
public static void printArray(String[] customers) //update signature of method
{
return;
}
public static void main(String[] args)
{
/**
* Write the javadoc comment for extractInitial here
*/
public static char extractInitial(String customer)
{
return '\0'; //replace this line when you write the method
}
/**
* Write the javadoc comment for printArray here
*/
public static void printArray(String[] customers) //update signature of method
{
return;
}
public static void main(String[] args)
{
String first_name, last_name, gender;
final int ARRAY_SIZE;
final String INPUT_FILE;
final String OUTPUT_FILE;
System.out.println("Welcome to Customer Tracker!\n");
//Use a while loop and hasNextLine() - required!
}
}
- Sample output:
Welcome to Customer Tracker!
Enter the name of the input file: customers1.txt
Enter the name of the output file: updated.txt
Thank you! The customer information has been written to updated.txt!
Please open the file to see the information.
- Note that the user should be able to enter any files names they want. The above file names are just an example.
- Example input file:
15
Barbara Stanton
F
Timoteo Martinez
F
Timoteo Martinez
M
Ally Gu
O
Xing Xiao
M
Dung Kim
F
Tanya White
F
Alan Ngo
M
Abir Fadel
M
Nataly Luna
F
Charles Atkinson
M
Stacey Cahill
O
Stephanie Teatro
F
Ping Li
M
June Park
F
Binh Nguyen
M
- Corresponding output file:
Ms. B. Stanton
Mr. T. Martinez
Mx. A. Gu
Mr. X. Xiao
Ms. D. Kim
Ms. T. White
Mr. A. Ngo
Mr. A. Fadel
Mr. T. Martinez
Mx. A. Gu
Mr. X. Xiao
Ms. D. Kim
Ms. T. White
Mr. A. Ngo
Mr. A. Fadel
Ms. N. Luna
Mr. C. Atkinson
Mx. S. Cahill
Ms. S. Teatro
Mr. P. Li
Ms. J. Park
Mr. B. Nguyen
- Example input file:
19
Jiming Wu
M
James Brown
M
Liana Perez
F
Xing Li
M
Stacey Cahill
O
Mohammed Abbas
M
Kumari Chakrabarti
F
Shakil Smith
M
Jung Ahrin
O
Pedro Martinez
M
Madison Wong
O
Tamara White
F
Alvin Ngo
M
Abir Fadel
M
Brad Feinman
M
Xiaohang Yue
M
Triana Brown
F
Anh Bao
F
Katiya Ivankova
F
- Corresponding output file:
Mr. J. Wu
Mr. J. Brown
Ms. L. Perez
Mr. X. Li
Mx. S. Cahill
Mr. M. Abbas
Ms. K. Chakrabarti
M. S. Smith
Mx. J. Ahrin
Mr. P. Martinez
Mx. M. Wong
Ms. T. White
Mr. A. Ngo
Mr. A. Fadel
Mr. B. Feinman
Mr. X. Yue
Ms. T. Brown
Ms. A. Bao
Ms. K. Ivankova
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 4 images

Recommended textbooks for you
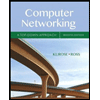
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
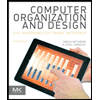
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
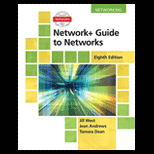
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
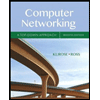
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
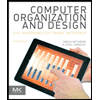
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
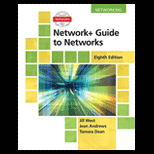
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
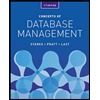
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
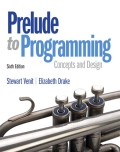
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
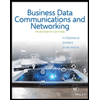
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY