include "queueLL.h" #include "priorityQueueLL.h" using namespace std; int main() { //////////Test code for priority queue///// priorityQueueLL pQueue; const int SIZE = 20; //insert a bunch of random numbers for (int i = 0; i < SIZE; i++) { pQueue.insert(rand()); } //pull them back out..
Can someone help me with C++? I have to implement a priorityQueue Linked List in my header file. It has to work with the tests that are in the main cpp file. Can't be adjusted.
//////////////////////////////////////////
driver.cpp
//////////////////////////////////////////
#include <iostream>
#include <string>
#include "stackLL.h"
#include "queueLL.h"
#include "priorityQueueLL.h"
using namespace std;
int main()
{
//////////Test code for priority queue/////
priorityQueueLL<int> pQueue;
const int SIZE = 20;
//insert a bunch of random numbers
for (int i = 0; i < SIZE; i++)
{
pQueue.insert(rand());
}
//pull them back out..
//They must come out in order from smallest to largest
while (!pQueue.empty())
{
cout << pQueue.extractMin() << endl;
}
priorityQueueLL<string> pqs;
pqs.insert("whale");
pqs.insert("snake");
pqs.insert("buffalo");
pqs.insert("elmo");
pqs.insert("fire");
pqs.insert("waffle");
//buffalo elmo fire snake waffle whale
while (!pqs.empty())
{
cout << pqs.extractMin() << endl;
}
return 0;
}
//////////////////////////////////////////
priorityQueueLL.h (In the image)
//////////////////////////////////////////

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

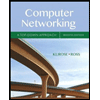
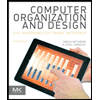
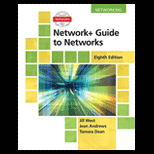
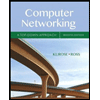
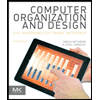
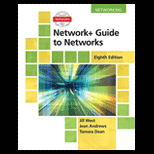
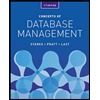
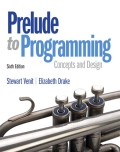
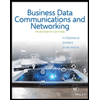