Instructions: Kindly implement the PYTHON CODING given below and refer for the expected output of the program in the photo provided below! I will like your solution if you answered it correctly with complete solutions, thank you~ class Fish: def __init__(self, size, name): self.size = size self.name = name pass def eat_fish(self, other_fish): # TODO: Add method that simulates this fish # trying to feed other_fish if self.size > other_fish.size: m = self.size + other_fish.size return self.size elif self.size < other_fish.size: n = self.size + other_fish.size return n # This method returns the "winning" `Fish` pass def feed(self, pellet_size): # TODO: Add method that simulates this fish # feeding on a pellet of size pellet_size # # This method returns nothing (i.e. None) pass def main(): t = int(input()) for i in range(t): n_fishes, n_evt = [int(x) for x in input().split()] for _ in range(n_fishes): name_f, size_f = input().split(maxsplit=1) # TODO: process each fish with initial sizes pass for _ in range(n_evt): evt, evt_args = input().split(maxsplit=1) evt = evt.strip() print(evt) if evt == "eat": evt_args = name_f, name_f print(evt_args) elif evt == "feed": evt_args = name_f, size_f print(evt_args) # TODO: Process feeding events # TODO: Print remaining fishes and fishes sorted in # decreasing size print(f"Case #{t}: Remaining fish(es):") print(evt_args.eat_fish()) # HINT: Use `sorted()` with a `key` argument. if __name__ == '__main__': main()
Instructions: Kindly implement the PYTHON CODING given below and refer for the expected output of the program in the photo provided below! I will like your solution if you answered it correctly with complete solutions, thank you~
class Fish:
def __init__(self, size, name):
self.size = size
self.name = name
pass
def eat_fish(self, other_fish):
# TODO: Add method that simulates this fish
# trying to feed other_fish
if self.size > other_fish.size:
m = self.size + other_fish.size
return self.size
elif self.size < other_fish.size:
n = self.size + other_fish.size
return n
# This method returns the "winning" `Fish`
pass
def feed(self, pellet_size):
# TODO: Add method that simulates this fish
# feeding on a pellet of size pellet_size
#
# This method returns nothing (i.e. None)
pass
def main():
t = int(input())
for i in range(t):
n_fishes, n_evt = [int(x) for x in input().split()]
for _ in range(n_fishes):
name_f, size_f = input().split(maxsplit=1)
# TODO: process each fish with initial sizes
pass
for _ in range(n_evt):
evt, evt_args = input().split(maxsplit=1)
evt = evt.strip()
print(evt)
if evt == "eat":
evt_args = name_f, name_f
print(evt_args)
elif evt == "feed":
evt_args = name_f, size_f
print(evt_args)
# TODO: Process feeding events
# TODO: Print remaining fishes and fishes sorted in
# decreasing size
print(f"Case #{t}: Remaining fish(es):")
print(evt_args.eat_fish())
# HINT: Use `sorted()` with a `key` argument.
if __name__ == '__main__':
main()

![When you were in grade school, your parents purchased for you a fish tank that contained two fishes. You
have been feeding them everyday and have noticed that over the days, one of the fishes have become bigger
than the other. Then, on one fateful day, only one fish remained in the tank. It is the bigger one that has
grown bigger during the day.
Until now, you have been curious about fish survival in such captivity. So, you have decided to simulate fish
growth by writing a program that "commands" fishes to either feed from a given pellet or eat another fish. All
fishes in the program start with an initial size. If a fish is fed with a pellet of size P, its size will grow to an
additional Punits. On the other hand, a fish A can try to eat another fish B. If A is bigger, then B will be
eaten by A and A will grow to an additional |B| (size of fish B) units. Otherwise, A gets eaten and B will grow
in a similar manner. The devoured fish definitely disappears from the tank.
For this simulation, you are interested in which fishes remain after all of the fishes have fed and eaten.
Input Format
The input to the program starts with a number T denoting the number of simulated fish tanks. T blocks of
lines then follow, with each block denoting a fish tank.
The first line in a block consists of two numbers N_f N_c with N_f denoting the number of fishes and N_c
the number of feeding events. The next N_f lines denote information about a fish of the format F_{name}
F_{size}. F_{name} is the name of the fish and F_{size} its initial size. Then the next N_c has this
format evt_name evt_args where evt_name is a feeding event and evt_args a space-separated list of
relevant event information. evt_name will only be from one of the following examples:
feed fish_name pellet_size-feed fish with name fish_name with a pellet of size pellet_size
eat fish_a fish_b - fish with name fish_a tries to eat fish with name fish_b
Constraints
Input Constraints
T≤ 10
0 < N₁ ≤ 50
0 < №e ≤ 100
fish_name consists only of alphanumeric characters and underscores.
Every fish_name is unique. They are case-sensitive, so the fishes named guppy and guppy are two
different fishes.
0 < |fish_name] ≤ 100
0 < fish_size ≤ 100
evt_name € { feed, eat}
You can assume that all of the inputs are well-formed and are always provided within these constraints. You
are not required to handle any errors.
Functional Constraints
You are required to implement a class named Fish with the following methods:
_init__(self, size: int, name: str) -add a fish with size initial size and name name in the
●
tank
eat_fish(self, other_fish: Fish) -> Fish - this fish tries to eat other_fish. This method
returns the "winning" fish.
feed(self, pellet_size: int) - this fish eats a pellet of size pellet_size](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd7a11170-bfa3-4bdf-ac5c-1156bdc056c3%2Ffcf5619e-c3d9-43de-a62c-85a11984330a%2F3pfpcso_processed.png&w=3840&q=75)

Step by step
Solved in 3 steps

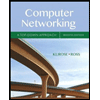
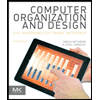
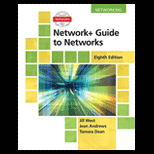
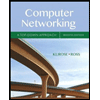
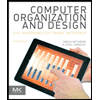
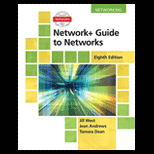
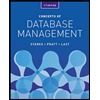
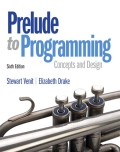
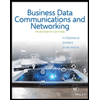