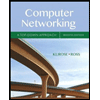
- Include private member data items for the x, y coordinates of the point (type double) pair
- Completed code for the overloaded ostream operator is provided. Implement code for an overloaded istream operator as a friend of the class
- Write a constructor that takes two arguments, each of type const double & and sets default values = 0. for each argument. Use member initialization to set the x-coordinate data member = arg 1 and the y-coordinate data member = arg 2
- Implement one set function and one get function for each of the private member data items: x and y
- Overload the arithmetic operators +, -. To implement the operators, apply the indicated arithmetic operation using the x and y coordinate values of the appropriate Point objects, respectively
- Add member function, isCoincident with the following prototype
bool isCoincident(const Point &P);
class Point {
friend ostream & operator<<( ostream &output, const Point &P ){
output << "x: " << P.x << " y: " << P.y;
return output;
}
// overloaded istream operator...
/**********************************************************
* Write your code here
public:
// constructor...
/**********************************************************
* Write your code here
**********************************************************/
// setX...
/**********************************************************
* Write your code here
**********************************************************/
// setY...
/**********************************************************
* Write your code here
**********************************************************/
// getX...
/**********************************************************
* Write your code here
**********************************************************/
// getY...
/**********************************************************
* Write your code here
**********************************************************/
// operator +()...
Point operator +(const Point &P) {
return Point(/* fill me in */);
}
// operator –()...
Point operator -(const Point &P) {
/**********************************************************
* Write your code here
**********************************************************/
}
bool isCoincident(const Point &P) {
/**********************************************************
* Write your code here
**********************************************************/
}
private:
/**********************************************************
* Write your code here for private member data items
**********************************************************/
};

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Using C++ programming language make a class Cone having radius and height as class members. Use parameterized constructor to initialize the radius and height of Cone. Use one member function display to print the area of total surface of cone.arrow_forwardRectangle Class Observe the Rectangle class in rectangle.h, and you'll see it has two member variables: length_ and width_. These member variables can be modified with the "Setter functions" SetLength and SetWidth (aka mutator functions) and can be retrieved with the "getter functions" GetLength and GetWidth (aka accessor functions). You will be implementing two member functions of the Rectangle class: Area, and Perimeter. Notice that if a function belongs to a class, when we define the function outside of the class, we indicate that it is a member function of a class using the :: operator. For example, because Area() belongs to the Rectangle class, when we define the function in rectangle.cc, it is written as: int Rectangle::Area() { ... } After implementing these two member functions, you will implement a function, LargestRectangleByArea that compares two input Rectangle objects and returns which of the two is larger, based on its area. Implement the member function Area that…arrow_forwardIn the Member class, complete the function definition for SetNumPoints() that takes in the integer parameter newNumPoints. Ex: If the input is 4.5 62, then the output is: Height: 4.5 Number of points: 62 3 4 class Member { 5 6 7 8 9 10 11 12 17 } 1's public: void SetHeight (double newHeight); void SetNumPoints (int newNumPoints); 13 20 21 } double GetHeight() const; int GetNumPoints() const; private: 13 }; 14 15 void Member::SetHeight (double newHeight) { height= newHeight; 16 double height; int numPoints; ce es erabyCamScanner ur numPoints = newNumPoints;arrow_forward
- Implement a nested class composition relationship between any two class types from the following list: Advisor Вook Classroom Department Friend Grade School Student Teacher Tutor Write all necessary code for both classes to demonstrate a nested composition relationship including the following: a. one encapsulated data member for each class b. inline default constructor using constructor delegation for each class c. inline one-parameter constructor for each class d. inline accessors for all data members e. inline mutators for all data membersarrow_forwardT/F Example Code Ch 09-2Assume that Poodle is a derived class of Dog and that Dog d = new Dog(...) and Poodlep = new Poodle(...) where the ... are the necessary parameters for the two classes.7. Refer to Example Code Ch 09-2: The assignment statement d = p; is legal even though d is not aPoodle.arrow_forward(2) Your member functions will be: Make sure that the mutators for height and width only take positive numbers! Test your class with the driver program given below. //--- Test driver for class Rectangle #include using namespace std; setHeight (int h): mutator for height variable setWidth (int w): mutator for width variable setDimensions (int h, int w): sets both height and width getArea (): returns the area of the rectangle getPerimeter () returns the perimeter of the rectangle print (): prints a rectangle with your dimensions composed of asterisks (3) Create a default constructor that sets the default values of Rectangle class to 1. int main() { Rectangle rec rec2; recl.setHeight (10); recl.setWidth (20); Page rec2.setDimensions (5, 10); cout of 2 ZOOM +arrow_forward
- write the definition of a class statistics containing: three data members x, y, and z of type integer. a member function called setValues that accepts three parameters and assigns them to x, y, and z the function returns no value. a member function called getSum that accepts no parameters and returns the sum of the member variables x, y, and z. a member function called getAvg that accepts no parameters and returns the average of the member variables x, y, and z (the average should be returnsed as a double value. to do this you can use a convert function or just divide by 3.0) a member function called getMin that accepts no parameters and returns the smallest member variable. a member function called getMax that accepts no parameters and returns the largest member variable.arrow_forwardUsing C++ Define the class called Student. The Student class has the following: Part 1) Private data members: name(string), age(int), units(int). The units represent the number of quarter units student is enrolled in. Define a default constructor as well as a constructor with parameters for the class Student. The class must have get and set functions for all private data members. The set function for the data member units must throw “out_of_range” exception if the number of units is not between 1 and 15. Include a function called tuition (double feePerUnit) that computes and returns the cost of registering for the number of units (in the private data member). The function receives the cost per unit as a parameter. Overload the operator (<<) to display student name and age. Test the class Student by writing a main program in which a Student object is created and displayed. Call the function tution(), you may pass any value as feePerUnit parameter to this function and display the…arrow_forwardDouble Bubble For this exercise you need to create a Bubble class and construct two instances of the Bubble object. You will then take the two Bubble objects and combine them to create a new, larger combined Bubble object. This will be done using functions that take in these Bubble objects as parameters. The Bubble class contains one data member, radius_, and the corresponding accessor and mutator methods for radius_, GetRadius and SetRadius. Create a member function called CalculateVolume that computes for the volume of a bubble (sphere). Use the value 3.1415 for PI. Your main function has some skeleton code that asks the user for the radius of two bubbles. You will use this to create the two Bubble objects. You will create a CombineBubbles function that receives two references (two Bubble objects) and returns a Bubble object. Combining bubbles simply means creating a new Bubble object whose radius is the sum of the two Bubble objects' radii. Take note that the CombineBubbles function…arrow_forward
- shows the complete anagram.cpp program. Use a class to represent the word to be anagrammed. Member functions of the class allow the word to be displayed, anagrammed, and rotated. The main() routine gets a word from the user, creates a word object with this word as an argument to the constructor, and calls the anagram() member function to anagram the word.arrow_forwardThe base class Pet has protected data members petName, and petAge. The derived class Dog extends the Pet class and includes a private data member for dogBreed. Complete main() to: create a generic pet and print information using PrintInfo(). create a Dog pet, use PrintInfo() to print information, and add a statement to print the dog's breed using the GetBreed() function. Ex. If the input is: Dobby2Kreacher3German Schnauzer the output is: Pet Information: Name: Dobby Age: 2Pet Information: Name: Kreacher Age: 3 Breed: German Schnauzer #include <iostream>#include<string>#include "Dog.h" using namespace std; int main() { string petName, dogName, dogBreed; int petAge, dogAge; Pet myPet; Dog myDog; getline(cin, petName); cin >> petAge; cin.ignore(); getline(cin, dogName); cin >> dogAge; cin.ignore(); getline(cin, dogBreed); // TODO: Create generic pet (using petName, petAge) and then call PrintInfo //…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
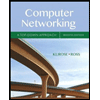
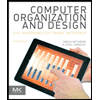
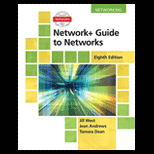
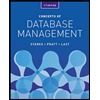
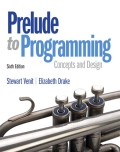
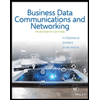